Getting to Know Python
Have you ever been curious about programming, but felt it was too complex? Don't worry, let's get to know Python, this magical programming language together!
Python is a simple yet powerful programming language. Its syntax is clear and straightforward, as natural as writing an essay in English. Did you know? Python's name actually comes from the Monty Python comedy troupe. Doesn't that make it seem more approachable?
I remember when I first encountered Python, I was attracted by its conciseness. For example, to print "Hello, World!", you only need to write:
print("Hello, World!")
It's that simple! Compared to other languages that require several lines of code to accomplish the same task, Python is like a shot of adrenaline for programming beginners!
Playing with Variables
In Python, variables are like little boxes where you can put all sorts of things. For example:
name = "Xiaoming"
age = 18
height = 1.75
is_student = True
Look, we just created four variables! name
is a string type, age
is an integer type, height
is a float type, and is_student
is a boolean type. Python automatically determines the type of the variable, you don't need to declare it in advance. Isn't that convenient?
I think understanding the concept of variables is crucial for programming. You can imagine it as your backpack, where you can put all sorts of things and take them out when needed. Thinking of it this way, programming doesn't seem so daunting, does it?
Conditional Statements
In life, we often need to make various judgments. For example, "If it rains today, I'll bring an umbrella." In Python, we use the if
statement to express this kind of logic:
weather = "rainy"
if weather == "rainy":
print("Remember to bring an umbrella!")
elif weather == "sunny":
print("Go out and play!")
else:
print("Decide based on the situation.")
Doesn't this code look a lot like how we normally speak? If the weather is rainy, it reminds us to bring an umbrella; if it's sunny, it suggests going out to play; if it's another weather condition, it suggests deciding based on the situation.
I think the beauty of the if
statement lies in the fact that it gives the computer the ability to "think." You can use it to simulate various complex decision-making processes. Imagine if you were a game developer, you could use the if
statement to control the behavior of game characters. Isn't that cool?
Loop Fun
Sometimes, we need to repeat a task. For example, printing out the numbers from 1 to 10. If we had to write them out one by one, that would be too much trouble, right? This is where loops come in handy:
for i in range(1, 11):
print(i)
Look, with just two lines of code, we printed out the numbers from 1 to 10! range(1, 11)
generates a sequence from 1 to 10 (note that it doesn't include 11), and then the for
loop iterates through each number in the sequence, assigning it to the variable i
, and executing the indented code block.
I personally love using loops to solve problems. It's like giving the computer an instruction: "Follow this rule, repeatedly execute this task until it's done." This way of thinking is very useful in programming, and you can use it to process large amounts of data or implement complex algorithms.
Function Magic
Functions are a very important concept in Python. You can think of it as a little machine: you give it some input, and it gives you the corresponding output. For example, let's define a function to calculate the area of a circle:
import math
def calculate_circle_area(radius):
return math.pi * radius ** 2
area = calculate_circle_area(5)
print(f"The area of a circle with radius 5 is: {area:.2f}")
This function takes a parameter radius
(the radius), and then returns the area of the circle. We used math.pi
to get the value of π, and the **
operator represents exponentiation.
The power of functions lies in the fact that you can call them from anywhere in your program without having to repeat the same code. This not only makes your program neater but also easier to maintain and debug.
I remember when I first learned functions, I found them a bit difficult to understand. But later, I realized that functions are like "recipes" in our daily lives. You give them ingredients (parameters), and they follow a set of steps (function body) to make a delicious dish (return value). Thinking of it this way, functions don't seem so mysterious anymore, do they?
List Operations
Lists are one of the most commonly used data structures in Python. You can think of it as a container that can hold multiple elements. For example:
fruits = ["apple", "banana", "orange", "grape"]
print(fruits[0]) # Output: apple
fruits[1] = "pear"
fruits.append("watermelon")
fruits.remove("orange")
for fruit in fruits:
print(fruit)
The power of lists lies in their flexibility. You can add, remove, or modify elements at any time. Plus, Python provides many built-in functions and methods for operating on lists, allowing you to easily perform various complex tasks.
