Hello, dear Python programmer friends! Today we're going to discuss a very important but often overlooked topic - Python debugging. As a Python blogger, I must say that mastering debugging techniques is crucial for improving programming efficiency and code quality. So, let's dive into the mysteries of Python debugging together!
Error Types
Before learning debugging techniques, let's first understand the common error types in Python. I'm sure you've all encountered these "old friends" during the programming process, right?
SyntaxError
This is probably the most common type of error. When your code has syntax issues, the Python interpreter will throw a SyntaxError. For example:
if x = 5: # Should be ==, not =
print("x is 5")
Here, the assignment operator =
is used instead of the comparison operator ==
. Python will point out the exact location of the error, which is very helpful!
NameError
You'll encounter a NameError when you try to use an undefined variable. For example:
print(undefined_variable) # 'undefined_variable' is not defined
This error is usually caused by a spelling mistake or forgetting to initialize a variable. In my experience, sometimes this error can make you think, "That's impossible, I clearly defined this variable." In such cases, it's worth double-checking the spelling of the variable name or whether the variable is in the correct scope.
TypeError
A TypeError will be raised when you perform an operation on an inappropriate type. For example:
result = "5" + 5 # Cannot add a string and an integer
This error reminds us to pay attention to data type conversions. It's especially common when handling user input or data from different sources.
IndexError
You'll encounter an IndexError when you try to access an index that's out of the sequence's range. For example:
my_list = [1, 2, 3]
print(my_list[3]) # Index 3 is out of the list's range
This error often occurs when dealing with lists, tuples, or strings. My advice is to check the length of the sequence before using an index.
ValueError
A ValueError will be raised when a function receives an inappropriate value. For example:
int("abc") # Cannot convert the string "abc" to an integer
This error reminds us to pay attention to the validity and legality of data. When handling user input or external data, it's best to validate the data first.
AttributeError
You'll encounter an AttributeError when you try to access an attribute that doesn't exist on an object. For example:
"hello".append("world") # String objects don't have an append method
This error is usually due to a misunderstanding of the object's type or available methods. My advice is to use the dir()
function to check an unfamiliar object's attributes and methods before using it.
KeyError
A KeyError will be raised when you try to access a key that doesn't exist in a dictionary. For example:
my_dict = {"a": 1, "b": 2}
print(my_dict["c"]) # Key "c" doesn't exist
This error reminds us to be careful when using dictionaries. If you're not sure whether a key exists, you can use the get()
method or the in
operator to check.
ImportError
You'll encounter an ImportError when Python can't find the module you're trying to import. For example:
import non_existent_module
This error could be caused by a misspelled module name, a missing installation, or a Python environment configuration issue. When encountering this error, first check if the module name is correct, then confirm if the module is installed.
Understanding these common error types can help us locate problems more quickly. However, knowing the error types is not enough; we also need to master some debugging techniques to solve problems more efficiently. Next, let's explore some practical debugging techniques!
Importance of Debugging
Before delving into specific debugging techniques, I want to discuss why debugging is so important. You might ask, "As long as my code runs, why bother spending time on debugging?"
Let me give you an example. Suppose you're developing an online shopping system. One day, you receive feedback from users that they're unable to complete their orders. You check the logs and find that it's due to a division by zero error when calculating the total price. This bug could lead to a significant loss of orders and revenue for the company. If you had thoroughly debugged during the development stage, this issue could have been discovered and fixed early on.
The importance of debugging lies in the following aspects:
-
Improving Code Quality: Through debugging, we can find and fix potential bugs, making our code more robust and reliable.
-
Saving Time: Although debugging may seem time-consuming, it can help us discover and solve problems early before they become more complex, ultimately saving time in the long run.
-
Deepening Code Understanding: The debugging process helps us better understand the code's execution flow and internal logic, which is very helpful for improving programming skills.
-
Enhancing User Experience: By promptly finding and fixing bugs, we can provide users with more stable and reliable software products.
-
Reducing Maintenance Costs: Good debugging habits can help us write clearer, more maintainable code, thereby reducing future maintenance costs.
I remember once, while developing a data analysis project, I encountered a very peculiar bug. The program would occasionally crash when processing large amounts of data, but the frequency was low, making it difficult to reproduce. I spent two whole days debugging, and eventually discovered it was due to a small memory leak issue. Although the debugging process was painful, the sense of accomplishment when I finally solved the problem was unmatched. Moreover, through this experience, I learned a lot about memory management and performance optimization.
So, my advice is: Don't view debugging as a burden, but rather as an opportunity to improve your programming skills. Now that we know the importance of debugging, let's learn some practical debugging techniques together!
