Hello everyone, today let's talk about Python debugging. As a Python programmer, are you often tormented by all kinds of weird bugs? Don't worry, today I'm going to share with you some practical debugging techniques that will leave those annoying bugs nowhere to hide!
The Print Method
When it comes to debugging, the simplest and most direct method is using print statements. This is our programmers' "primitive weapon", simple and crude but surprisingly effective.
Have you ever encountered this situation: you wrote a long piece of code, but it reported an error when running, and you had no idea where the error was? This is where strategic print statements come in handy.
Let's look at an example:
def factorial(n):
print(f"Calculating factorial({n})") # Track function entry
if n == 0:
print("Returning 1") # Track base case
return 1
else:
result = n * factorial(n-1)
print(f"When n = {n}, returning {result}") # Track intermediate results
return result
factorial(5)
See? By inserting print statements at key positions, we can clearly see the execution path of the function and intermediate calculation results. This is especially helpful for understanding recursive functions.
However, overusing print also has its drawbacks. If your code is full of print statements everywhere, it might end up in a mess. So, moderation is important.
Debugger to the Rescue
If you feel print statements are not powerful enough, then it's time for Python's built-in debugger pdb to take the stage. pdb allows you to trace the execution of your code step by step like a detective.
Let's see how to use it:
import pdb
def buggy_function(x):
pdb.set_trace() # Set breakpoint
y = x * 2
z = y / 0 # Deliberately create an error
return z
buggy_function(10)
When the code runs to the pdb.set_trace() line, the program will pause and enter pdb interactive mode. Here, you can use various commands to inspect variables, execute code, and even modify the program state.
For example: - Enter n (next) to execute the current line and move to the next line - Enter s (step) to step into function calls - Enter p variable_name to print the value of a variable - Enter c (continue) to continue execution until the next breakpoint
I personally think that pdb is like giving you a time pause button, allowing you to stop at any moment during code execution and carefully observe the internal state of the program. This is particularly useful for tracking complex logical errors.
Logging
If you're developing a large project, you might find that print statements and pdb are not quite enough. This is when you should consider using the logging module.
The logging module allows you to record information of different severity levels, and it's convenient to output logs to files or other places. Let's look at an example:
import logging
logging.basicConfig(level=logging.DEBUG, filename="debug.log")
def my_function():
logging.debug("Entering my_function")
# ... your code ...
logging.info("Function completed successfully")
my_function()
This code will record log information of debug level and above to a file named debug.log. You can set different log levels as needed, such as DEBUG, INFO, WARNING, ERROR, CRITICAL, etc.
I think a big advantage of the logging module is that you can enable detailed logging during development, and only record important information in the production environment, without needing to modify the code. This flexibility is especially useful in large projects.
Assertions for Protection
Assertions (assert) are another powerful debugging tool. They can help you set some "checkpoints" in your code to ensure certain conditions must be met. If the condition is not met, an exception will be thrown immediately.
Let's look at an example:
def calculate_area(length, width):
assert length > 0 and width > 0, "Length and width must be positive numbers"
return length * width
area = calculate_area(-5, 10) # This will raise an AssertionError
In this example, if someone tries to calculate an area with negative numbers, the program will stop immediately and report an error. This is much better than waiting until a meaningless negative area is calculated, right?
I personally like using assertions because they can help you discover and locate problems early. Moreover, assertions can also serve as self-documentation for the code, clearly indicating the preconditions of functions.
Advanced Toolbox
In addition to these basic techniques, there are some more professional tools worth mentioning:
-
Memory profilers (such as memory_profiler): Can help you find memory leak issues.
-
Performance profiling tools (such as cProfile): Can help you find performance bottlenecks in your code.
-
Code analysis tools (such as pylint, flake8): Can help you discover potential code issues, such as unused variables, inconsistent code styles, etc.
These tools are like equipping your debugging work with microscopes and X-ray machines, allowing you to see the internal structure and running state of the code.
Summary and Reflection
Alright, today we've discussed many Python debugging techniques, from simple print statements to complex professional tools. You might ask, which method should I use?
My suggestion is: choose the appropriate tool based on the complexity of the problem. For simple problems, print statements might be enough. For more complex problems, you might need to use pdb or logging. And for large projects or performance issues, you might need to use those professional tools.
Remember, debugging is not just a technique, but more of a mindset. It requires you to maintain curiosity, constantly asking "why" until you find the root of the problem.
Finally, I want to ask you: What debugging method do you use most often? Have you encountered any particularly tricky bugs? How did you solve them? Feel free to share your experiences in the comments!
Debugging can sometimes be frustrating, but don't forget, every time you solve a bug, you're one step closer to becoming a better programmer. So, next time you encounter a bug, don't be discouraged, treat it as an opportunity to learn. Keep going, I believe you can definitely become a debugging expert!
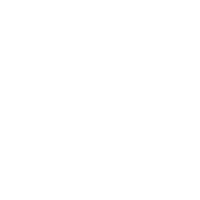
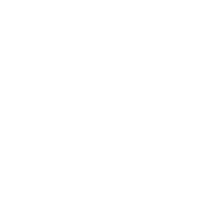