I think mastering list operations is crucial for data processing. Imagine if you were a data analyst, you might need to process thousands of data records. In that case, if you're proficient in using lists, you can easily organize and analyze that data.
Dictionary Magic
Dictionaries are another very useful data structure. They allow you to store data in key-value pairs. For example:
student = {
"name": "Xiaoming",
"age": 18,
"grades": {"math": 90, "english": 85, "physics": 95}
}
print(student["name"]) # Output: Xiaoming
student["age"] = 19
student["hobby"] = "basketball"
for key, value in student.items():
print(f"{key}: {value}")
The advantage of dictionaries is that they allow you to organize data in a more intuitive way. You can use meaningful names as keys, making it easier to access and modify data.
I personally love using dictionaries because they make the code more readable and self-explanatory. For example, student["name"]
is much more intuitive than student[0]
, don't you think? Furthermore, dictionaries allow you to nest complex data structures, just like the grades
example above.
Exception Handling
In the process of programming, you'll inevitably encounter various errors, such as attempting to divide by zero or accessing a non-existent file. This is where exception handling comes in handy:
try:
number = int(input("Please enter a number: "))
result = 10 / number
print(f"10 divided by {number} is: {result}")
except ValueError:
print("Invalid input, please enter a number.")
except ZeroDivisionError:
print("Cannot divide by zero.")
except Exception as e:
print(f"An unknown error occurred: {e}")
else:
print("Calculation completed successfully!")
finally:
print("Program execution finished.")
This code demonstrates how to handle various exceptions that may occur. The try
block contains code that might cause an error, the except
block catches and handles specific types of exceptions, the else
block executes if no exceptions occur, and the finally
block executes regardless of whether an exception occurred or not.
I think learning to handle exceptions is key to becoming an excellent programmer. It not only makes your program more robust but also helps you better understand and debug your code. Remember when I first started learning programming, I was often scared by various error messages. But later, I realized that these error messages are actually the program telling you where the problem is – they're your best teachers!
File Operations
In practical programming, we often need to read and write files. Python provides simple yet powerful file operation capabilities:
with open("example.txt", "w") as file:
file.write("Hello, World!
")
file.write("This is an example file.
")
with open("example.txt", "r") as file:
content = file.read()
print(content)
with open("example.txt", "r") as file:
for line in file:
print(line.strip())
The with
statement ensures that the file is properly closed after use, which is a good programming practice. The "w"
mode indicates writing (which will overwrite existing content), and the "r"
mode indicates reading.
File operations allow your program to interact with the outside world. You can use them to save program results, read configuration files, or process large amounts of data. I remember once I wrote a small Python program that could automatically organize my photo files by shooting date, categorizing them into different folders. File operations played a crucial role in this process.
Module Imports
One of Python's great advantages is the abundance of third-party libraries available for use. You can extend Python's functionality by importing modules:
import random
import math
from datetime import datetime
print(random.randint(1, 100))
print(math.sqrt(16))
print(datetime.now())
Here, we imported the random
, math
, and datetime
modules. The random
module is used for generating random numbers, the math
module provides various mathematical functions, and the datetime
module is used for handling dates and times.
I think learning to use modules is key to improving programming efficiency. Why reinvent the wheel? The Python community has already prepared various "wheels" for us; we just need to learn how to use them. For instance, if you want to do data analysis, pandas
and numpy
are essential; if you want to do web scraping, requests
and beautifulsoup
will make your job much easier.
