Hello, Python enthusiasts! Today, let's talk about Python debugging. As a Python developer, have you ever been tormented by a stubborn bug? Have you ever lost your way in the sea of code, not knowing where the problem lies? Don't worry, this article will take you into the fascinating world of Python debugging, transforming you from a debugging novice into a master!
Introduction
Do you remember the first time you encountered a bug? It felt like groping in the dark, not knowing where the problem was, let alone how to solve it. But don't lose heart! Every programmer has gone through this phase. Debugging skills are like a key that helps us unlock the mysterious door of the coding world.
When I first started learning Python, I was always confused when I encountered bugs. But with experience and the mastery of techniques, I gradually discovered that debugging is actually an art. It not only helps us find errors but also allows us to understand the running mechanism of the code more deeply. Today, I'll share some valuable experiences I've learned on the debugging path.
Starting with print
When it comes to debugging, the simplest and most commonly used method is to use the print()
function. Although it seems basic, don't underestimate this simple function; it is the first step on our debugging journey!
The Magic of print
The print()
function is like probes we plant in the code, allowing us to monitor variable values and execution flow in real-time. Let's look at a simple example:
def calculate_discount(price, discount_rate):
print(f"Original price: {price}") # Debug info
print(f"Discount rate: {discount_rate}") # Debug info
discounted_price = price * (1 - discount_rate)
print(f"Discounted price: {discounted_price}") # Debug info
return discounted_price
result = calculate_discount(100, 0.2)
print(f"Final result: {result}")
By inserting print()
statements at critical points, we can clearly see each step of the function's execution. This is very helpful for understanding the program's process and pinpointing issues.
Advanced Usage of print
But did you know? The print()
function actually has some little-known advanced uses. For example, we can customize the separator with the sep
parameter:
print("Debug info", "Variable value", "Timestamp", sep=" | ")
This makes our debug output clearer and more readable.
Additionally, if you don't want the debug info to affect the program's standard output, you can use the file
parameter to redirect the debug info to a separate file:
with open('debug.log', 'w') as f:
print("This is a debug message", file=f)
This way, your debug information will be written to the debug.log
file without interfering with the program's normal output.
IDE: Your Powerful Helper
After print()
, let's talk about more advanced tools—Integrated Development Environments (IDEs). If print()
is the screwdriver in our debugging toolbox, then an IDE is a Swiss Army knife: powerful and easy to use.
Choosing the Right IDE
In the Python world, there are many excellent IDEs to choose from, such as PyCharm, Visual Studio Code, and Spyder. Each has its characteristics, so choosing one that suits you is very important.
I personally prefer PyCharm because it offers powerful debugging features. However, Visual Studio Code is also popular for its lightweight nature and rich plugin ecosystem. Which IDE do you think suits you best?
Unveiling IDE Debugging Features
No matter which IDE you choose, they usually provide the following core debugging features:
- Breakpoint setting: You can set breakpoints anywhere in the code, and the program will pause automatically when it reaches here.
- Step execution: Execute code line by line and observe the result of each step.
- Variable watching: View variable values in real-time and even modify variables during debugging.
- Call stack: View the hierarchy of function calls to understand the execution flow.
Let's look at an example of debugging with PyCharm:
def factorial(n):
if n == 0:
return 1
else:
return n * factorial(n-1)
result = factorial(5)
print(f"Factorial of 5 is: {result}")
Suppose we want to understand this recursive function's execution process in detail. We can set a breakpoint at the first line of the factorial
function. Then, start debug mode, and the program will pause at the breakpoint. We can use the "step into" function to execute the code line by line and observe how the value of n
changes and how the recursion proceeds.
This way, we can clearly see each step of the recursion, which is very helpful for understanding complex algorithms. Have you ever thought about how we'd understand complex concepts like recursion without these powerful debugging tools?
pdb: Python's Secret Weapon
After IDEs, let's talk about Python's built-in debugger, pdb. Pdb is like a Swiss Army knife; it may not look impressive, but its functions are extraordinarily powerful.
