Hello everyone, today we're going to talk about how to learn Python game development from scratch. Game development might sound complex, but getting started isn't actually that difficult, especially when you choose Python, a simple and easy-to-learn language. However, before we start making games, we need to lay some groundwork.
Preparing the Basics
Python Language Basics
As a programming novice, you first need to master Python's basic syntax. Things like variables, loops, and functions can be considered the "big three" of programming basics. Fortunately, Python syntax is very concise and easy to understand, and you only need to spend a few days to get started. However, merely staying at the syntax level is not enough; you need to practice more to apply these syntaxes fluently.
My suggestion is to first look at Python beginner tutorials to understand how to use the syntax. Then start writing some small programs, such as printing the multiplication table in the console, a calculator, etc. Only through practice can you truly master Python. Oh, and if you have any questions, don't forget to search online. There are plenty of questions and answers on Stack Overflow for reference.
Game Development Concepts
To learn game development, knowing how to code is not enough; you also need to understand some basic game development concepts. For example, collision detection, scoring systems, and so on. Although these concepts may sound a bit dry, they are essential for making games.
My personal advice is not to rush into making games. It's better to watch some game development tutorials first to understand these basic concepts. Once you have a certain understanding of these concepts, it will be much easier when you start making games. There's an old saying, "To do a good job, an artisan needs the best tools." Mastering these basic knowledge is laying the foundation for your "tools".
Development Tools and Frameworks
After acquiring the basic knowledge, we can start preparing development tools and frameworks. For Python game development, there are two essential frameworks.
Pygame Library
Pygame is an excellent Python game development library that provides most of the functions needed for game development, such as graphics drawing, event handling, sound playback, and so on. With Pygame, we can quickly develop various 2D games.
Installing Pygame is very simple; you can do it directly with pip. However, it's worth noting that Pygame only provides the most basic functions. For advanced features like sprite animations and particle effects, you need to write the code yourself. So while learning Pygame, it's also important to accumulate game development experience.
My personal experience is that it's best to start with some simple games, like the classic Snake or Breakout. Through practice, you will gradually master how to use Pygame and also accumulate game development experience. Once you're familiar enough with Pygame, trying to develop more complex games will become much easier.
Phaser3 Framework
Besides Pygame, Phaser3 is also an excellent game development framework. Unlike Pygame which is purely Python-based, Phaser3 is developed based on HTML5 technology. With Phaser3, we can develop HTML5 games that run in browsers.
Compared to Pygame, Phaser3's advantage is that it provides more out-of-the-box features, such as sprite animations, particle systems, physics engines, and so on. It also supports various modern browsers, making it very cross-platform. However, its disadvantage is that the learning curve is relatively steep, making it more challenging for beginners to get started.
If you're already familiar with Pygame, it's worth learning Phaser3 as well. After all, game development covers a wide range, and it's very necessary to master different frameworks and technologies. However, I suggest you still start with simple games, like recreating the classic game "Tank Battle". Once you're familiar with Phaser3's development mode, you can try developing larger games later.
Game Development Practice
With development tools and basic knowledge, we can start practical game development. At the beginning, making some simple small games is the best choice.
Snake Game
Snake is definitely an "old friend" of programmers. Although the rules of this game are simple, it's challenging enough for beginners. In the process of making this game, you need to handle keyboard input, collision detection, scoring system, and many other aspects.
My personal suggestion is to make a basic version first, with only the snake and food, no obstacles or scores. Once you're familiar with the basic structure of the game, gradually add new features, such as adding obstacles, scoring system, etc. Through continuous iteration, you'll find your code becoming more elegant and the game experience getting better.
Breakout Game
The Breakout game is a bit more difficult compared to Snake, but it's definitely a very good practice project. When making this game, you need to handle the ball's rebound, brick collision detection, game over judgment, and many other logics.
I suggest you can make a basic version first, just with a paddle and a ball, keeping the ball's rebound logic as simple as possible. Once you've mastered the basic development mode, gradually add new features, such as adding different levels of bricks, life system, etc.
By the way, when making these two games, don't be discouraged if you encounter any difficulties. Look up information online or ask questions in forums, you can usually find solutions. After all, with so many predecessors who have walked this path, we just need to follow along.
Advanced Techniques
After making some simple games, do you feel that game development isn't that difficult? That's right, getting started is easy, but to make a truly excellent game, you need to master some advanced techniques.
Scene Switching
In most games, we need to switch between different scenes, such as switching from the main menu to the game scene, from the game scene to the end scene, and so on. To implement this scene switching, we need to master some advanced techniques.