Object-Oriented Programming
Object-oriented programming is a very powerful programming paradigm. In Python, everything is an object. Let's look at a simple example:
class Dog:
def __init__(self, name, age):
self.name = name
self.age = age
def bark(self):
print(f"{self.name} barks!")
def celebrate_birthday(self):
self.age += 1
print(f"{self.name} is now {self.age} years old!")
my_dog = Dog("Wangcai", 3)
my_dog.bark()
my_dog.celebrate_birthday()
In this example, we defined a Dog
class. It has two attributes (name
and age
) and two methods (bark
and celebrate_birthday
). __init__
is a special method used to initialize objects.
Object-oriented programming allows us to organize code in a more intuitive and natural way. You can think of a class as a template, and objects are instances created from that template. This way of thinking is particularly suitable for modeling real-world objects and concepts.
I remember when I first learned object-oriented programming, I found it a bit abstract. But later, I realized that if you think of a class as a description of a kind of thing, and methods as the things that kind of thing can do, it becomes much easier to understand. For example, the Dog
class describes the characteristics and behaviors of dogs as animals. Don't you think this way of programming is similar to how we think about problems?
Decorator Tricks
Decorators are a fun feature in Python. They allow you to add new functionality to a function without modifying the original function. Let's look at an example:
import time
def timer(func):
def wrapper(*args, **kwargs):
start_time = time.time()
result = func(*args, **kwargs)
end_time = time.time()
print(f"{func.__name__} function run time: {end_time - start_time:.5f} seconds")
return result
return wrapper
@timer
def slow_function():
time.sleep(2)
print("This is a time-consuming function")
slow_function()
In this example, we defined a timer
decorator that can calculate the run time of the decorated function. Then, we applied this decorator to the slow_function
using the @timer
syntax.
The power of decorators lies in the fact that they allow you to add new functionality to a function without changing the original code. This not only improves code reusability but also makes your program more modular and easier to maintain.
I think understanding and using decorators is a milestone in Python programming. It signifies that you're starting to think about how to write more elegant and efficient code. You can use decorators to implement logging, performance testing, permission checking, and various other functionalities. Isn't that cool?
Generator Magic
Generators are another powerful feature in Python. They allow you to generate values one by one during iteration, rather than generating all values at once. This is particularly useful when dealing with large amounts of data. Let's look at an example:
def fibonacci_generator():
a, b = 0, 1
while True:
yield a
a, b = b, a + b
fib = fibonacci_generator()
for i in range(10):
print(next(fib))
This generator generates the Fibonacci sequence. The yield
keyword returns a value and saves the function's state, so the next call will continue from where it left off.
The advantage of generators is that they can save memory. Imagine if you need to process a list with millions of elements – if you generate all the elements at once, it might consume a lot of memory. But with a generator, you can generate elements on-demand, greatly reducing memory usage.
I personally love using generators because they make my code more efficient and elegant. Especially when dealing with large data sets or performing complex calculations, generators are a lifesaver! Have you ever experienced a program crash due to too much data? Next time, you can try using a generator to solve this problem.
Concurrent Programming
In modern programming, concurrent programming is becoming increasingly important. Python provides several ways to achieve concurrency, including multi-threading, multi-processing, and asynchronous programming. Let's look at an example using multi-threading:
import threading
import time
def worker(name):
print(f"Thread {name} started working")
time.sleep(2)
print(f"Thread {name} finished working")
threads = []
for i in range(5):
t = threading.Thread(target=worker, args=(f"Thread-{i}",))
threads.append(t)
t.start()
for t in threads:
t.join()
print("All threads completed")
This example creates 5 threads, each executing the worker
function. threading.Thread
is used to create threads, start()
method is used to start a thread, and join()
method is used to wait for a thread to complete.
Concurrent programming can greatly improve program efficiency, especially when dealing with I/O-intensive tasks. Imagine if your program needs to handle multiple network requests simultaneously – using concurrent programming allows your program to process other requests while waiting for one request, thereby improving overall efficiency.
I remember when I first tried concurrent programming, it felt like opening the door to a new world. Suddenly, my program could do multiple things at once! However, keep in mind that concurrent programming also brings new challenges, such as how to handle shared resources and how to avoid deadlocks. It's like upgrading from a single-player game to a multiplayer game – exciting and challenging at the same time.