Print Debugging
When it comes to debugging techniques, the simplest and most commonly used method is undoubtedly using print()
statements. Although this method may seem basic, when used properly, the effects can be quite astonishing!
Strategically Placing Print Statements
The key to using print()
statements for debugging is to strategically place these statements. We need to insert print()
statements at critical points in the code to trace the program's execution flow and variable changes.
Let's look at an example:
def calculate_discount(price, discount_rate):
print(f"Entering calculate_discount with price={price}, discount_rate={discount_rate}")
if discount_rate < 0 or discount_rate > 1:
print(f"Invalid discount_rate: {discount_rate}")
return None
discounted_price = price * (1 - discount_rate)
print(f"Calculated discounted_price: {discounted_price}")
return discounted_price
result = calculate_discount(100, 0.2)
print(f"Final result: {result}")
In this example, we've inserted print()
statements at the beginning of the function, after the condition check, and after calculating the result. This way, we can clearly see the function's execution flow and the values of each variable.
Inspecting Variable Values and Program Flow
Using print()
statements not only allows us to inspect variable values but also helps us understand the program's execution flow. For example:
def process_data(data):
print("Starting data processing...")
for i, item in enumerate(data):
print(f"Processing item {i}: {item}")
# Complex processing logic
result = item * 2
print(f"Processed result: {result}")
print("Data processing completed.")
data = [1, 2, 3, 4, 5]
process_data(data)
Through these print()
statements, we can clearly see the program's execution order and the result of each step.
Using print()
statements for debugging has the following advantages:
- Simple and Direct: No additional tools or setup required.
- Flexible: You can print any information you want to see.
- Easy to Understand: The output information is straightforward.
However, this method also has some limitations:
- It may produce a large amount of output, making it difficult to find critical information.
- You need to manually add and remove
print()
statements, which may affect the code's cleanliness. - It may not be suitable for complex program flows or multi-threaded programs.
Nevertheless, for simple debugging tasks, print()
statements are still a very useful tool. I personally use this method frequently in my daily programming to quickly locate issues.
What do you think about using print()
statements for debugging? Have you encountered any problems that this method couldn't solve? Feel free to share your experiences in the comments!
Interactive Debugger
After discussing print()
debugging, let's talk about a more advanced debugging technique - using an interactive debugger. Python's built-in pdb
(Python Debugger) is a powerful interactive debugging tool. It allows us to pause program execution, step through code line by line, inspect variable values, and even execute arbitrary Python code in the program's context.
pdb Basics
The simplest way to use pdb
is to insert the breakpoint()
function (Python 3.7+) or import pdb; pdb.set_trace()
in your code. When the program execution reaches this line, it will pause and enter interactive debugging mode.
Let's look at an example:
def divide_numbers(a, b):
breakpoint() # The program will pause here
result = a / b
return result
print(divide_numbers(10, 0))
When the program execution reaches breakpoint()
, it will pause and enter the pdb debugger. At this point, you can enter various commands to inspect and control the program's execution.
Common pdb Commands
In the pdb debugger, there are many useful commands. Here are some of the most common ones:
n
(next): Execute the current line and move to the next line.s
(step): Step into a function call.c
(continue): Continue execution until the next breakpoint or the program ends.p
(print): Print the value of an expression.l
(list): Display the code around the current line.q
(quit): Exit the debugger.
Let's see how to use these commands through an actual example:
def calculate_average(numbers):
total = sum(numbers)
breakpoint()
average = total / len(numbers)
return average
numbers = [1, 2, 3, 4, 5]
result = calculate_average(numbers)
print(f"The average is: {result}")
When the program execution reaches breakpoint()
, it will enter the pdb debugger. At this point, you can:
- Enter
p total
to inspect the value oftotal
. - Enter
n
to executeaverage = total / len(numbers)
. - Enter
p average
again to inspect the calculated average value. - Enter
c
to continue executing the program until it finishes.
The benefits of using the pdb debugger are:
- You can pause execution at any point in the program.
- You can step through the code line by line, gaining a deeper understanding of the program's execution process.
- You can inspect and modify variable values at any time.
- You can execute arbitrary Python code in the program's context.
However, pdb also has some limitations:
- For beginners, it may take some time to become familiar with the various commands.
- When dealing with large programs, it may feel a bit cumbersome.
- It's not as intuitive as a graphical debugger.
Nevertheless, I strongly recommend that every Python programmer learn how to use pdb. It's a powerful tool that can help you deeply understand the program's execution process and quickly locate and solve problems.