Starting Your Debugging Journey with pdb
Using pdb is very simple. You only need to insert import pdb; pdb.set_trace()
in the code, and the program will pause here, entering an interactive debugging mode. Let's look at an example:
def divide(a, b):
import pdb; pdb.set_trace() # Program will pause here
return a / b
result = divide(10, 0) # This will cause an exception
print(result)
When the program runs to pdb.set_trace()
, it will pause and enter the pdb interactive environment. Here, you can input various commands to check the program's state.
pdb Advanced: Command List
Pdb provides many useful commands. Here are some common ones:
n
(next): Execute the next lines
(step): Step into a functionc
(continue): Continue execution until the next breakpointp
(print): Print the value of a variablel
(list): Show code around the current lineq
(quit): Exit the debugger
For example, in the example above, when the program pauses, you can use p a
and p b
to view variable values, use n
to execute the division operation, and then observe if an exception is triggered.
pdb Advanced Techniques: Conditional Breakpoints
Pdb also supports conditional breakpoints, which are very useful when debugging complex logic. For example:
import pdb
def process_list(items):
for i, item in enumerate(items):
if i == 5:
pdb.set_trace()
# Code to process item
process_list(range(10))
In this example, the debugger will only pause when the loop reaches the sixth element (index 5). This way, we can focus on specific situations without manually skipping uninteresting iterations.
Isn't pdb powerful? The first time I discovered these advanced features, I was amazed! Have you had similar experiences? Feel free to share your pdb usage insights in the comments!
Logs: Your Debugging Diary
After discussing real-time debugging tools, let's talk about another powerful debugging method—logging. If pdb is a scalpel that allows us to pinpoint issues precisely, logs are like medical records that document the entire process of the program's execution.
Why Do We Need Logs?
You might ask, since we already have print and debuggers, why do we need logs? Great question! Logs have unique advantages:
- Persistence: Logs can be saved for us to view anytime.
- Levels: We can set different log levels to flexibly control the amount of output information.
- Formatting: Logs can contain more information like timestamps and code locations.
- Minimal performance impact: In production environments, we can record only important logs with minimal impact on program performance.
The logging Module: Python's Logging Magic
Python's logging
module provides powerful logging features. Let's look at an example:
import logging
logging.basicConfig(level=logging.DEBUG,
format='%(asctime)s - %(name)s - %(levelname)s - %(message)s',
filename='app.log')
def calculate_average(numbers):
logging.info(f"Starting calculation of average, input data: {numbers}")
if not numbers:
logging.warning("The input list is empty!")
return 0
total = sum(numbers)
count = len(numbers)
average = total / count
logging.debug(f"Calculation result: total = {total}, count = {count}, average = {average}")
return average
result = calculate_average([1, 2, 3, 4, 5])
logging.info(f"Final result: {result}")
result = calculate_average([])
logging.info(f"Final result: {result}")
In this example, we use different log levels:
INFO
: Record normal program flow.WARNING
: Record potential problems or exceptional situations.DEBUG
: Record detailed debugging information.
Running this code, you'll see detailed log records in the app.log
file, including inputs, outputs, and possible warnings. This is very helpful for understanding the program's process and troubleshooting issues.
Best Practices for Logging
In actual projects, I have some small tips for using logs:
- Use different log levels: Use DEBUG during development and INFO or WARNING in production.
- Record key information: Include function inputs and outputs, important intermediate results, and exception information.
- Use structured logging: If possible, use JSON format for logs for easier subsequent analysis.
- Archive regularly: Set up log rotation to avoid overly large log files.
Do you have any insights on using logs? Feel free to share in the comments!
Exception Handling: The Art of Turning Danger into Safety
When it comes to debugging, we must mention exception handling. Exceptions are like "accidents" in the programming world, and excellent exception handling is our "insurance."
Why Handle Exceptions?
You might ask, since we have so many debugging tools, why do we need exception handling? Great question! Exception handling has several important roles:
- Prevent program crashes: Catching and handling exceptions allows the program to continue running.
- Provide useful information: Exceptions can tell us what went wrong and where.
- Handle errors gracefully: We can provide user-friendly error messages instead of cold stack traces.
try-except: The Basic Weapon of Exception Handling
Python's try-except
statement is the basic tool for handling exceptions. Let's look at an example:
def safe_divide(a, b):
try:
result = a / b
except ZeroDivisionError:
print("Error: Division by zero!")
return None
except TypeError:
print("Error: Inputs must be numbers!")
return None
else:
print("Division successful.")
return result
finally:
print("Division operation ended.")
print(safe_divide(10, 2)) # Normal case
print(safe_divide(10, 0)) # Zero division error
print(safe_divide(10, '2')) # Type error
In this example, we handle two possible exceptions: zero division error and type error. The else
clause executes when no exceptions occur, and the finally
clause executes regardless of whether an exception occurs.