Network Programming
In this internet age, network programming is becoming increasingly important. Python provides powerful network programming support, allowing us to easily create network applications. Here's an example of a simple HTTP server:
from http.server import HTTPServer, SimpleHTTPRequestHandler
import socketserver
PORT = 8000
Handler = SimpleHTTPRequestHandler
with socketserver.TCPServer(("", PORT), Handler) as httpd:
print(f"Server running on port {PORT}")
httpd.serve_forever()
This simple HTTP server will run on local port 8000 and serve files from the current directory as the root directory. You can access http://localhost:8000
in your browser to see the effect.
The charm of network programming lies in the fact that it allows your program to communicate with other programs around the world. You can use it to create website backends, develop APIs, implement remote control, and more. I remember the first time I successfully created a Python application that could be accessed over the network – the sense of accomplishment was indescribable. Suddenly, my program was no longer limited to my own computer but could be accessed by people all over the world!
Of course, network programming also brings new challenges, such as how to ensure communication security and how to handle large numbers of concurrent connections. But don't worry, the Python community has already provided us with many mature libraries and frameworks to solve these problems, such as Flask, Django, and more. You just need to stand on the shoulders of giants, and you can easily create powerful network applications.
Data Analysis
Python has widespread applications in the field of data analysis. With the help of powerful third-party libraries such as NumPy, Pandas, and Matplotlib, we can easily process and visualize large amounts of data. Let's look at a simple example:
import pandas as pd
import matplotlib.pyplot as plt
data = {
'Name': ['Zhangsan', 'Lisi', 'Wangwu', 'Zhaoliu', 'Qianqi'],
'Age': [25, 30, 35, 40, 45],
'Salary': [5000, 6000, 7000, 8000, 9000]
}
df = pd.DataFrame(data)
average_salary = df['Salary'].mean()
print(f"Average salary: {average_salary}")
plt.figure(figsize=(10, 5))
plt.bar(df['Name'], df['Salary'])
plt.title('Employee Salary Situation')
plt.xlabel('Name')
plt.ylabel('Salary')
plt.show()
This example demonstrates how to use Pandas to process data and how to use Matplotlib to plot charts. We created a simple dataset, calculated the average salary, and plotted a salary bar chart.
The charm of data analysis lies in its ability to extract valuable information from massive amounts of data. You can use it to analyze company sales data, study stock market trends, explore scientific experimental results, and more. I remember the first time I successfully analyzed a large dataset with Python – the feeling of uncovering patterns behind the data was fantastic!
Moreover, Python's data analysis ecosystem is incredibly rich. In addition to the Pandas and Matplotlib we just used, there are many other powerful tools, such as SciPy for scientific computing, Scikit-learn for machine learning, and more. These tools have made Python one of the preferred languages for data scientists.
Web Scraping in Action
Web scraping is another popular application area for Python. It can help us automatically extract information from web pages. Let's look at a simple web scraping example:
import requests
from bs4 import BeautifulSoup
url = "https://news.qq.com/"
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
news_titles = soup.find_all('h3', class_='tit')
for title in news_titles:
print(title.text.strip())
This example demonstrates how to use the requests library to send HTTP requests and how to use the BeautifulSoup library to parse HTML and extract information. We scraped the homepage of Tencent News and extracted all the news titles.
The power of web scraping lies in its ability to help us automatically collect large amounts of information. You can use it to monitor product prices, collect social media data, scrape website content, and more. I remember the first time I successfully scraped data from a large website – the sense of accomplishment was indescribable. Suddenly, I could easily access a large amount of information that previously had to be collected manually!
