Hello, dear Python enthusiasts! Today, we'll dive deep into a crucial yet often overlooked topic - Python's memory management. As a Python developer, understanding memory management mechanisms not only helps you write more efficient code but also makes it easier to find solutions when encountering memory-related issues. So, let's begin this journey of exploring memory management!
Object Lifecycle
In Python, everything is an object. From simple integers to complex class instances, all data are objects. Have you ever wondered how these objects are created, used, and destroyed in memory?
Let's start with a simple example:
def create_list():
my_list = [1, 2, 3]
print(f"List created: {my_list}")
create_list()
print("Function finished")
In this example, we created a list object in the create_list
function. What happens to this list object when the function completes?
In fact, Python uses reference counting to track objects. Whenever an object is referenced (like being assigned to a variable), its reference count increases. When the reference count drops to zero, the object is destroyed and memory is reclaimed.
In our example, when the create_list
function finishes executing, the my_list
variable goes out of scope, the list object's reference count drops to zero, so it gets automatically recycled.
However, reference counting isn't perfect. Do you know about the circular reference problem? Look at this example:
def create_cycle():
list1 = []
list2 = []
list1.append(list2)
list2.append(list1)
create_cycle()
In this example, list1
and list2
reference each other, so their reference counts won't drop to zero even when they go out of scope. This is called circular reference, which can lead to memory leaks.
To solve this problem, Python also uses a garbage collector. The garbage collector periodically checks and cleans up these circularly referenced objects. You can control garbage collection through the gc
module:
import gc
gc.collect()
gc.disable()
gc.enable()
Memory Allocation Strategy
You might wonder, how does Python allocate memory for these objects? Here's an interesting fact: Python uses a technique called "memory pool" to improve allocation efficiency for small objects.
For small integers (-5 to 256) and short strings, Python pre-allocates some objects and keeps them in a memory pool. This way, when you create these objects, Python can fetch directly from the memory pool instead of allocating memory each time.
Here's an example:
a = 5
b = 5
print(a is b) # Output: True
c = 1000
d = 1000
print(c is d) # Output: False
In this example, a
and b
actually point to the same object because 5 is fetched from the memory pool. However, c
and d
are different objects because 1000 is outside the memory pool range.
This mechanism greatly improves Python's performance, especially when handling many small objects. However, it can also lead to some confusing behaviors, like the is
comparison results in the above example.
Memory Optimization Tips
Understanding Python's memory management mechanisms allows us to optimize our code. Here are some practical tips:
- Use generators instead of lists:
numbers = [x for x in range(1000000)]
numbers = (x for x in range(1000000))
Generators only generate data when needed, thus greatly reducing memory usage.
- Release objects that are no longer needed:
import gc
def process_large_data(data):
# Process data
result = do_something_with(data)
# Manually delete large objects to free memory
del data
gc.collect()
return result
- Use
__slots__
:
class Point:
__slots__ = ['x', 'y']
def __init__(self, x, y):
self.x = x
self.y = y
__slots__
can significantly reduce instance object memory usage, especially when creating many objects.
- Use the
array
module instead of lists for storing numbers:
from array import array
numbers = [1, 2, 3, 4, 5]
numbers = array('i', [1, 2, 3, 4, 5])
array
is more memory efficient than regular lists, especially when storing large amounts of numbers.
Memory Leak Detection
Even when we're careful, memory leaks can still occur. So, how do we detect memory leaks? Here are some useful tools:
memory_profiler
:
from memory_profiler import profile
@profile
def my_func():
a = [1] * (10 ** 6)
b = [2] * (2 * 10 ** 7)
del b
return a
if __name__ == '__main__':
my_func()
Running this code will show you detailed memory usage reports.
objgraph
:
import objgraph
objgraph.show_most_common_types()
objgraph.show_backrefs([obj], filename='backrefs.png')
objgraph
can help you visualize object graphs and find the source of memory leaks.
tracemalloc
:
import tracemalloc
tracemalloc.start()
snapshot = tracemalloc.take_snapshot()
top_stats = snapshot.statistics('lineno')
print("[ Top 10 ]")
for stat in top_stats[:10]:
print(stat)
tracemalloc
is a built-in module introduced in Python 3.4 that helps you track memory allocations.
Summary
Well, today we've deeply explored Python's memory management mechanisms, from object lifecycle to memory allocation strategies, and from memory optimization to leak detection. Do you now have a deeper understanding of Python's internal workings?
Remember, understanding memory management isn't just for answering interview questions. In real development, especially when dealing with big data or long-running programs, this knowledge can help you write more efficient and stable code.
Do you have any experiences or questions about Python memory management? Feel free to share your thoughts in the comments. Let's discuss and grow together!
Next time, we'll talk about Python's concurrent programming, stay tuned!
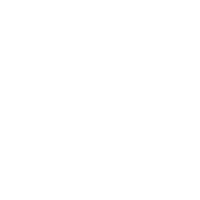
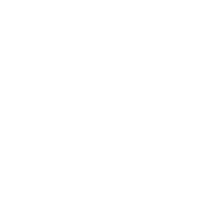