Have you ever encountered this situation: you wrote a bunch of code, but it always reports errors when running, and you can't find out where the problem is after searching for a long time? Debugging code is really a headache! But don't worry, today I'll teach you some practical Python debugging techniques step by step, so you'll fall in love with debugging from now on!
Basic Debugging Tools
Let's start with the most basic debugging tools and methods.
pdb and ipdb modules
Python has a built-in very useful pdb
module that allows you to set breakpoints in your code for debugging. You just need to insert the line import pdb; pdb.set_trace()
at key locations in your code, and the program will pause execution there, waiting for you to enter commands for debugging.
You ask me, why not just print variable values? That's because the pdb
module can do far more than that! For example, you can use the n
(next) command to execute the code step by step, use p variable_name
to print the value and type of variables, use c
(continue) to resume execution, and so on. It's really a treasure trove!
However, if you prefer debugging in the IPython environment, then use the enhanced ipdb
module. It supports auto-completion and syntax highlighting, which is quite handy to use!
print and logging
Of course, our old methods of printing and logging are also very useful when debugging. You can print variable values at key locations in the code, or use the logging
module to record detailed log information.
However, I personally prefer to define a debug_print
function, so that all debug outputs can be disabled with one click in the release version. Isn't that convenient?
DEBUG = True
def debug_print(*args):
if DEBUG:
print(*args)
x = 10
debug_print(f"The value of x is: {x}")
IDE debugging tools
Let's talk about IDE debugging tools. For example, the famous PyCharm has a built-in super powerful debugger that supports various practical functions such as visual code execution tracking and variable monitoring. Even the compact Visual Studio Code has pretty good debugging support.
Using IDE debugging tools, you can find and fix bugs with half the effort, which is really convenient! However, one thing to note is that when debugging large projects, the performance of the IDE may be affected to some extent, so we still need to choose the best debugging method according to the specific situation.
Advanced Debugging Techniques
Next, let's talk about some more advanced debugging techniques. Get ready, these are the big moves!
Minimal Reproducible Example
When seeking help from others, it's best to provide a "Minimal Reproducible Example" (MRE). An MRE is a minimized code snippet that can reproduce the problem you encountered.
The trick to building an MRE is to keep the code as concise and readable as possible, remove all irrelevant parts, while including enough context information. Don't forget to accurately describe the expected and actual behavior, and provide detailed error messages and relevant code lines. With an MRE, others can understand and locate your problem more quickly.
Tracing with the trace module
Want to fully understand the execution process of your code? Then come use the trace
module! It can record the execution of every line in your code, including all function calls, exception throws, line number changes, etc., and finally generate a detailed log file.
With this log, you can investigate the code step by step like an old detective, discovering the root cause of the problem from it. Isn't that interesting?
repr() magic method
Want to better view the internal state of objects during debugging? Then define a useful __repr__
magic method for it!
The return value of this method will be displayed when the object is printed. For example, for a student class, you can make it print important information such as the student's name, age, and score, instead of a boring memory address. This is very helpful for debugging objects!
Common Problems and Solutions
Even with these tools, we still encounter some common problems during debugging. Let's see how to solve them efficiently.
Script won't run
If your Python script won't run, first check if the Python version and dependent libraries are correctly installed. If everything is fine, try using python -m pdb script.py
to start the script and enter debug mode to see if there's a problem with the code itself.
Syntax errors
Syntax errors are usually prompted by the IDE when you're writing code. But if you insist on checking manually, you can use python -m py_compile script.py
to compile your code, which will list all syntax errors for you to fix one by one.
Debugging Best Practices
Finally, let's talk about some best practices in the debugging process to make your debugging journey more efficient and smooth.
Divide and conquer strategy
When faced with a tricky bug, don't blindly make changes here and there, that will only make things worse. We need to adopt a "divide and conquer" strategy, gradually narrowing down the scope of the problem until we find the root cause.
For example, try commenting out part of the code to see if the problem still exists; or add more log outputs to understand where the code failed; or write unit tests to test whether the behavior of each module meets expectations. By progressively investigating and fixing bugs, you'll achieve twice the result with half the effort.
The power of version control
You must have used version control systems like Git, right? It's a powerful assistant when debugging! Whenever you modify the code, commit it to the Git repository, so you can roll back to previous versions at any time, compare differences between different versions, and quickly locate and fix problems.