Of course, when using web scrapers, we need to be mindful of a few issues. First, we need to respect the website's robots.txt file and terms of use. Second, we need to control the scraping speed to avoid putting too much pressure on the target website. Finally, for some complex websites, we may need to handle JavaScript rendering, captchas, and other issues. But don't worry, the Python community has already provided us with many tools to solve these problems, such as Selenium, Scrapy, and more.
Introduction to Machine Learning
Machine learning is one of the hottest technology fields today, and Python is the primary programming language for machine learning. Let's look at a simple machine learning example:
from sklearn import datasets
from sklearn.model_selection import train_test_split
from sklearn.neighbors import KNeighborsClassifier
from sklearn.metrics import accuracy_score
iris = datasets.load_iris()
X = iris.data
y = iris.target
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42)
knn = KNeighborsClassifier(n_neighbors=3)
knn.fit(X_train, y_train)
y_pred = knn.predict(X_test)
accuracy = accuracy_score(y_test, y_pred)
print(f"Model accuracy: {accuracy:.2f}")
This example demonstrates how to use the scikit-learn library for machine learning. We used the famous Iris dataset, trained a K-Nearest Neighbors classifier to predict the species of iris flowers.
The charm of machine learning lies in its ability to allow computers to learn from data and make intelligent decisions. You can use it to predict stock prices, recognize images, recommend products, and more. I remember the first time I successfully trained a model that could make accurate predictions – the feeling was like creating a small artificial intelligence!
Python's advantage in the field of machine learning is evident. It has a wealth of libraries and frameworks, such as scikit-learn, TensorFlow, PyTorch, and more, which greatly lower the barrier to entry for machine learning. Plus, Python's syntax is concise and clear, allowing us to focus on algorithms and models rather than being bogged down by complex syntax.
Of course, machine learning is a profound field, and we've only scratched the surface here. If you're interested in machine learning, there's much more to learn in-depth, such as deep learning, reinforcement learning, and more. But don't be intimidated by these advanced terms – remember, every expert started as a beginner. As long as you maintain your curiosity and passion for learning, you too can become an expert in the field of machine learning!
Summary and Outlook
Wow, we've covered many aspects of Python together, from basic syntax to advanced applications. Can you feel Python's charm? It's concise yet powerful, capable of solving everyday small problems as well as developing complex systems.
Let's review what we've learned: We started with Python's basic syntax, learning fundamental concepts like variables, conditional statements, and loops. Then we delved into more complex structures like functions, lists, and dictionaries. Next, we learned advanced features like object-oriented programming, decorators, and generators. Finally, we touched on practical application areas such as concurrent programming, network programming, data analysis, web scraping, and machine learning.
Did you know? What we've covered is just the tip of the iceberg in the Python world. Python's application scope is continuously expanding, from web development to scientific computing, from game development to artificial intelligence – Python is almost omnipotent.
So, which direction would you like to study further? Do you want to become a data scientist, delving into data analysis and machine learning? Or perhaps you're interested in becoming a backend developer, focusing on web development and server-side programming? Or maybe you're interested in cybersecurity and want to learn how to use Python for penetration testing?
No matter which direction you choose, remember that the most important thing in learning programming is practice. Write more code, do more projects, and when you encounter problems, look up resources or ask others. Believe me, when you solve a problem that has been bothering you for a long time, the sense of accomplishment is unparalleled.
Finally, I want to say that programming is not just a skill but also a way of thinking. It teaches us how to break down complex problems into smaller, manageable parts and how to abstract and model real-world problems. These abilities are not only useful in programming but also in our daily lives and work.
So, let's continue our Python journey! Remember, in the world of programming, the only limit is your imagination. Are you ready to create something?
