Introduction
Have you ever been frustrated by a stubborn bug late at night? Ever felt like finding a needle in a haystack while debugging? Today, I want to share my years of Python debugging experience and insights with you. As a Python developer who frequently deals with bugs, I deeply understand the ups and downs of the debugging process. Let's explore the mysteries of Python debugging together and master the techniques that make debugging twice as effective.
Understanding
Before diving into the details, I want to talk about the mindset of debugging. Many people view debugging as a painful task, but I think it's actually a great learning opportunity. Each debugging session is a process of deepening our understanding of the code.
I remember when I first started learning Python, I was always afraid of encountering errors. Looking back now, it was those headache-inducing bugs that helped me build systematic debugging thinking. Like a detective solving a case, debugging is an interesting process of unraveling mysteries.
Basics
Let's start with the most basic debugging concepts. In Python, errors mainly fall into two categories: Syntax Errors and Runtime Errors.
Syntax errors are easy to understand - like writing "their" instead of "there" in an essay - these errors are immediately apparent to the Python interpreter. For example:
if True print("Hello") # Missing colon
This code will immediately report a syntax error because the if statement is missing a colon.
Runtime errors are trickier - they only appear when the program runs under specific conditions. The most common example is an index error:
numbers = [1, 2, 3]
print(numbers[3]) # Index out of range
You see, this code is syntactically correct, but will raise an IndexError at runtime because we're trying to access a non-existent list element.
Tools
When it comes to debugging tools, Python provides us with many useful weapons. First is the print debugging method, possibly the simplest and most commonly used approach:
def calculate_average(numbers):
print(f"Input numbers: {numbers}") # Print input
total = sum(numbers)
print(f"Sum: {total}") # Print sum
average = total / len(numbers)
print(f"Average: {average}") # Print average
return average
But print debugging isn't the most efficient method. Python's built-in pdb debugger is your real assistant. Look at this example:
def complex_calculation(x, y):
import pdb; pdb.set_trace() # Set breakpoint
result = x * y
if result > 100:
result = result / 2
return result
Using pdb, you can pause program execution, inspect variable values, and step through code. It's like putting a magnifying glass on your code, allowing you to clearly see every execution detail.
Strategy
Debugging isn't random trial and error - it needs strategy. I've summarized a "three-step" debugging method:
Step One: Reproduce the problem. Find a reliable way to reproduce the bug so you can verify if the fix works. For example:
def divide_numbers(a, b):
try:
return a / b
except ZeroDivisionError:
print("Error: Division by zero!")
return None
test_cases = [
(10, 2),
(10, 0),
(0, 5)
]
for a, b in test_cases:
result = divide_numbers(a, b)
print(f"{a} / {b} = {result}")
Step Two: Locate the problem. Use binary search to quickly narrow down the problem area. Suppose you have a long function:
def long_process():
# Set checkpoints at key points
step1_result = process_step1()
print("Step 1 completed") # Checkpoint 1
step2_result = process_step2(step1_result)
print("Step 2 completed") # Checkpoint 2
final_result = process_step3(step2_result)
print("Step 3 completed") # Checkpoint 3
return final_result
Step Three: Verify the fix. Don't rush to celebrate - write test cases to verify if the fix works:
def test_fix():
# Prepare test data
test_inputs = [
{"name": "test1", "data": [1, 2, 3]},
{"name": "test2", "data": []},
{"name": "test3", "data": [0]}
]
for test in test_inputs:
try:
result = your_fixed_function(test["data"])
print(f"Test {test['name']} passed: {result}")
except Exception as e:
print(f"Test {test['name']} failed: {str(e)}")
Advanced
Once you've mastered basic debugging techniques, it's time to learn some advanced tricks.
Logging is a very important debugging tool. Compared to print, the logging module offers more flexibility:
import logging
logging.basicConfig(
level=logging.DEBUG,
format='%(asctime)s - %(levelname)s - %(message)s',
filename='debug.log'
)
def complex_operation(data):
logging.info(f"Starting operation with data: {data}")
try:
result = process_data(data)
logging.debug(f"Intermediate result: {result}")
final_result = post_process(result)
logging.info(f"Operation completed successfully")
return final_result
except Exception as e:
logging.error(f"Error occurred: {str(e)}")
raise
Decorators are also a powerful debugging tool. You can create a decorator for debugging:
def debug_decorator(func):
def wrapper(*args, **kwargs):
print(f"Calling {func.__name__} with args: {args}, kwargs: {kwargs}")
result = func(*args, **kwargs)
print(f"{func.__name__} returned: {result}")
return result
return wrapper
@debug_decorator
def calculate_something(x, y, multiply=False):
return x * y if multiply else x + y
Practice
Let's apply these debugging techniques through a practical example. Suppose we're developing a data processing system:
class DataProcessor:
def __init__(self):
self.logger = logging.getLogger(__name__)
self.data = []
def process_batch(self, batch):
self.logger.info(f"Processing batch of size {len(batch)}")
try:
for item in batch:
processed_item = self._process_single_item(item)
self.data.append(processed_item)
except Exception as e:
self.logger.error(f"Error processing batch: {str(e)}")
# Set breakpoint here for debugging
import pdb; pdb.set_trace()
def _process_single_item(self, item):
# Complex processing logic
if not isinstance(item, dict):
raise ValueError(f"Expected dict, got {type(item)}")
self.logger.debug(f"Processing item: {item}")
result = {
'id': item.get('id'),
'value': item.get('value', 0) * 2,
'timestamp': datetime.now()
}
return result
processor = DataProcessor()
test_batch = [
{'id': 1, 'value': 10},
{'id': 2, 'value': 20},
'invalid_item', # This will trigger an error
{'id': 3, 'value': 30}
]
processor.process_batch(test_batch)
Insights
After many years of Python development experience, I've gathered some debugging insights:
-
Stay calm. Don't panic when encountering bugs - systematic analysis is the right approach.
-
Form hypotheses. Continuously make and verify assumptions during debugging to locate problems faster.
-
Record experiences. Document every bug you solve - these experiences will become your valuable assets.
-
Use version control. Commit code frequently during debugging so you can quickly roll back when problems occur.
-
Write tests. Good test cases can help you discover problems earlier:
import unittest
class TestDataProcessor(unittest.TestCase):
def setUp(self):
self.processor = DataProcessor()
def test_valid_data(self):
test_data = {'id': 1, 'value': 10}
result = self.processor._process_single_item(test_data)
self.assertEqual(result['value'], 20)
def test_invalid_data(self):
with self.assertRaises(ValueError):
self.processor._process_single_item("invalid")
if __name__ == '__main__':
unittest.main()
Looking Forward
Debugging technology keeps evolving, with new tools and methods constantly emerging. For example, VSCode debugging plugins and various intelligent debugging assistants are now popular. But regardless of how tools advance, understanding the essence of debugging and mastering basic techniques remains most important.
What do you find most challenging about debugging? Feel free to share your experiences and concerns in the comments. Let's progress together on the path of debugging.
Remember, debugging isn't something to fear - it's an excellent opportunity to improve your programming skills. I hope this article helps you build systematic debugging thinking and makes you more confident when encountering bugs.
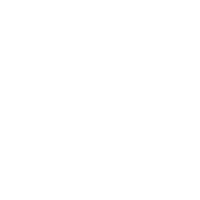