Introduction
Have you ever encountered situations where you wrote Python code that threw errors when running, but couldn't figure out what went wrong after looking at it for a long time? Or the code runs but produces unexpected results, and you can't find the reason? These are common issues we face during programming. As a Python developer, I deeply understand the importance of debugging code, and today I'd like to share my years of debugging experience and techniques with you.
Basics
Before discussing specific techniques, we need to understand the essence of debugging. Debugging isn't random trial and error, but a systematic process. Like a detective solving a case, we need to collect clues, analyze evidence, and finally find the "culprit."
Let's first look at the basic print method. Although many people think using print for debugging is low-tech, I believe it's actually one of the most direct and effective methods. Did you know that according to statistics, over 80% of simple bugs can be located using print?
Here's an example:
def calculate_average(numbers):
total = 0
for num in numbers:
print(f"Current number: {num}") # Print each number
total += num
print(f"Total: {total}") # Print sum
average = total / len(numbers)
print(f"Average: {average}") # Print average
return average
numbers = [1, 2, 3, 4, 5]
result = calculate_average(numbers)
By printing intermediate values, we can clearly see the program's execution flow and quickly identify potential issues.
Tools
When it comes to debugging tools, Python's built-in pdb is absolutely essential to master. It's like installing a "microscope" for your code, allowing you to observe the code execution process line by line.
Let me teach you some of the most commonly used pdb commands:
import pdb
def complex_calculation(x, y):
pdb.set_trace() # Set breakpoint
result = x * y
for i in range(result):
temp = i ** 2
if temp > result:
result = temp
return result
value = complex_calculation(3, 4)
- n(next): Execute next line
- s(step): Step into function
- c(continue): Continue execution
- p variable: Print variable value
- l(list): Display code around current position
I remember once debugging a complex data processing function using pdb to trace step by step, finally discovering the problem was in a loop boundary condition. This made me deeply realize that sometimes "slow is fast."
Advanced
Debugging requires strategy. Like playing chess, masters always think several moves ahead. Let's look at some advanced debugging techniques:
- Binary Search Method
When facing a long function, you can use binary search to quickly locate the problem area. Here's an example:
def process_large_dataset(data):
# Insert breakpoint at middle position
midpoint = len(data) // 2
first_half = data[:midpoint]
second_half = data[midpoint:]
# Process first part
result1 = process_subset(first_half)
if not verify_result(result1):
return debug_section(first_half)
# Process second part
result2 = process_subset(second_half)
if not verify_result(result2):
return debug_section(second_half)
return combine_results(result1, result2)
- Logging Debug Method
Compared to print, logging is more professional and flexible. We can set different logging levels and save logs to files:
import logging
logging.basicConfig(
level=logging.DEBUG,
format='%(asctime)s - %(levelname)s - %(message)s',
filename='debug.log'
)
def complex_operation(data):
logging.debug(f"Start processing data: {data}")
try:
result = perform_calculation(data)
logging.info(f"Calculation result: {result}")
return result
except Exception as e:
logging.error(f"Error occurred: {str(e)}")
raise
Practical Cases
Let's look at a real debugging case. Suppose we have a function that processes user data:
class UserManager:
def __init__(self):
self.users = {}
def add_user(self, user_id, name, age):
try:
if user_id in self.users:
logging.warning(f"User ID {user_id} already exists")
return False
if not isinstance(age, int) or age < 0:
logging.error(f"Invalid age value: {age}")
return False
self.users[user_id] = {
'name': name,
'age': age,
'created_at': datetime.now()
}
logging.info(f"Successfully added user: {user_id}")
return True
except Exception as e:
logging.error(f"Error occurred while adding user: {str(e)}")
return False
In this example, we use multiple layers of defense: parameter validation, exception handling, and logging. This way, even if problems occur, we can quickly locate the cause.
Tips
At this point, I'd like to share some tips I frequently use:
- Using Assertions for Pre-checks:
def calculate_percentage(part, whole):
assert whole != 0, "Denominator cannot be zero"
assert part <= whole, "Part cannot be greater than whole"
return (part / whole) * 100
- Creating Test Data:
def generate_test_data(size=1000):
return [
{
'id': i,
'name': f'test_user_{i}',
'score': random.randint(0, 100)
}
for i in range(size)
]
- Using Decorators for Function Debugging:
def debug_decorator(func):
def wrapper(*args, **kwargs):
print(f"Calling function: {func.__name__}")
print(f"Parameters: args={args}, kwargs={kwargs}")
result = func(*args, **kwargs)
print(f"Return value: {result}")
return result
return wrapper
@debug_decorator
def complex_function(x, y, z=None):
return x + y + (z or 0)
Experience Share
In my years of Python development experience, I've found that many beginners make some common mistakes when debugging:
-
Blindly Modifying Code Some people randomly change code when encountering problems, hoping to solve them by chance. This is a very dangerous approach. The correct way is to understand the problem first, then make targeted modifications.
-
Ignoring Error Messages Python's error messages are actually very useful, telling you the error type and location. I suggest carefully reading these messages, as they often contain key clues to solving the problem.
-
Not Making Good Use of Debugging Tools Many people only use print for debugging, but Python has many powerful debugging tools. For example, VSCode's debugger can visually display variable values and call stacks.
Looking Forward
As Python evolves, debugging techniques are constantly advancing. There are now some AI-based debugging tools that can automatically analyze code and provide fix suggestions. However, I believe mastering basic debugging thinking and methods remains most important.
Debugging is not just a technique, but a way of thinking. It helps you understand code more deeply and improve your programming skills. What do you think? Feel free to share your debugging experiences in the comments.
Finally, here's a thought: debugging is like solving a case - it requires patience, attention to detail, and systematic thinking. With the right method, there's no bug that can't be solved.
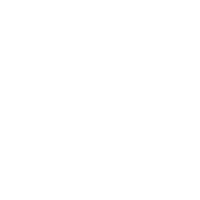
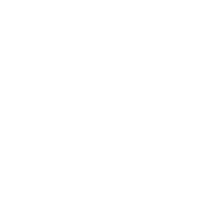