Hello, dear Python enthusiasts. Today, we're going to talk about Python debugging. As an experienced Python developer, I deeply understand the importance of debugging in programming. After all, writing code inevitably leads to errors, and the process of finding and fixing those errors is debugging. Have you ever been frustrated trying to solve a bug? Have you ever felt lost when facing a pile of error messages? Don't worry, today I'm going to share some practical Python debugging techniques with you to help you easily tackle various debugging challenges.
Importance
Before we dive into specific debugging techniques, I want to talk about the importance of debugging first. You might ask, "As long as the code runs, why spend so much time debugging?" This is a good question, let me explain.
First, debugging helps us find and fix errors in our code. This may seem obvious, but it's actually crucial. Imagine if your program has a hidden bug that causes it to crash or produce incorrect results under certain conditions. If you don't thoroughly debug, this bug might lurk in your code until it strikes at the worst possible moment.
Second, the debugging process helps us understand the working mechanisms of our code more deeply. As you step through the program execution and observe variable changes, you'll gain a clearer understanding of how the program works internally. This not only helps solve the current problem but also improves your programming skills, allowing you to write better code in the future.
Finally, debugging is an important means of improving code quality. Through debugging, you may discover potential performance issues or inelegant code structures. This provides opportunities for code optimization and refactoring, making your program more efficient and maintainable.
I remember one time when I was developing a data processing program, I encountered a strange bug. The program worked fine for most data, but occasionally produced incorrect results. If it weren't for careful debugging, I might never have found the root cause. It turned out to be a tiny logical error that only triggered under specific data combinations. This experience made me deeply realize the importance of debugging.
Common Methods
Now that we've covered the importance of debugging, let's look at some common debugging methods in Python. These methods have their own characteristics and are suitable for different scenarios.
- Using print statements
This is probably the simplest and most direct debugging method. By inserting print statements into our code, we can output variable values or information about reaching certain points during program execution. Although this method may seem primitive, it's very effective for quickly checking small issues.
```python def calculate_sum(a, b): print(f"Calculating sum of {a} and {b}") # Debug output result = a + b print(f"Result: {result}") # Debug output return result
print(calculate_sum(3, 4)) ```
- Using assertions (assert)
Assertions are a way to insert checkpoints into your code. If the assertion condition is false, the program will immediately raise an exception. This is useful for validating critical assumptions.
```python def divide(a, b): assert b != 0, "Divisor cannot be zero" return a / b
print(divide(10, 2)) # Normal print(divide(10, 0)) # Will raise AssertionError ```
- Using the Python debugger (pdb)
pdb is Python's built-in debugger, providing more powerful debugging features. You can set breakpoints, step through code, and inspect variable values.
```python import pdb
def complex_function(x, y): result = x * y pdb.set_trace() # Set a breakpoint return result * 2
print(complex_function(3, 4)) ```
- Using logging
Compared to print statements, the logging module provides more flexible and powerful logging capabilities. You can set different log levels to control the output detail.
```python import logging
logging.basicConfig(level=logging.DEBUG)
def calculate_average(numbers): logging.debug(f"Calculating average of {numbers}") total = sum(numbers) count = len(numbers) average = total / count logging.info(f"Average calculated: {average}") return average
print(calculate_average([1, 2, 3, 4, 5])) ```
These methods have their own pros and cons. Print statements are simple and direct, but they can produce a lot of output in large programs. Assertions are suitable for checking critical conditions but cannot provide detailed debugging information. pdb is powerful but has a steeper learning curve. Logging is flexible but requires some additional setup.
In real development, I often use a combination of these methods. For simple checks, I might quickly insert a few print statements. If I encounter more complex issues, I would use pdb for interactive debugging. For long-running programs, I would set up appropriate logging to monitor the program's execution status at any time.
Choosing which method depends on your specific needs and personal preferences. I suggest you try them all and find the debugging approach that suits you best. Remember, debugging is a skill that requires constant practice. As you gain experience, you'll find yourself getting better and better at finding and solving problems.
