Have you ever dreamed of creating your own game world? Are you passionate about programming and game development? If so, you've come to the right place! Today, let's delve into the mysteries of Python game development and see how this powerful and flexible language can help us realize our game development dreams.
Origins
Do you remember the excitement when I first encountered Python game development? That feeling of being able to create interactive worlds with simple code was simply irresistible. From then on, I embarked on this challenging and fun-filled journey. Today, I want to share with you my experiences in this field, hoping to provide some inspiration and guidance for your game development journey.
Applicability
First, let's talk about the applicability of Python in game development. You might ask, "Is Python really suitable for making games?" The answer is definitely yes! While Python may not be the first choice for game development, it certainly has its unique advantages.
Python's concise syntax and rich library resources make rapid prototype development exceptionally easy. Imagine having a brilliant game idea and being able to build a working prototype in just a few hours using Python. This kind of instant feedback is invaluable for idea validation and iteration.
Moreover, Python's cross-platform nature is a major highlight. Did you know that games developed using Python can easily run on different operating systems like Windows, Mac, and Linux? This provides the possibility for your game to win a wider audience.
However, we also need to face some limitations of Python in game development. For example, Python might be slightly inferior in execution speed compared to low-level languages like C++. But don't worry! We have ways to overcome this problem. By using some optimization techniques and tools, we can make Python games run really fast.
Core
Speaking of core technologies, the Pygame library is definitely a star in Python 2D game development. Have you used Pygame? If not, I strongly recommend you give it a try. Pygame provides a simple yet powerful set of tools that allow us to easily handle core elements of game development such as graphics, sound, and user input.
For instance, collision detection is a big challenge in game development. But with Pygame, we can easily implement this feature. You just need to create rectangles for each game object (using pygame.Rect
) and then use the colliderect()
method to detect collisions, it's that simple!
player_rect = pygame.Rect(player_x, player_y, player_width, player_height)
enemy_rect = pygame.Rect(enemy_x, enemy_y, enemy_width, enemy_height)
if player_rect.colliderect(enemy_rect):
print("Collision occurred!")
See, it's that intuitive! This code detects whether a collision has occurred between the player and the enemy, and if so, it prints a message. Based on this principle, you can easily implement various collision effects in your game.
Sound effects and music are also indispensable elements in games. Remember those unforgettable game soundtracks? Using Pygame's mixer
module, we can easily add sound effects and background music to our games.
pygame.mixer.init()
background_music = pygame.mixer.music.load("background.mp3")
pygame.mixer.music.play(-1) # -1 means loop playback
sound_effect = pygame.mixer.Sound("explosion.wav")
sound_effect.play()
This code demonstrates how to load and play background music and sound effects. Isn't it simple? You can use this method to add various sound effects to your game, making the game experience richer.
For those who want to challenge 3D game development, the Panda3D engine is a good choice. It allows us to write 3D games using Python, and if needed, we can even port part of the code to C++ to improve performance. This flexibility is really great, isn't it?
Optimization
Speaking of performance, this might be a major concern for many people about Python game development. But don't worry, we have many ways to optimize the performance of Python games.
First, using OpenGL and Pyglet can significantly improve the rendering performance of games. OpenGL is a powerful graphics library, while Pyglet provides a concise Python interface to use OpenGL. By combining these two, we can achieve efficient graphics rendering.
import pyglet
from pyglet.gl import *
window = pyglet.window.Window()
@window.event
def on_draw():
glClear(GL_COLOR_BUFFER_BIT)
glLoadIdentity()
glBegin(GL_TRIANGLES)
glVertex2f(0, 0)
glVertex2f(window.width, 0)
glVertex2f(window.width, window.height)
glEnd()
pyglet.app.run()
This code uses Pyglet and OpenGL to draw a simple triangle. Although this is just a basic example, it demonstrates how to use these tools for efficient graphics rendering.
