Hey, dear Python enthusiasts! Today, we're diving into a super interesting topic - Python game development. Have you always dreamed of creating your own game world? Or maybe you're just looking for a fun way to improve your programming skills? Either way, Python game development is an excellent choice. Let's explore this magical field and see how you can realize your game dreams with Python!
Why Choose Python?
You might ask, why choose Python for game development? Great question! Let me give you a few compelling reasons:
-
Easy to Learn: Python's syntax is clear and straightforward, especially friendly for beginners. You don't need to spend a lot of time understanding complex syntax rules, allowing you to focus more on implementing game logic.
-
Rich Library Resources: Python has a vast number of third-party libraries, including powerful tools specifically for game development. For example, Pygame and Panda3D can make your game development much more efficient.
-
Cross-Platform Compatibility: Games developed with Python can often run on multiple platforms, meaning your creations can be enjoyed and used by more people.
-
Active Community Support: The Python community is very active, and you can easily find various tutorials, example code, and solutions. When you encounter problems, there's always a helpful developer willing to assist you.
-
Rapid Prototyping: Python allows you to quickly build game prototypes, which is very beneficial for testing game ideas and iterative development.
Getting Started
Alright, now that you're captivated by the charm of Python game development, let's see what you need to get started!
1. Python Environment
First, you need to install Python on your computer. I suggest installing the latest stable version, like Python 3.9 or 3.10. The installation process is simple: just download the installer for your operating system from the Python website and follow the instructions.
Once installed, open the command line or terminal and enter the following command to check if the installation was successful:
python --version
If it shows the Python version number, the installation was successful!
2. Integrated Development Environment (IDE)
While you can write Python code with any text editor, I highly recommend using a good IDE. My personal favorite is PyCharm, which offers many powerful features like code completion and debugging tools. However, if you find PyCharm too heavy, you might consider Visual Studio Code, which is lighter and has rich plugin support for Python development.
3. Game Development Library
Next, you need to choose a game development library. For beginners, I strongly recommend Pygame. It's simple to use, yet powerful enough for 2D game development. You can install Pygame via pip:
pip install pygame
Start Your Game Journey
Now that the preparations are done, it's time to start your actual game development journey! Let's begin with a simple example and gradually delve into the world of game development.
Hello, Pygame!
First, let's create the most basic Pygame window, which will be the "canvas" of your game world. Create a new Python file, perhaps named hello_pygame.py
, and enter the following code:
import pygame
import sys
pygame.init()
screen = pygame.display.set_mode((800, 600))
pygame.display.set_caption("Hello, Pygame!")
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
# Fill background color
screen.fill((255, 255, 255)) # White
# Update display
pygame.display.flip()
Run this code, and you should see a white window with the title "Hello, Pygame!" Congratulations, you've just created your first Pygame program!
Let me explain this code:
- First, we imported the necessary modules:
pygame
andsys
. - Then, we initialized Pygame, which is a necessary step to use it.
- Next, we created a window of 800x600 pixels and set the title.
- In the main loop, we continuously check for events (like the user closing the window) and update the screen display.
This example is simple, but it contains the basic structure of all Pygame games. Next, we can add more game elements based on this foundation.
Adding Game Elements
Now that we have a basic window, let's add some game elements to make it more like a game. We'll create a simple bouncing ball game.
Create a new file named bouncing_ball.py
and enter the following code:
import pygame
import sys
pygame.init()
width, height = 800, 600
screen = pygame.display.set_mode((width, height))
pygame.display.set_caption("Bouncing Ball")
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
RED = (255, 0, 0)
ball_x, ball_y = width // 2, height // 2
ball_radius = 20
ball_speed_x, ball_speed_y = 5, 5
clock = pygame.time.Clock()
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
# Move the ball
ball_x += ball_speed_x
ball_y += ball_speed_y
# Check for collisions
if ball_x <= ball_radius or ball_x >= width - ball_radius:
ball_speed_x = -ball_speed_x
if ball_y <= ball_radius or ball_y >= height - ball_radius:
ball_speed_y = -ball_speed_y
# Fill background color
screen.fill(WHITE)
# Draw the ball
pygame.draw.circle(screen, RED, (int(ball_x), int(ball_y)), ball_radius)
# Update display
pygame.display.flip()
# Control frame rate
clock.tick(60)
Run this code, and you'll see a red ball bouncing around the screen. Cool, right?