The pdb Module
When it comes to Python debugging, we can't ignore the pdb module. It's Python's built-in debugger, providing powerful interactive debugging features. Let's take a deep dive into how to use pdb for debugging.
Setting Breakpoints
The first step in using pdb is to set breakpoints. A breakpoint is a point in the program where execution pauses, allowing you to inspect the program's state. In pdb, there are several ways to set breakpoints:
- Using pdb.set_trace()
This is the simplest method. You just need to insert this line of code where you want the program to pause:
```python import pdb
def complex_calculation(x, y): result = x * y pdb.set_trace() # The program will pause here return result * 2
print(complex_calculation(3, 4)) ```
- Using the breakpoint() function (Python 3.7+)
Starting from Python 3.7, you can use the built-in breakpoint() function to set a breakpoint, which automatically calls pdb.set_trace():
```python def complex_calculation(x, y): result = x * y breakpoint() # Same as pdb.set_trace() return result * 2
print(complex_calculation(3, 4)) ```
- Setting breakpoints in the pdb prompt
If you're already in the pdb environment, you can use the b (break) command to set breakpoints:
(Pdb) b 10 # Set a breakpoint at line 10
(Pdb) b my_function # Set a breakpoint at the entry of my_function
Stepping Through Code
Once the program pauses at a breakpoint, you can start stepping through the code. pdb provides several commands to control program execution:
- n (next): Execute the current line, but do not step into function calls
- s (step): Execute the current line, stepping into function calls
- c (continue): Continue execution until the next breakpoint
- r (return): Continue execution until the current function returns
- q (quit): Quit the debugger
Here's an example:
def calculate_sum(a, b):
result = a + b
return result
def main():
x = 5
y = 7
breakpoint()
sum_result = calculate_sum(x, y)
print(f"The sum is: {sum_result}")
main()
When you run this code, the program will pause at the breakpoint() line. Then you can use pdb commands to explore the code:
-> sum_result = calculate_sum(x, y)
(Pdb) n
-> print(f"The sum is: {sum_result}")
(Pdb) p sum_result
12
(Pdb) c
The sum is: 12
During this process, you can use the p (print) command to inspect variable values and the l (list) command to view the code around the current position.
Using pdb for debugging might feel a bit complex at first, but with practice, you'll find it to be a very powerful tool. It allows you to precisely control program execution and gain a deep understanding of the program's internal state.
I remember one time when I was working on a complex data processing function and encountered an issue. By using pdb, I could step through the function's execution, observing the result of each calculation step. This helped me discover a subtle logical error that might have taken me much longer to find without this detailed debugging.
Logging
After discussing pdb, let's talk about another powerful debugging tool: logging. Python's logging module provides flexible and powerful logging capabilities that can help you better understand and monitor your programs.
Using the logging Module
Using the logging module is straightforward. First, you need to import the logging module and configure it:
import logging
logging.basicConfig(level=logging.DEBUG)
Then, you can use various logging functions in your code:
def complex_calculation(x, y):
logging.debug(f"Starting calculation with x={x}, y={y}")
result = x * y
logging.info(f"Intermediate result: {result}")
final_result = result * 2
logging.debug(f"Calculation complete. Final result: {final_result}")
return final_result
print(complex_calculation(3, 4))
Running this code, you'll see output similar to this:
DEBUG:root:Starting calculation with x=3, y=4
INFO:root:Intermediate result: 12
DEBUG:root:Calculation complete. Final result: 24
24
Logging Information Based on Importance
The logging module provides several logging functions at different levels of importance, in ascending order:
- debug(): Detailed debugging information
- info(): Confirmations that the program is running as expected
- warning(): Indications of potential issues
- error(): Due to more serious issues, some functionality has not been executed
- critical(): Severe errors, indicating the program may not be able to continue running
You can choose an appropriate level based on the importance of the information:
def divide(a, b):
logging.debug(f"Dividing {a} by {b}")
if b == 0:
logging.error("Cannot divide by zero!")
return None
result = a / b
logging.info(f"Division result: {result}")
return result
print(divide(10, 2))
print(divide(10, 0))
The output of this code might look like this:
DEBUG:root:Dividing 10 by 2
INFO:root:Division result: 5.0
5.0
DEBUG:root:Dividing 10 by 0
ERROR:root:Cannot divide by zero!