The importance of unit testing
Writing unit tests not only helps you better understand the code, but also helps detect potential bugs when introducing new features. Unit tests are like your private detective, always keeping an eye on every line of code to ensure they're all running normally.
You see, debugging is not scary at all. As long as you master the right methods and tools, you can handle the debugging process of Python code very well. Now do you have a new understanding of debugging? Hurry up and put it into practice, and let debugging become a great help in your programming career!
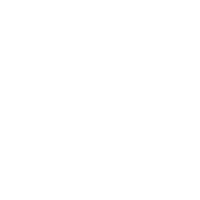
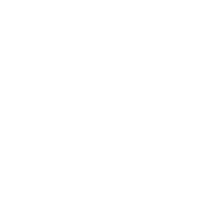
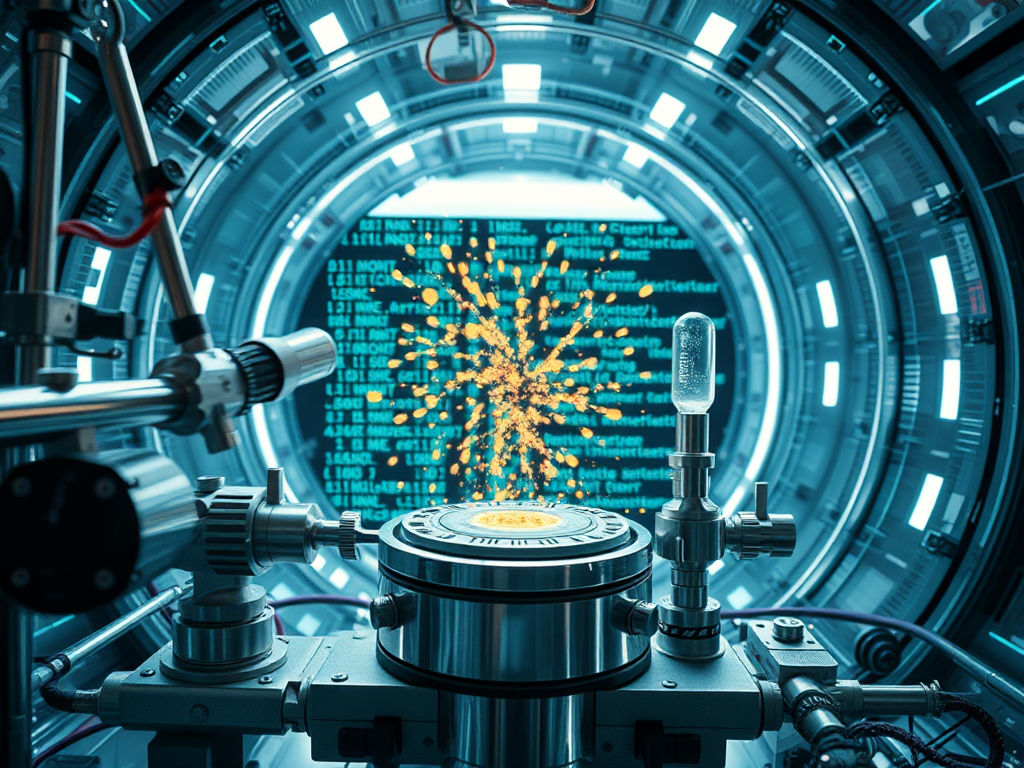
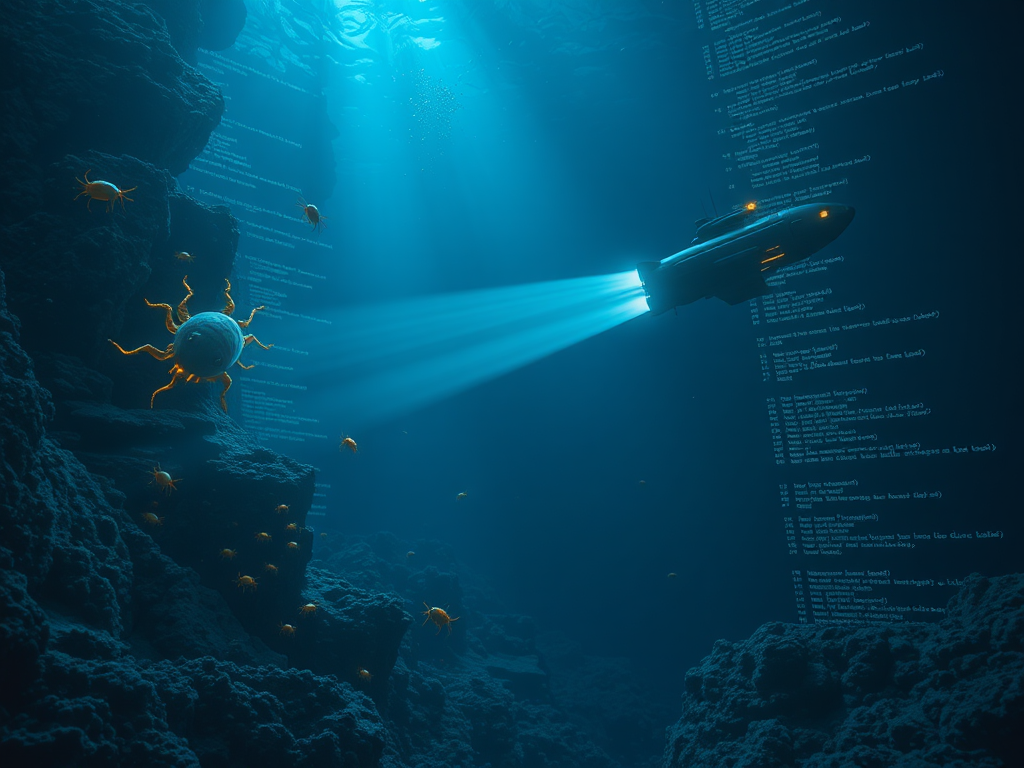
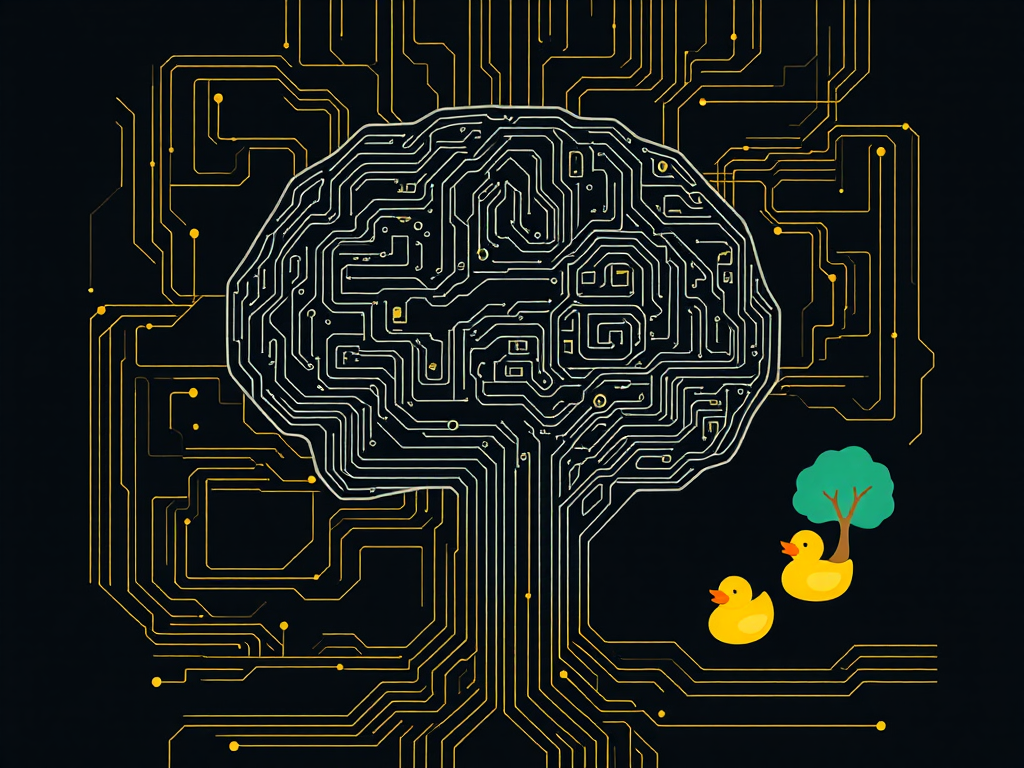