Let's analyze this code:
- We defined the ball's initial position, size, and speed.
- In the main loop, we update the ball's position and check if it hits the boundaries. If it does, we reverse the speed to make the ball bounce.
- We use
pygame.draw.circle()
to draw the ball. - Finally, we use
clock.tick(60)
to control the game's frame rate, ensuring it doesn't run too fast.
Adding Interactivity
So far, our game has no player interaction. Let's change that by adding a paddle that the player can control.
Modify the bouncing_ball.py
file, updating it with the following content:
import pygame
import sys
pygame.init()
width, height = 800, 600
screen = pygame.display.set_mode((width, height))
pygame.display.set_caption("Pong Game")
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
RED = (255, 0, 0)
BLUE = (0, 0, 255)
ball_x, ball_y = width // 2, height // 2
ball_radius = 20
ball_speed_x, ball_speed_y = 5, 5
paddle_width, paddle_height = 100, 20
paddle_x = (width - paddle_width) // 2
paddle_y = height - paddle_height - 10
clock = pygame.time.Clock()
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
# Move the paddle
keys = pygame.key.get_pressed()
if keys[pygame.K_LEFT] and paddle_x > 0:
paddle_x -= 7
if keys[pygame.K_RIGHT] and paddle_x < width - paddle_width:
paddle_x += 7
# Move the ball
ball_x += ball_speed_x
ball_y += ball_speed_y
# Check for collisions
if ball_x <= ball_radius or ball_x >= width - ball_radius:
ball_speed_x = -ball_speed_x
if ball_y <= ball_radius:
ball_speed_y = -ball_speed_y
# Check if the ball hits the paddle
if (ball_y >= paddle_y - ball_radius and
paddle_x < ball_x < paddle_x + paddle_width):
ball_speed_y = -ball_speed_y
# Check if the ball hits the bottom
if ball_y >= height - ball_radius:
print("Game Over!")
pygame.quit()
sys.exit()
# Fill background color
screen.fill(WHITE)
# Draw the ball
pygame.draw.circle(screen, RED, (int(ball_x), int(ball_y)), ball_radius)
# Draw the paddle
pygame.draw.rect(screen, BLUE, (paddle_x, paddle_y, paddle_width, paddle_height))
# Update display
pygame.display.flip()
# Control frame rate
clock.tick(60)
Now, you can use the left and right arrow keys to control the paddle. Your goal is to prevent the ball from falling to the bottom of the screen. If it does, the game ends.
Let's look at what's new in this version:
- We added a paddle and defined its position and size.
- In the main loop, we check for keyboard input to move the paddle based on the left and right arrow keys.
- We added collision detection to check if the ball hits the paddle. If it does, the ball bounces.
- If the ball hits the bottom, the game ends.