None
By using different log levels, you can control the level of detail in the output. During development, you might want to see all debugging information. In production environments, you might only want to log warnings and errors.
I personally love using the logging module. It's not only useful for debugging but can also help you monitor long-running programs. I once developed a data processing pipeline that needed to run for a long time to process large amounts of data. By setting up appropriate logging, I could check the program's progress and see if any issues arose at any time. This greatly improved my work efficiency.
Remember, good logging practices can make your code more understandable and maintainable. Don't be stingy about adding log statements to your code; they might save you a lot of time and effort in the future.
Print Statements
When it comes to debugging, we can't overlook the simplest and most direct method: using print statements. Although it may seem a bit "primitive," print statements are still a quick and effective debugging tool in many situations. Let's look at how to optimize the use of print statements for debugging.
Define a debug_print Function
While directly using print statements is convenient, it has an obvious drawback: when you no longer need these debug outputs, you need to manually comment out or remove all the print statements. This is not only tedious but also error-prone. A better approach is to define a dedicated debug_print function:
DEBUG = True # Global switch
def debug_print(*args, **kwargs):
if DEBUG:
print(*args, **kwargs)
def complex_calculation(x, y):
debug_print(f"Starting calculation with x={x}, y={y}")
result = x * y
debug_print(f"Intermediate result: {result}")
final_result = result * 2
debug_print(f"Final result: {final_result}")
return final_result
print(complex_calculation(3, 4))
This way, you only need to modify the value of the DEBUG variable to control whether debug information is printed or not. When DEBUG is True, all debug_print calls will output information; when DEBUG is False, these calls won't produce any output.
Using the pprint Module to Print Complex Data Structures
When you need to print complex data structures (such as nested dictionaries or lists), the regular print function might not be very useful. This is where the pprint (pretty print) module comes in handy:
from pprint import pprint
complex_data = {
'name': 'John',
'age': 30,
'skills': ['Python', 'JavaScript', 'SQL'],
'work_experience': [
{'company': 'Tech Corp', 'years': 2},
{'company': 'Innovative Solutions', 'years': 3}
]
}
print("Using regular print:")
print(complex_data)
print("
Using pprint:")
pprint(complex_data)
Running this code, you'll see that pprint provides a clearer and more readable output format, especially for nested data structures.
I often use these techniques in my daily programming. The debug_print function, in particular, allows me to freely add debug output to my code without worrying about having to clean up the code later. When I need to quickly check a variable's value or confirm that the code has reached a certain point, this function is very useful.
As for pprint, it's my go-to helper when dealing with complex data structures. Once, when I was processing a complex JSON data from an API, the regular print output gave me a headache. Using pprint made the data structure clear and visible, helping me quickly find the issue.
Remember, although print debugging seems simple, it's still a powerful tool. The key is to use it flexibly and choose the most appropriate method for the specific situation. Sometimes, a simple print statement might help you find the problem faster than a complex debugger.
Stack Traces
In Python debugging, stack traces are a crucial concept. When a program encounters an error, the stack trace can help you understand where the error occurred and the sequence of calls that led to the error. Let's delve into how to use stack traces for debugging.