Taking Phaser3 as an example, it provides multiple methods for scene switching. The most commonly used are scene.start(key)
and scene.switch(key)
. The former destroys the current scene and switches to a new scene; the latter preserves the state of the current scene and switches to a new scene.
You can choose the appropriate switching method according to the specific needs of the game. For example, when switching to a new level during the game, you can use the switch
method; while returning from the game to the main menu, you might need the start
method to completely destroy the game scene.
Collision Detection and Scoring
In addition to scene switching, collision detection and scoring systems are also two essential aspects of game development. Fortunately, whether it's Pygame or Phaser3, they both provide ready-made APIs for us, we just need to call them according to the documentation.
Taking Pygame as an example, it provides the sprite.spritecollide
method to detect collisions between sprites. The scoring system is even simpler, you just need to maintain a score variable in the game logic and increase or decrease the score when specific events occur.
However, just calling APIs is not enough, we also need to think about how to design game rules. For example, in the Breakout game, we need to decide the score values for different types of bricks; in the Snake game, we need to decide how to increase the score after the snake eats food, and so on. Good game rule design can give players a better experience.
Development Methodology
In addition to the specific techniques mentioned above, when doing game development, we also need to master some general development methodologies to improve code quality and development efficiency.
Test-Driven Development
Test-Driven Development (TDD) is a widely used agile development methodology. Its core idea is to write test cases first, then write code to pass the tests. Through this method, we can ensure the correctness of the code and make it easier to find and fix bugs.
However, TDD is not a common practice in game development. This is mainly because game development usually requires a lot of visual and interactive design, which is difficult to cover with automated tests. Also, game development requires rapid iteration, and TDD might slow down the development speed.
So in game development, manual testing is more common. That is, we constantly run the game during the development process and manually check if each function is working properly. Although this method is less efficient, it can comprehensively check various aspects of the game.
Version Control
Whether it's making games or other software projects, version control is an essential part. It can help us track the change history of code, facilitate collaborative development, and prevent accidents like code loss.
For Python game development, Git is a very good version control tool. We can create a code repository on GitHub or GitLab and use Git to manage the code. Every time a new feature is developed or a bug needs to be fixed, we can create a new commit and push it to the remote repository.
In addition to daily version control, we also need to create official release versions for the game. Taking GitHub as an example, we can use GitHub Actions to automate this process. Specifically, we need to configure a workflow that automatically creates a release and packages and uploads the game code under specific conditions (such as pushing a new tag).
In this way, players can directly download the latest version of the game from GitHub, without having to clone the code repository and compile it manually. This will undoubtedly greatly improve user experience.
Application of Algorithms in Games
Finally, let's talk about the application of algorithms in game development. You might think that algorithms are far from game development, but that's not the case. Many classic algorithms can be applied to game development, helping us solve some complex problems.
Search Algorithms
In many games, we need to find a path from a starting point to an endpoint. This is where search algorithms come in. Taking Breadth-First Search (BFS) as an example, it continuously expands all neighboring nodes of the current node until it finds the target node, thus obtaining the shortest path.
We can apply BFS to puzzle games. By treating each state of the game as a node, BFS can find the shortest path from the initial state to the target state, which is the minimum number of steps.
Specifically, we need to define a queue to store nodes to be visited, and a set to store visited nodes. Starting from the initial state, we add it to the queue and the set. Then we continuously take nodes out of the queue, generate all their next step states, and for unvisited states, add them to the queue and the set. When we find the target state, we have obtained the shortest path.
Besides BFS, there are many other search algorithms that can be applied to game development, such as A algorithm, IDA algorithm, and so on. Mastering these algorithms can make our games smarter and enhance the gaming experience.
Summary
Alright, that's all for today's sharing. Through the above introduction, you should have a preliminary understanding of how to learn Python game development from scratch. Of course, this is just the beginning. To become an excellent game developer, you still need to continuously learn and improve in practice.
My advice is to first lay a good foundation, mastering Python syntax and game development concepts. Then learn game development frameworks like Pygame or Phaser3, and accumulate experience through practice. During the development process, also pay attention to mastering some advanced techniques, such as scene switching, collision detection, etc. In addition, good development methodologies and algorithm knowledge are also necessary to become an excellent developer.
Finally, although the road is long, as long as you persist, you will definitely reach the end. Come on, programmers! Let's go further and further on the road of game development!
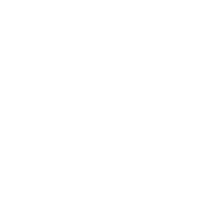