I remember once, while working on a complex data processing task, I encountered a tricky bug. Using print()
statements couldn't effectively locate the issue because the data structures involved were too complex. In the end, I used pdb to step through the code line by line, carefully observing the result of each step, and finally found the root cause of the problem. This experience made me deeply appreciate the power of an interactive debugger.
Have you used pdb before? Do you find it useful? Feel free to share your experiences and opinions in the comments!
IDE Integrated Debugging
When it comes to debugging tools, we can't overlook the debugging features integrated into Integrated Development Environments (IDEs). Modern IDEs like PyCharm and VS Code provide powerful graphical debugging tools that combine the functionality of pdb with an intuitive user interface, making the debugging process simpler and more efficient.
PyCharm Debugging Features
PyCharm is a very popular Python IDE, and it provides powerful debugging features. Here are some of the main features of the PyCharm debugger:
-
Breakpoint Setting: You can set or remove breakpoints by simply clicking on the blank space next to the line numbers in the code.
-
Step Control: You can control the program's execution using toolbar buttons or shortcuts, including step into, step over, and step out operations.
-
Variable Inspection: During the debugging process, you can inspect the values of all local and global variables in real-time.
-
Expression Evaluation: You can enter any Python expression in the "Evaluate Expression" window and see the result.
-
Call Stack: You can view the current function call stack and switch between different stack frames.
-
Conditional Breakpoints: You can set breakpoints that only trigger when specific conditions are met.
Let's look at an example of using PyCharm for debugging:
def factorial(n):
if n == 0 or n == 1:
return 1
else:
return n * factorial(n-1)
result = factorial(5)
print(f"The factorial of 5 is: {result}")
In PyCharm, you can set a breakpoint on the line return n * factorial(n-1)
. Then, run the program in debug mode. When the program execution reaches the breakpoint, it will pause, and you can:
- Inspect the current value of the local variable
n
. - Use the "Step Into" button to step into the recursive call.
- Use the "Evaluate Expression" window to calculate the value of
factorial(n-1)
. - Use the "Step Over" button to execute the current line and then inspect the return value.
VS Code Debugging Features
Although VS Code is a lightweight editor, its debugging features are also quite powerful. Here are some of the main features of the VS Code debugger:
-
Multi-language Support: VS Code supports debugging for multiple programming languages, including Python.
-
Breakpoint Management: You can easily set, disable, and remove breakpoints.
-
Variable Inspection: You can inspect the values of all variables in the VARIABLES panel.
-
Watch Expressions: You can add expressions to the WATCH panel to monitor their value changes in real-time.
-
Call Stack: You can view and manage the call stack in the CALL STACK panel.
-
Breakpoint Conditions: You can set conditions or hit counts for breakpoints.
Using VS Code to debug the factorial function example is similar to the process in PyCharm. You'll need to configure a Python debugging environment first, and then you can set breakpoints and use the debug console to control the program's execution.
Using an IDE for debugging has the following advantages:
- The graphical interface is intuitive and user-friendly, suitable for beginners.
- It provides a wealth of features, such as conditional breakpoints and expression evaluation.
- You can conveniently inspect variable values and the call stack.
- It's integrated with the code editor, improving development efficiency.
However, IDE debugging also has some limitations:
- For simple debugging tasks, it may seem "heavy."
- It may not be usable in certain environments (e.g., remote servers).
- It may lead to over-reliance on the graphical interface, overlooking the importance of command-line debugging tools.
Personally, I really enjoy using PyCharm for debugging, especially when dealing with complex projects. However, I also frequently use pdb because it's lightweight and can be used in any environment.
Which debugging tool do you prefer? The graphical IDE debugger or the command-line pdb? Or do you have other recommended debugging tools? Feel free to share your thoughts in the comments!
Logging
When it comes to debugging, we can't overlook a very important but often overlooked technique - logging. Logging not only helps us debug programs but also allows us to monitor the program's runtime status and analyze its behavior patterns. Python's logging
module provides powerful and flexible logging capabilities, so let's explore it together!
Using the logging Module
Python's logging
module is part of the standard library, so no additional installation is required. Using it is very simple; here's a basic example:
import logging
logging.basicConfig(level=logging.DEBUG, format='%(asctime)s - %(levelname)s - %(message)s')
def divide(x, y):
logging.info(f"Dividing {x} by {y}")
try:
result = x / y
logging.debug(f"Result is {result}")
return result
except ZeroDivisionError:
logging.error("Division by zero!")
return None
print(divide(10, 2))
print(divide(10, 0))
In this example, we:
- Use
basicConfig()
to configure the basic logging settings, including the log level and output format. - Use different logging functions (
info()
,debug()
,error()
) at different levels to log various information within the function.