Using the traceback Module
Python's traceback module provides a standard way to extract, format, and print stack traces from Python programs. Here's a simple example:
import traceback
def function_c():
raise Exception("Something went wrong in function_c")
def function_b():
function_c()
def function_a():
function_b()
try:
function_a()
except Exception:
print("An error occurred. Here's the traceback:")
traceback.print_exc()
Running this code, you'll see output similar to this:
An error occurred. Here's the traceback:
Traceback (most recent call last):
File "example.py", line 13, in <module>
function_a()
File "example.py", line 10, in function_a
function_b()
File "example.py", line 7, in function_b
function_c()
File "example.py", line 4, in function_c
raise Exception("Something went wrong in function_c")
Exception: Something went wrong in function_c
This stack trace clearly shows the source of the error (function_c) and the call chain that led to the error (function_a -> function_b -> function_c).
Interpreting Stack Trace Information
A stack trace contains a lot of useful information:
- Error type: In this example, it's an Exception.
- Error message: "Something went wrong in function_c".
- Call chain: Starting from the bottom-most function_c up to the top-level
. - File name and line number for each call: This can help you quickly locate the specific code position.
Understanding and interpreting stack traces is an important debugging skill. When you're faced with a complex error, carefully reading the stack trace can help you quickly pinpoint the issue.
I remember one time when I was working on a large project, I encountered a hard-to-understand error. The error message itself wasn't very helpful, but by carefully analyzing the stack trace, I found that the error was actually caused by a deeply nested function call. Without the stack trace, it might have taken me much longer to locate the problem.
Here are some tips for using stack traces:
- Read from the bottom up: Errors usually occur at the bottom of the stack and propagate upwards.
- Pay attention to your own code: Stack traces may include calls from internal libraries, but you're usually more interested in the code you wrote yourself.
- Use your IDE's jump-to-code feature: Many IDEs allow you to jump directly from the stack trace to the corresponding code line.
Remember, stack traces are not only useful for handling errors but can also help you understand a program's execution flow. Sometimes, even if a program doesn't throw an exception, you might want to inspect the current call stack. In such cases, you can use the traceback.print_stack() function:
import traceback
def function_d():
print("Current call stack:")
traceback.print_stack()
def function_e():
function_d()
function_e()
This will print the current call stack, helping you understand how the program reached its current position.
Overall, stack traces are a powerful debugging tool. They can not only help you find errors but also help you understand a program's execution flow. Becoming proficient in using stack traces can greatly improve your debugging efficiency.
IDE Debugging
When it comes to Python debugging, we can't overlook the powerful debugging tools provided by Integrated Development Environments (IDEs). Modern IDEs not only offer code editing and execution capabilities but also integrate advanced debugging features. Let's look at how to leverage IDEs for efficient Python debugging.
Common IDE Debugging Features
- Breakpoint Setting
Almost all IDEs support setting breakpoints. You can add or remove breakpoints by clicking on the blank space next to the line numbers. When the program reaches a breakpoint, it will automatically pause, allowing you to inspect the program's state.
- Step Execution
IDEs typically provide several ways to step through code execution: - Step Into: Execute the current line, and if it's a function call, step into the function. - Step Over: Execute the current line, but if it's a function call, execute the entire function without stepping into it. - Step Out: Execute until the current function returns.
- Variable Inspection
In debug mode, IDEs display the values of all variables in the current scope. You can expand complex data structures like lists or dictionaries to view their internal structure.
- Watch Expressions
You can add watch expressions, and the IDE will continuously evaluate and display their values. This is useful for tracking complex computations.
- Call Stack Inspection
IDEs show the current call stack, allowing you to easily switch between different stack frames and inspect local variables for each function.
- Conditional Breakpoints
You can set conditional breakpoints that only trigger when specific conditions are met. This is particularly useful when debugging loops or bugs that only occur under certain circumstances.
Advantages of GUI Debugging
Compared to command-line debugging tools (like pdb), GUI debugging in IDEs offers the following advantages:
- Intuitiveness
The graphical interface allows you to visually observe the program's execution flow, variable values, and call stack. You don't need to remember complex commands; you can control program execution by clicking buttons.
- Efficiency
In an IDE, you can quickly switch between code and debugging information. For example, you can jump directly from the call stack to the corresponding code line. This greatly improves debugging efficiency.
