Introduction
Are you often troubled by bugs in your code? Do you frequently find yourself scratching your head at error messages on the screen late at night? Don't worry, as a Python enthusiast, I can relate. Today, let's explore the mysteries of Python debugging together, and make those pesky bugs have nowhere to hide!
Basics
The Print Method
When it comes to debugging, many people's first reaction is to use print statements. Indeed, this is the simplest and most direct method. But did you know? We can actually make it more sophisticated!
Take a look at this example:
def debug_print(*args, **kwargs):
import sys
print(*args, file=sys.stderr, **kwargs)
debug_print("The value of variable x is:", x)
What are the benefits of this little trick? First, we can easily distinguish between normal output and debug output. Second, we can control the display of debug information by redirecting stderr. Isn't that clever?
However, for complex data structures, ordinary print might make your head spin. This is where the pprint module comes in handy:
from pprint import pprint
complex_data = {'name': 'Alice', 'age': 30, 'friends': ['Bob', 'Charlie', 'David'], 'info': {'city': 'New York', 'job': 'Engineer'}}
pprint(complex_data)
See, isn't it much clearer? pprint automatically formats the output, making complex data structures easy to read.
Breakpoint Magic
Print is good, but it doesn't feel elegant enough? Then let's get to know Python's debugging tool - the pdb module!
import pdb
def complex_function(x, y):
result = x * y
pdb.set_trace() # Set breakpoint
return result * 2
complex_function(3, 4)
When you run this code, the program will pause at pdb.set_trace() and enter interactive debugging mode. You can enter various commands to inspect variables and step through the code. Don't you feel like a code detective suddenly?
However, if you feel pdb is not powerful enough, try ipdb! It's an enhanced version of pdb, supporting syntax highlighting, auto-completion, and other features. It's also easy to use:
import ipdb
def complex_function(x, y):
result = x * y
ipdb.set_trace() # Set breakpoint
return result * 2
complex_function(3, 4)
Advanced
Logging
As your program becomes more complex, relying solely on print and breakpoints may not be enough. At this point, we need a more systematic method to record the program's running state - logging.
Python's logging module is born for this:
import logging
logging.basicConfig(level=logging.DEBUG, format='%(asctime)s - %(levelname)s - %(message)s')
def complex_calculation(x, y):
logging.debug(f"Starting calculation, parameters: x={x}, y={y}")
result = x * y
logging.info(f"Calculation result: {result}")
return result
complex_calculation(3, 4)
Through this method, we can record the program's running process in detail, and we can set different logging levels as needed. We can record detailed information during development and only record important information in the production environment. How flexible!
Stack Tracing
When an exception occurs in the program, understanding the context of the exception is crucial. Python's traceback module can help us get detailed stack trace information:
import traceback
def function_c():
raise ValueError("This is an intentional error")
def function_b():
function_c()
def function_a():
try:
function_b()
except Exception as e:
print("An error occurred:")
print(traceback.format_exc())
function_a()
When you run this code, you'll see detailed error information, including the location of each function call. This is very helpful for locating errors in complex programs.
IDE Section
After all this, you might ask: Do I have to write these debugging codes manually every time? Don't worry, modern IDEs have provided us with powerful debugging tools, making debugging more intuitive and efficient.
PyCharm: Professional Python IDE
PyCharm is a professional Python IDE developed by JetBrains, and its debugging features are very powerful.
- Breakpoint setting: You can set or cancel breakpoints by clicking next to the code line number.
- Variable watching: In the Debug window, you can view the values of all variables in real-time.
- Conditional breakpoints: You can set breakpoints that are only triggered when specific conditions are met.
- Expression evaluation: During debugging, you can calculate the value of any Python expression at any time.
A typical scenario of using PyCharm for debugging:
def calculate_fibonacci(n):
if n <= 1:
return n
else:
return calculate_fibonacci(n-1) + calculate_fibonacci(n-2)
result = calculate_fibonacci(10)
print(result)
In this example, you can set a breakpoint on the first line of the calculate_fibonacci function and then start debugging. You'll see the recursive calling process of the function and how the value of n changes with each call. This is very helpful for understanding recursive algorithms.
Visual Studio Code: Lightweight but Powerful
If you prefer a more lightweight IDE, Visual Studio Code (VS Code) is a good choice. Its Python debugging features are also quite powerful:
- Debug console: You can execute Python code here and check variable values.
- Stack trace visualization: VS Code displays the call stack graphically, making it easy to understand.
- Multi-thread debugging: For multi-threaded programs, VS Code allows you to debug multiple threads simultaneously.
Let's look at an example of debugging a multi-threaded program using VS Code:
import threading
import time
def worker(name):
print(f"Thread {name} starts working")
time.sleep(2)
print(f"Thread {name} finishes working")
threads = []
for i in range(3):
t = threading.Thread(target=worker, args=(f"Thread-{i}",))
threads.append(t)
t.start()
for t in threads:
t.join()
print("All threads finished working")
When debugging this code in VS Code, you can clearly see the status and execution process of each thread. This is very helpful for understanding and debugging concurrent programs.
Summary
Alright, we've delved deep into various techniques and tools for Python debugging. From simple print statements to powerful IDE debugging features, each method has its applicable scenarios.
Remember, debugging is not just about finding and fixing errors, but also an excellent opportunity to understand how code runs. Through debugging, you can gain a deeper understanding of Python's internal workings and improve your programming skills.
So, which debugging method do you like best? Are you eager to try them out? Go ahead, make those nasty bugs have nowhere to hide!
Finally, I want to say that although debugging can sometimes be frustrating, the sense of achievement when you finally solve the problem is incomparable. So, don't be afraid of bugs, treat them as opportunities for learning and growth.
Do you have any unique debugging techniques? Feel free to share your experiences in the comments! Let's become Python debugging masters together!
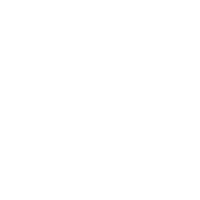
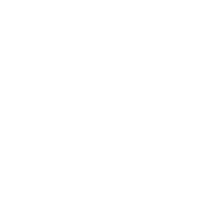