Debugging Overview
Have you encountered situations where your code works fine during writing but unexpected errors occur during runtime? When facing errors, the first reaction is often to add numerous print statements to locate the problem. In fact, Python provides many powerful debugging tools and techniques that can help us find and solve problems more efficiently.
As a Python developer, I frequently use these debugging techniques in my daily coding. Today I'd like to share my experience and insights with you.
Common Misconceptions
Many Python beginners fall into certain misconceptions when debugging. The most typical one is over-reliance on print debugging. While print is indeed simple and direct, it's inefficient in complex projects. For example:
def process_data(data):
print("Start processing data...")
result = []
for item in data:
print(f"Processing: {item}")
# Processing logic
processed = item * 2
print(f"Processing result: {processed}")
result.append(processed)
print(f"Final result: {result}")
return result
data = [1, 2, 3]
process_data(data)
Such code is filled with print statements, which not only affects code readability but also generates a lot of redundant output information. If the project scale is large, finding key information becomes like finding a needle in a haystack.
Breakpoint Debugging
Compared to print, using breakpoint debugging is a more professional approach. Python's built-in pdb module provides powerful breakpoint debugging capabilities:
import pdb
def calculate_total(items):
total = 0
for item in items:
pdb.set_trace() # Set breakpoint
total += item
return total
items = [10, 20, 30]
result = calculate_total(items)
You can control program execution through the following commands: - n (next): Execute next line - s (step): Step into function - c (continue): Continue execution until next breakpoint - p variable: Print variable value - l (list): Show code around current position
I think the advantage of breakpoint debugging is that you can view the state of all variables when the program is paused, and step through to track program flow. This is much more powerful than simple print statements.
Exception Handling
Besides debugging tools, proper exception handling is also key to improving code robustness. Let's look at a practical example:
def divide_numbers(a, b):
try:
result = a / b
except ZeroDivisionError:
print("Divisor cannot be zero")
return None
except TypeError:
print("Input must be numbers")
return None
else:
return result
finally:
print("Calculation completed")
print(divide_numbers(10, 2)) # Normal case
print(divide_numbers(10, 0)) # Zero division error
print(divide_numbers('a', 2)) # Type error
This example shows how to gracefully handle various possible exception cases. In real projects, proper exception handling can prevent program crashes while providing meaningful error messages.
Logging
For larger projects, using the logging module is a better choice than print:
import logging
logging.basicConfig(
level=logging.DEBUG,
format='%(asctime)s - %(levelname)s - %(message)s',
filename='app.log'
)
def process_user_data(user_id):
logging.info(f"Start processing data for user {user_id}")
try:
# Simulate data processing
if user_id < 0:
raise ValueError("User ID cannot be negative")
result = user_id * 2
logging.debug(f"Data processing result: {result}")
return result
except Exception as e:
logging.error(f"Error processing data for user {user_id}: {str(e)}")
raise
try:
process_user_data(-1)
except ValueError:
pass
The advantages of logging systems are: 1. Different logging levels can be set (DEBUG, INFO, WARNING, ERROR, CRITICAL) 2. Logs can be saved to files for later analysis 3. Can include timestamps and other context information 4. Doesn't affect normal code execution
Assertion Mechanism
Assertions are a form of defensive programming that can help us detect problems early:
def calculate_average(numbers):
assert isinstance(numbers, list), "Input must be a list"
assert len(numbers) > 0, "List cannot be empty"
assert all(isinstance(x, (int, float)) for x in numbers), "List elements must be numbers"
total = sum(numbers)
return total / len(numbers)
try:
print(calculate_average([1, 2, 3])) # Normal case
print(calculate_average([])) # Empty list
print(calculate_average(['a', 'b'])) # Non-numeric elements
print(calculate_average("not a list")) # Non-list input
except AssertionError as e:
print(f"Assertion error: {str(e)}")
The benefit of assertions is that potential problems can be discovered during development rather than waiting until runtime.
Performance Profiling
Sometimes we need to find performance bottlenecks in code, and this is where Profile tools come in handy:
import cProfile
import pstats
from pstats import SortKey
def fibonacci(n):
if n <= 1:
return n
return fibonacci(n-1) + fibonacci(n-2)
def profile_code():
# Create Profile object
profiler = cProfile.Profile()
# Execute profiling
profiler.enable()
fibonacci(30)
profiler.disable()
# Analyze results
stats = pstats.Stats(profiler)
stats.sort_stats(SortKey.TIME)
stats.print_stats()
profile_code()
Through performance profiling, we can see: - Number of times each function is called - Execution time of each function - Function call relationships
This is very helpful for optimizing code performance.