Custom Exceptions: Craft Your Exception Handling Tool
In addition to using Python's built-in exceptions, we can also create custom exceptions. This is especially useful for handling errors specific to your application. For example:
class ValueTooSmallError(Exception):
"""Exception raised when a value is below a threshold"""
pass
class ValueTooLargeError(Exception):
"""Exception raised when a value is above a threshold"""
pass
def validate_age(age):
if age < 0:
raise ValueTooSmallError("Age cannot be negative!")
elif age > 150:
raise ValueTooLargeError("Age seems too large!")
else:
print(f"Age {age} is valid.")
try:
validate_age(200)
except ValueTooSmallError as e:
print(e)
except ValueTooLargeError as e:
print(e)
By creating custom exceptions, we can more precisely describe and handle specific error situations.
Best Practices for Exception Handling
In actual projects, I've summarized some small tips for exception handling:
- Catch only exceptions you know how to handle.
- Use specific exception types whenever possible instead of general
Exception
. - Provide useful context in exception messages.
- Consider using the
logging
module to record exceptions instead of simply printing them. - Re-raise exceptions in appropriate places to give higher-level code a chance to handle them.
Exception handling is an art that requires continuous practice and reflection. Do you have any unique methods for handling exceptions? Feel free to share your experiences in the comments!
The Art of Debugging: Beyond Tools
After discussing so many specific debugging techniques, I want to talk about the "art" level of debugging in the end. Because a true debugging master is not just proficient in using various tools but more importantly, cultivates a debugging mindset.
Cultivating Debugging Intuition
Have you ever encountered a situation where just by looking at a piece of code, your intuition tells you "there might be an issue here"? That's debugging intuition. It comes from experience, but it's not just a simple accumulation of experience. Here are a few small tips for cultivating debugging intuition:
- Think more, guess less: When encountering problems, stop and think about possible causes instead of rushing to try solutions.
- Focus on edge cases: Pay special attention to boundary conditions and special inputs in the code.
- Learn to ask questions: Ask yourself "What if...?" which helps uncover potential issues.
Systematic Debugging Methods
While intuition is important, systematic debugging methods are equally indispensable. One method I often use is the "binary method":
- Determine the scope of the problem.
- Divide the scope into two halves.
- Check which half contains the problem.
- Repeat steps 2 and 3 until the specific problem point is located.
This method is especially useful for dealing with large codebases or complex logic issues.
Prevention is Better than Cure
Finally, I'd like to say that the best debugging is not needing to debug at all. How can we reduce the occurrence of bugs? Here are some suggestions:
- Write clear and concise code.
- Use type hints and static type checking tools like mypy.
- Write unit tests that cover various possible cases.
- Conduct code reviews to have others help you discover potential problems.
Remember, every bug is a learning opportunity. When you've solved a tricky issue, don't just celebrate, but think about how to prevent similar problems from happening again.
Conclusion
Wow, our Python debugging journey is coming to an end. From the simple print()
to complex debuggers, from basic exception handling to advanced logging techniques, we've mastered a powerful set of debugging tools. But remember, tools are always just tools; a true debugging master relies on sharp insight and systematic thinking.
Debugging is a skill that requires continuous practice. Every time you encounter a bug, it's an opportunity to improve yourself. So, don't fear bugs; embrace them and learn from them.
Finally, I'd like to ask you: What is your favorite debugging technique? Do you have any unique debugging experiences you want to share? Feel free to leave a comment, and let's discuss and grow together!
Remember, in the world of programming, we are all lifelong learners. Stay curious, keep the passion for learning, and you'll find that debugging is no longer a chore but a fun exploration journey.
Happy coding and successful debugging in the world of Python!
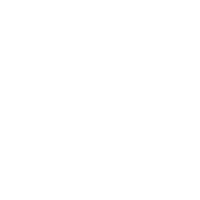
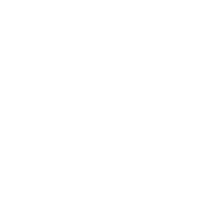