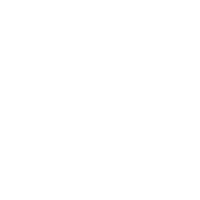
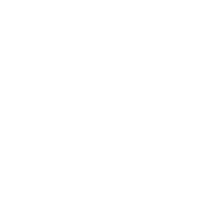
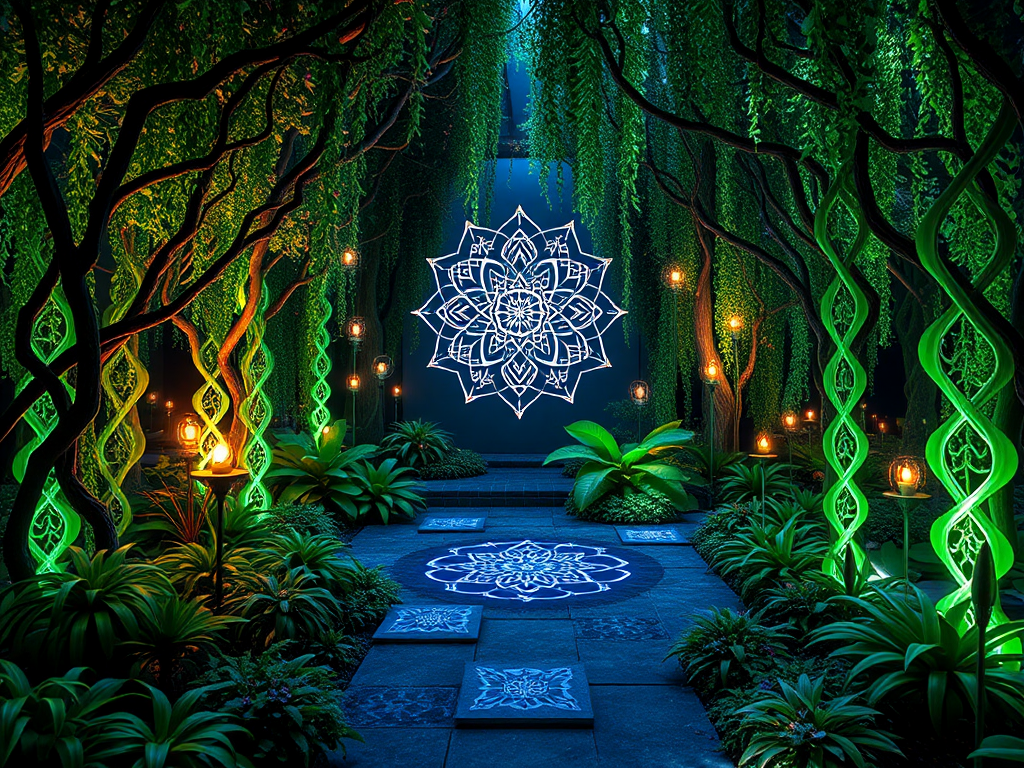
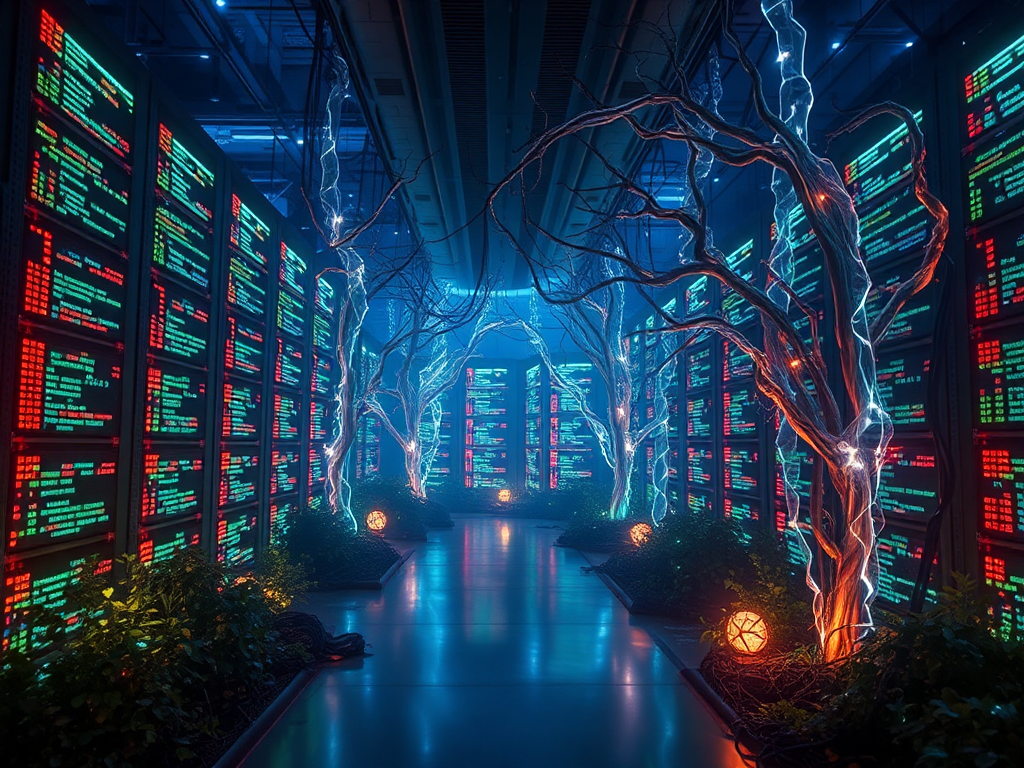
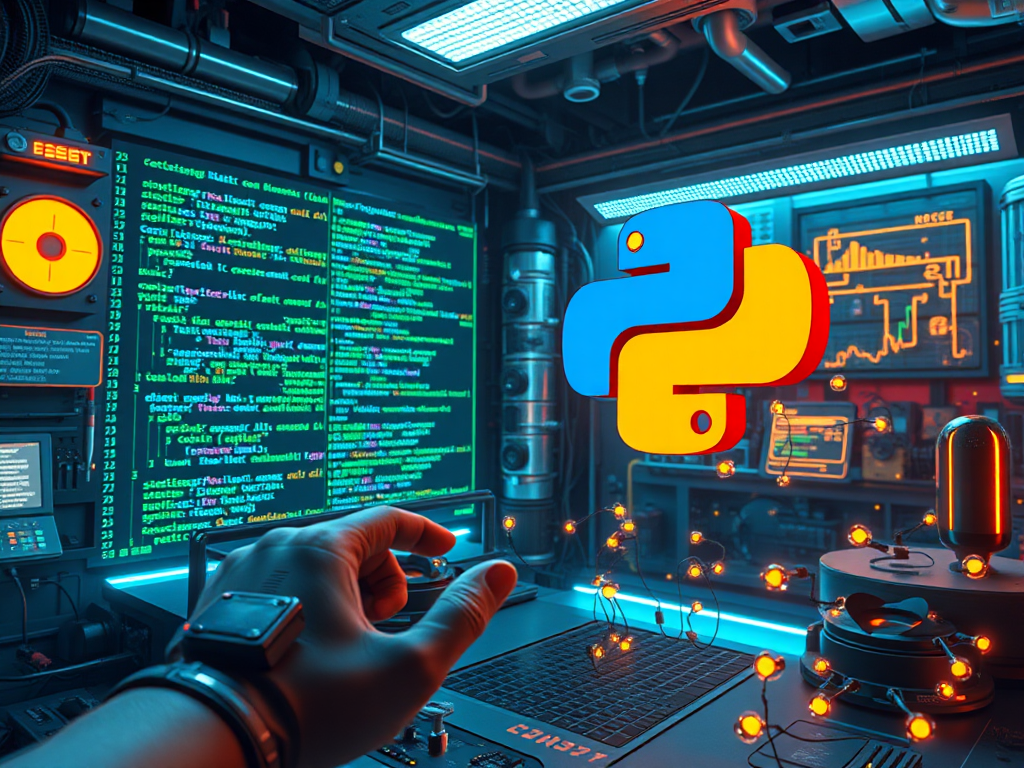