Additionally, Cython and PyPy are two powerful tools for improving Python performance. Cython allows us to compile Python code into C code, greatly increasing execution speed. PyPy is a just-in-time compiler that can significantly boost the running speed of Python code.
%%cython
def fast_function(int x, int y):
cdef int result = x * y
return result
This simple example shows how to use Cython to optimize a function. By adding type declarations, we can make Python code run faster.
AI Implementation
Game AI is another exciting topic. Have you ever thought about how to make characters in games behave more intelligently and interestingly?
A simple and effective method is to use state machines. We can define different states for game characters (such as patrol, chase, attack, etc.), and then switch between these states based on conditions in the game.
class EnemyAI:
def __init__(self):
self.state = "patrol"
def update(self, player_position):
if self.state == "patrol":
self.patrol()
if self.detect_player(player_position):
self.state = "chase"
elif self.state == "chase":
self.chase(player_position)
if self.in_attack_range(player_position):
self.state = "attack"
elif self.state == "attack":
self.attack()
if not self.in_attack_range(player_position):
self.state = "chase"
def patrol(self):
# Implement patrol logic
pass
def chase(self, player_position):
# Implement chase logic
pass
def attack(self):
# Implement attack logic
pass
def detect_player(self, player_position):
# Implement player detection logic
pass
def in_attack_range(self, player_position):
# Implement attack range detection logic
pass
This code demonstrates a simple enemy AI state machine. The enemy can switch between three states: patrol, chase, and attack, deciding its behavior based on the player's position and other game conditions. You can design more complex and intelligent behavior patterns for your game characters based on this framework.
Practice
We've talked about a lot of theory, let's get practical! Creating a simple 2D platform game is a good way to get started with Python game development. Let's look at the basic steps:
- Set up the game window
- Handle user input
- Update game state
- Draw graphics
Here's a simple example showing how to create a basic game loop:
import pygame
pygame.init()
screen = pygame.display.set_mode((800, 600))
clock = pygame.time.Clock()
player_x = 400
player_y = 300
running = True
while running:
# Handle user input
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
# Update game state
keys = pygame.key.get_pressed()
if keys[pygame.K_LEFT]:
player_x -= 5
if keys[pygame.K_RIGHT]:
player_x += 5
# Draw graphics
screen.fill((0, 0, 0)) # Clear screen
pygame.draw.rect(screen, (255, 0, 0), (player_x, player_y, 50, 50)) # Draw player
pygame.display.flip() # Update display
clock.tick(60) # Control frame rate
pygame.quit()
This code creates a simple game where the player can move a red square using the left and right arrow keys. Although simple, it contains the basic elements of game development: initialization, game loop, input handling, state updating, and graphics drawing.
You can add more features to this foundation, such as jumping mechanics, obstacles, scoring systems, etc., gradually building a complete platform game.
Conclusion
Python game development is a field full of challenges and fun. From simple 2D games to complex 3D worlds, Python can handle it all. Although it may not be as good as languages like C++ in some aspects, its simplicity and rich library resources make rapid development and prototype design exceptionally easy.
Remember, game development is a process of continuous learning and practice. Don't be afraid to make mistakes, every failure is a step towards success. Keep your curiosity and be brave to try new technologies and methods.
Do you have any game development ideas or experiences you'd like to share? Or do you have any questions about Python game development? Feel free to leave a message in the comments section, let's discuss and progress together.
Finally, I want to say that whether you're just starting to get into game development or already have some experience, Python is a choice worth trying. It may not be the fastest, but it's definitely one of the friendliest and easiest languages to get started with. So, are you ready to start your Python game development journey? Let's create something fun together!
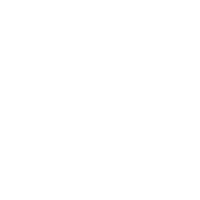
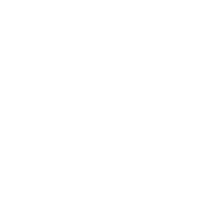