Adding a Scoring System
To make the game more interesting, we can add a scoring system. Each time the ball hits the paddle, the player scores a point. Let's update our code:
import pygame
import sys
pygame.init()
width, height = 800, 600
screen = pygame.display.set_mode((width, height))
pygame.display.set_caption("Pong Game")
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
RED = (255, 0, 0)
BLUE = (0, 0, 255)
ball_x, ball_y = width // 2, height // 2
ball_radius = 20
ball_speed_x, ball_speed_y = 5, 5
paddle_width, paddle_height = 100, 20
paddle_x = (width - paddle_width) // 2
paddle_y = height - paddle_height - 10
score = 0
font = pygame.font.Font(None, 36)
clock = pygame.time.Clock()
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
# Move the paddle
keys = pygame.key.get_pressed()
if keys[pygame.K_LEFT] and paddle_x > 0:
paddle_x -= 7
if keys[pygame.K_RIGHT] and paddle_x < width - paddle_width:
paddle_x += 7
# Move the ball
ball_x += ball_speed_x
ball_y += ball_speed_y
# Check for collisions
if ball_x <= ball_radius or ball_x >= width - ball_radius:
ball_speed_x = -ball_speed_x
if ball_y <= ball_radius:
ball_speed_y = -ball_speed_y
# Check if the ball hits the paddle
if (ball_y >= paddle_y - ball_radius and
paddle_x < ball_x < paddle_x + paddle_width):
ball_speed_y = -ball_speed_y
score += 1 # Increase score
# Check if the ball hits the bottom
if ball_y >= height - ball_radius:
print(f"Game Over! Final Score: {score}")
pygame.quit()
sys.exit()
# Fill background color
screen.fill(WHITE)
# Draw the ball
pygame.draw.circle(screen, RED, (int(ball_x), int(ball_y)), ball_radius)
# Draw the paddle
pygame.draw.rect(screen, BLUE, (paddle_x, paddle_y, paddle_width, paddle_height))
# Display score
score_text = font.render(f"Score: {score}", True, BLACK)
screen.blit(score_text, (10, 10))
# Update display
pygame.display.flip()
# Control frame rate
clock.tick(60)
In this version, we've added the following new features:
- Initialized a
score
variable to track the score. - Increased the score by 1 each time the ball hits the paddle.
- Used Pygame's font feature to display the current score on the screen.
- Displayed the final score when the game ends.
Further Improvements
How do you like the game so far? Isn't it fun already? But as a creative developer, I'm sure you have more ideas. Here are some suggestions for further improving the game:
-
Increasing Difficulty: As the score increases, you can make the ball move faster or make the paddle shorter to increase the game's difficulty.
-
Multiple Balls: Why only one ball? We can add multiple balls to make the game more challenging.
-
Special Items: You can randomly generate special items on the screen, such as temporarily lengthening the paddle or slowing down the ball.
-
Sound Effects: Add sound effects for events like the ball hitting the paddle or boundaries to enrich the game experience.
-
Level System: Design multiple levels, each with different layouts and challenges.
-
Leaderboard: Record players' high scores and display a leaderboard.
-
Pause Function: Allow players to pause the game.
-
Start Screen: Add a start screen where players can choose to start the game or view instructions.
Implementing these features will not only make your game more fun but also help you learn more about Python and Pygame. Each new feature is a learning opportunity that can take your programming skills to the next level.
Conclusion
Through this simple bouncing ball game, we've learned the basics of Python game development, including how to create a game window, handle user input, move game objects, detect collisions, and more. This is just the tip of the iceberg in game development, but it's enough to start creating your own game world.
Remember, game development is a process that requires continuous learning and practice. Don't be afraid to make mistakes; every bug is a learning opportunity. Stay curious, be brave to try new ideas, and you'll find the fun of Python game development is endless.
Finally, I want to say that programming is like magic, and game development is one of the most magical things. With just a few lines of code, you can create a whole new world, isn't that exciting? So, keep exploring, keep creating, and let your imagination soar in the code!
Alright, dear Python game developer, are you ready to start your game development journey? Remember to share your creations often and listen to feedback from others. Who knows, maybe your next game will be the next big hit!
Have fun and learn a lot on your Python game development journey! If you have any questions or ideas, feel free to let me know. Let's explore this magical programming world together!
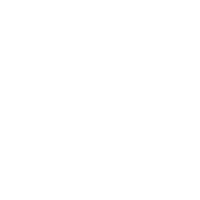
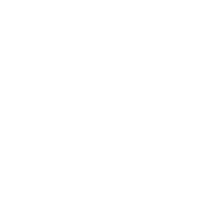