Debugging Tool Selection
Besides the tools mentioned above, there are many excellent debugging tools worth recommending:
- PyCharm's graphical debugger:
- Visual breakpoint management
- Variable viewer
-
Call stack analysis
-
VS Code + Python plugin:
- Lightweight
- Supports remote debugging
-
Integrated terminal
-
ipdb (IPython debugger):
- More friendly interface
- Code completion
- Syntax highlighting
Personally, I prefer using PyCharm's debugger because it provides a complete visual interface making debugging more intuitive. However, pdb or ipdb are often better choices when debugging on servers.
Best Practices
Based on my experience, I recommend the following debugging best practices:
- Layered debugging
- First ensure input data is correct
- Check if basic functionality works
-
Gradually dive into complex logic
-
Write unit tests ```python import unittest
def add_numbers(a, b): return a + b
class TestAddNumbers(unittest.TestCase): def test_positive_numbers(self): self.assertEqual(add_numbers(1, 2), 3)
def test_negative_numbers(self):
self.assertEqual(add_numbers(-1, -2), -3)
def test_zero(self):
self.assertEqual(add_numbers(0, 0), 0)
if name == 'main': unittest.main() ```
- Code review
- Have colleagues check your code
- Use static code analysis tools
-
Follow code standards
-
Documentation
- Record known issues
- Record solutions
- Update related documentation
Practical Case
Let's combine these debugging techniques in a practical example:
import logging
import time
from typing import List, Union
from functools import wraps
logging.basicConfig(level=logging.DEBUG,
format='%(asctime)s - %(levelname)s - %(message)s')
def monitor_performance(func):
@wraps(func)
def wrapper(*args, **kwargs):
start_time = time.time()
try:
result = func(*args, **kwargs)
end_time = time.time()
logging.debug(f"{func.__name__} execution time: {end_time - start_time:.4f} seconds")
return result
except Exception as e:
logging.error(f"{func.__name__} execution error: {str(e)}")
raise
return wrapper
class DataProcessor:
def __init__(self):
self.data = []
@monitor_performance
def process_batch(self, items: List[Union[int, float]]) -> List[float]:
"""Process a batch of data
Args:
items: List of numbers to process
Returns:
List of processed results
Raises:
ValueError: When input data is invalid
"""
assert isinstance(items, list), "Input must be a list"
assert all(isinstance(x, (int, float)) for x in items), "All elements must be numbers"
results = []
for item in items:
try:
# Simulate complex data processing
processed = item * 2.5
if processed < 0:
logging.warning(f"Processing result is negative: {processed}")
results.append(processed)
except Exception as e:
logging.error(f"Error processing {item}: {str(e)}")
raise
self.data.extend(results)
return results
if __name__ == '__main__':
processor = DataProcessor()
# Test normal case
try:
result1 = processor.process_batch([1, 2, 3])
print(f"Normal processing result: {result1}")
except Exception as e:
print(f"Processing error: {str(e)}")
# Test exception case
try:
result2 = processor.process_batch([1, 'a', 3])
print(f"Exception processing result: {result2}")
except Exception as e:
print(f"Processing error: {str(e)}")
This example demonstrates: 1. Using decorators for performance monitoring 2. Proper exception handling 3. Comprehensive logging 4. Type hints 5. Assertion checks 6. Documentation strings
Final Thoughts
Debugging is an essential part of programming, and mastering debugging techniques can: - Improve development efficiency - Reduce bugs - Enhance code quality - Lower maintenance costs
Which of these debugging techniques do you frequently use? Which ones haven't you tried yet? Feel free to share your experience and thoughts in the comments.
For your future programming journey, I suggest: 1. Develop systematic debugging thinking 2. Master various debugging tools 3. Establish your own debugging best practices 4. Continuously learn new debugging techniques
Let's write more robust and reliable Python code together.
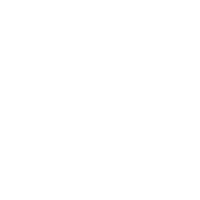
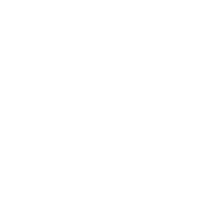