Running this code, you'll see output similar to this:
2023-06-10 15:30:45,123 - INFO - Dividing 10 by 2
2023-06-10 15:30:45,124 - DEBUG - Result is 5.0
5.0
2023-06-10 15:30:45,125 - INFO - Dividing 10 by 0
2023-06-10 15:30:45,126 - ERROR - Division by zero!
None
Configuring Log Levels and Output
The logging
module provides multiple log levels, from lowest to highest:
- DEBUG: Detailed debug information
- INFO: Confirming that the program is running as expected
- WARNING: Indicating potential issues
- ERROR: Due to more severe problems, the program was unable to perform some functions
- CRITICAL: Severe errors, indicating that the program may not be able to continue running
You can set an appropriate log level based on your needs. For example, in a development environment, you may want to see all DEBUG information; whereas in a production environment, you may only want to see WARNING and higher-level information.
In addition to console output, you can also write logs to a file:
import logging
logging.basicConfig(filename='app.log', level=logging.DEBUG,
format='%(asctime)s - %(levelname)s - %(message)s')
This way, all logs will be written to the 'app.log' file.
Using logging for debugging has the following advantages:
- It can persistently log the program's runtime status, helping to discover and analyze intermittent issues.
- You can control the level of detail in the output (by modifying the log level) without modifying the code.
- You can output to multiple destinations simultaneously (e.g., console, file, network, etc.).
- It's safe to use in multi-threaded environments.
However, there are also some considerations when using logging:
- Excessive logging may impact program performance, especially in I/O-intensive scenarios.
- You need to plan a reasonable logging strategy, including log levels, rotation policies, etc.
- Sensitive information (such as passwords) should not be logged.
Personally, I often use logging in development, especially when developing server-side programs. Once, while developing a web service, I encountered strange errors in the production environment that couldn't be reproduced in the development environment. By carefully analyzing the production logs, I eventually discovered the root cause - it was due to certain special inputs. Without detailed logging, this issue might have plagued me for a long time.
Have you used logging in your projects? Do you have any insights or experiences to share? Or have you encountered any tricky problems that logging helped you solve? Feel free to discuss in the comments!
Testing Frameworks
When it comes to debugging, we can't overlook an important step - testing. Good testing practices can help us discover and fix bugs early, improving code quality. Python has two main testing frameworks: unittest and pytest. Today, let's explore how to use these two frameworks for testing.
Introduction to unittest
unittest is Python's standard library testing framework, inspired by JUnit. Writing tests with unittest is very intuitive; let's look at a simple example:
import unittest
def add(a, b):
return a + b
class TestAddFunction(unittest.TestCase):
def test_add_positive_numbers(self):
self.assertEqual(add(1, 2), 3)
def test_add_negative_numbers(self):
self.assertEqual(add(-1, -1), -2)
def test_add_zero(self):
self.assertEqual(add(5, 0), 5)
if __name__ == '__main__':
unittest.main()
In this example, we:
- Define a simple
add
function. - Create a test class that inherits from
unittest.TestCase
. - Define several test methods within the test class, each testing a different aspect of the
add
function. - Use the
self.assertEqual
method to assert the expected results.
Running this script, you'll see the test results:
...
----------------------------------------------------------------------
Ran 3 tests in 0.001s
OK
Introduction to pytest
pytest is a more modern and powerful Python testing framework. Using it is even simpler; you don't need to create test classes, just write test functions directly. Let's rewrite the above example using pytest:
def add(a, b):
return a + b
def test_add_positive_numbers():
assert add(1, 2) == 3
def test_add_negative_numbers():
assert add(-1, -1) == -2
def test_add_zero():
assert add(5, 0) == 5
Notice that we don't need to import any modules or create test classes. We only need to write functions starting with test_
and use assert
statements to check the results.
To run these tests, you'll need to install pytest first (pip install pytest
), then run the pytest
command in the command line.
pytest also provides many powerful features, such as test parameterization and fixtures. For example, we can write a parameterized test like this:
import pytest
def add(a, b):
return a + b
@pytest.mark.parametrize("a,b,expected", [
(1, 2, 3),
(-1, -1, -2),
(5, 0, 5),
])
def test_add(a, b, expected):
assert add(a, b) == expected
This way, we cover multiple cases with a single test function.
Using testing frameworks for debugging has the following advantages:
- It can automate the testing process, improving efficiency.
- It can help discover issues early, preventing bugs from entering the production environment.
- Tests can serve as documentation, helping to understand the expected behavior of the code.
- It facilitates refactoring, as you can immediately know if modifications break existing functionality.
However, writing and maintaining tests also comes with a cost:
- It requires additional time to write test code.