- Rich Information Display
IDEs can simultaneously display various debugging information, such as variable values, watch expressions, and call stacks. This information is presented in a structured way, making it easier for you to quickly access the information you need.
- Integration with Other Development Tools
IDE debuggers are often integrated with other development tools (like version control systems and testing frameworks), providing a one-stop development experience.
Personally, I love using PyCharm for Python development and debugging. I remember one time when I was working on a complex data processing task and encountered an issue. With PyCharm's debugging tools, I could easily set breakpoints, step through the code, and inspect variable values in real-time. This helped me quickly locate the problem – it turned out to be a misspelled dictionary key. Without the IDE's help, finding this small error might have taken me much more time.
When using an IDE for debugging, I have a few tips to share:
-
Make good use of conditional breakpoints. When you're only interested in the program's behavior under specific conditions, conditional breakpoints can save you a lot of time.
-
Leverage watch expressions. Sometimes you might be interested in the value of a complex expression that doesn't directly appear in your code. In such cases, adding a watch expression can be very useful.
-
Learn to modify variable values in debug mode. Many IDEs allow you to directly modify variable values during the debugging process. This is useful for quickly testing "what if" scenarios.
-
Use exception breakpoints. You can set breakpoints to pause the program when a specific type of exception occurs, which is helpful for catching unexpected exceptions.
Remember, although IDE debugging tools are powerful, they cannot completely replace other debugging methods. Sometimes, a simple print statement might help you find the problem faster than a complex IDE debugging session. The key is to choose the most appropriate tool for the specific situation.
Overall, IDE debugging tools greatly improve development efficiency, especially when dealing with complex projects. If you haven't fully utilized your IDE's debugging features, I strongly recommend taking some time to learn and practice them. I'm confident you'll find that they make your programming journey smoother and more enjoyable.
Conclusion
Well, dear Python enthusiasts, our journey through Python debugging techniques is coming to an end. Let's review the important things we've learned today:
-
We discussed the importance of debugging, which not only helps us find and fix errors but also deepens our understanding of our code.
-
We learned several common debugging methods, including using print statements, assertions, the Python debugger (pdb), and logging.
-
We explored the use of the pdb module in depth, learning how to set breakpoints and step through code.
-
We understood the powerful capabilities of the logging module and learned how to log information based on importance levels.
-
We optimized the use of print statements, learning to define a debug_print function and use the pprint module to print complex data structures.
-
We learned how to interpret stack trace information, which is extremely helpful for quickly locating errors.
-
Finally, we discussed the powerful debugging features of IDEs and understood the advantages of GUI debugging.
Remember, debugging is a skill that requires constant practice. The techniques you learned today might not make you a debugging master immediately, but they provide you with a solid foundation. As you continue on your programming journey, you'll find yourself becoming better and better at finding and solving problems.
I want to encourage you not to be afraid of encountering bugs. Every bug is an opportunity to learn, and they can help you gain a deeper understanding of Python and programming principles. When you encounter difficulties, don't get discouraged; instead, maintain your curiosity and spirit of exploration. Try applying the techniques we learned today, and I'm confident you'll find solutions.
Finally, I'd like to ask you: Which debugging technique did you find most useful from today's content? Do you have any favorite debugging techniques of your own that you'd like to share? Feel free to leave comments, and let's exchange ideas and progress together.
Remember, the joy of programming lies not only in writing beautiful code but also in the process of solving problems. Enjoy the debugging process, because with every bug you solve, you're one step closer to becoming a better programmer.
Well, that's it for today's sharing. I hope these debugging techniques will help you go further on your Python programming journey. Until next time, happy coding, and may the bugs stay away!
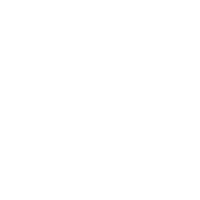
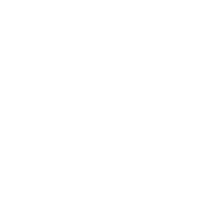