- The tests themselves may contain bugs.
- Over-reliance on tests may inhibit code flexibility.
Personally, I really enjoy using pytest for testing. I remember once, while refactoring a complex data processing module, it was precisely because of the comprehensive test suite that I was able to confidently make large-scale modifications. Running the tests after each modification immediately revealed whether existing functionality was broken. This greatly improved my development efficiency while ensuring code quality.
Have you used unittest or pytest in your projects? Which one do you prefer? Or do you have another favorite testing framework? Feel free to share your experiences and opinions in the comments!
Performance Analysis Tools
When it comes to Python debugging, we can't overlook the important topic of performance analysis. Sometimes, our programs may run correctly but be very slow. In such cases, we need to use performance analysis tools to identify bottlenecks in our programs. Python's standard library provides a powerful performance analysis tool - cProfile. Let's explore how to use it together!
Using cProfile
cProfile is Python's built-in performance profiler; it can provide detailed function call statistics, including call counts, cumulative times, etc. Using cProfile is very simple; let's look at an example:
import cProfile
def fibonacci(n):
if n < 2:
return n
return fibonacci(n-1) + fibonacci(n-2)
def main():
result = fibonacci(30)
print(f"The 30th Fibonacci number is {result}")
cProfile.run('main()')
When you run this script, cProfile will gather performance data and print a report showing the time spent in each function, like this:
4 function calls in 0.000 seconds
Ordered by: standard name
ncalls tottime percall cumtime percall filename:lineno(function)
1 0.000 0.000 0.000 0.000 <string>:1(<module>)
1 0.000 0.000 0.000 0.000 {built-in method builtins.exec}
1 0.000 0.000 0.000 0.000 {method 'disable' of '_lsprof.Profiler' objects}
1 0.000 0.000 0.000 0.000 profiling.py:15(fibonacci)
This report shows that the fibonacci
function was called once and took 0 seconds to complete. While this example is trivial, cProfile can provide valuable insights for more complex programs.
Understanding cProfile Output
Let's look at a more detailed example to better understand the cProfile output:
import cProfile
def factorial(n):
if n == 0:
return 1
else:
return n * factorial(n-1)
def main():
result = factorial(10)
print(f"The factorial of 10 is {result}")
cProfile.run('main()')
Running this script will produce output similar to the following:
20 function calls in 0.000 seconds
Ordered by: standard name
ncalls tottime percall cumtime percall filename:lineno(function)
1 0.000 0.000 0.000 0.000 <string>:1(<module>)
1 0.000 0.000 0.000 0.000 {built-in method builtins.exec}
1 0.000 0.000 0.000 0.000 {method 'disable' of '_lsprof.Profiler' objects}
1 0.000 0.000 0.000 0.000 profiling.py:9(main)
11 0.000 0.000 0.000 0.000 profiling.py:5(factorial)
5 0.000 0.000 0.000 0.000 {built-in method builtins.len}
Here's what each column in the report means:
ncalls
: The number of times the function was called.tottime
: The total time spent in the function (excluding time spent in sub-functions).percall
: The quotient oftottime
divided byncalls
.cumtime
: The total time spent in the function (including time spent in sub-functions).percall
: The quotient ofcumtime
divided byncalls
.filename:lineno(function)
: The name of the function and its location in the source code.
Using cProfile for performance analysis has the following advantages:
- It provides detailed function-level profiling data, making it easier to identify bottlenecks.
- It's built into the Python standard library, so no additional installation is required.
- It can be used to profile both simple scripts and complex applications.
However, there are also some limitations:
- The profiling process itself can introduce overhead, potentially affecting the accuracy of the results.
- The output can be overwhelming for large and complex programs, making it difficult to identify the root cause of performance issues.
- It doesn't provide insights into other performance factors, such as memory usage or I/O operations.
Despite these limitations, cProfile is a valuable tool for performance analysis and optimization. By identifying and addressing performance bottlenecks, we can significantly improve the efficiency of our Python programs.
I remember once, while working on a computationally intensive project, I noticed that the program was running slower than expected. Using cProfile, I was able to identify the most time-consuming functions and optimize them, resulting in a significant performance improvement.
Have you used cProfile or other performance analysis tools in your Python projects? What has been your experience? Feel free to share your thoughts and insights in the comments!
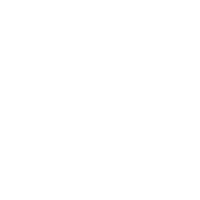
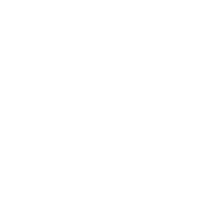