Hello, dear Python enthusiasts! Today we're going to explore a very interesting topic - Python game development. As someone who loves programming, I believe you must be curious and excited about creating game worlds using your familiar language. So, let's begin this wonderful journey!
Origins
Do you remember the first time you encountered electronic games? Maybe it was playing Street Fighter in an arcade, or Super Mario on a Nintendo at home. Back then, we were simply immersed in the fun of games, never imagining that one day we could create such worlds ourselves.
As my programming skills improved, I began to wonder: could I develop games using Python? After all, Python has concise syntax and a gentle learning curve, making it very suitable for rapid prototype development. After some research, I was delighted to discover that Python has great potential in the field of game development!
Advantages
You might ask, isn't Python known for web development and data analysis? Can it really be used for game development? The answer is definitely yes! Python has unique advantages in game development:
-
Concise and easy to learn: Python's syntax is simple and clear, allowing even programming beginners to quickly get started. This lets us focus more energy on game logic and creativity, rather than being bogged down by complicated syntax.
-
Rich libraries: Python has a large number of mature game development libraries, such as Pygame and Arcade. These libraries provide comprehensive support from graphics rendering to audio processing, greatly reducing development difficulty.
-
Cross-platform: Games developed using Python can easily run on multiple platforms such as Windows, Mac, and Linux without requiring extensive code modifications.
-
Rapid prototyping: Python's advanced features and dynamic typing allow us to quickly implement ideas, making it very suitable for game prototype development and testing.
-
Community support: Python has a large and active community where you can easily find various tutorials, examples, and solutions.
Of course, Python also has some limitations in game development. For example, when dealing with complex 3D graphics or large-scale multiplayer online games, its performance may not be as good as lower-level languages like C++. However, for most independent game developers and small teams, Python is already more than capable.
Toolbox
When it comes to Python game development, we must mention some powerful tools and libraries. Let's take a look at these magical weapons!
2D Game Development
For 2D game development, Pygame is undoubtedly one of the most popular choices. It provides simple and easy-to-use APIs that can easily handle graphics, sound, and input devices. I remember being amazed by its simplicity and power when I first used Pygame. With just a few lines of code, you can draw graphics on the screen, play sound effects, and implement basic game logic.
import pygame
pygame.init()
screen = pygame.display.set_mode((400, 300))
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
screen.fill((255, 255, 255))
pygame.draw.circle(screen, (0, 0, 255), (200, 150), 30)
pygame.display.flip()
pygame.quit()
This code creates a simple window and draws a blue circle in it. See, it's that simple!
Besides Pygame, Arcade is also a good choice. It's a more modern 2D game library that offers better performance and more user-friendly features. If you're looking for a more modern 2D game development tool, Arcade is definitely worth a try.
3D Game Development
When it comes to 3D game development, Panda3D is a gem in the Python world. It allows us to write 3D games using Python, and when needed, parts of the code can be ported to C++ to improve performance. Although it might be a bit complex for beginners, it demonstrates Python's potential in 3D game development.
I once used Panda3D to create a simple 3D maze game. Although the process was somewhat arduous, the sense of achievement when seeing the 3D world I created appear on the screen was incomparable.
Technical Exploration
During the game development process, we often encounter some technical challenges. Let's discuss a few common problems and their solutions.
Game Physics Implementation
Physics engines are an important component in game development. They are responsible for simulating real-world physical laws, such as gravity and collisions. In Python, we can use Pygame's built-in functions to implement simple physical effects, such as collision detection:
import pygame
pygame.init()
screen = pygame.display.set_mode((400, 300))
clock = pygame.time.Clock()
ball = pygame.Rect(200, 150, 20, 20)
speed = [2, 2]
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
ball.move_ip(speed)
if ball.left < 0 or ball.right > 400:
speed[0] = -speed[0]
if ball.top < 0 or ball.bottom > 300:
speed[1] = -speed[1]
screen.fill((255, 255, 255))
pygame.draw.ellipse(screen, (0, 0, 255), ball)
pygame.display.flip()
clock.tick(60)
pygame.quit()
This code creates a small ball that bounces on the screen. Through simple boundary detection and speed reversal, we've achieved a basic collision effect.
For more complex physical simulations, we can use professional physics engines like Pymunk or Box2D. These libraries provide more advanced physical simulation functions that can handle complex collisions, gravity, and other physical phenomena.
Performance Optimization
As the scale of the game increases, performance optimization becomes increasingly important. In Python game development, we have several methods to improve performance:
-
Use Cython to compile critical code: Cython can compile Python code into C, greatly improving execution speed.
-
Utilize PyPy's JIT compiler: PyPy is an alternative implementation of Python that uses just-in-time compilation technology, which can significantly improve the execution speed of certain types of code.
-
Optimize algorithms and data structures: Choosing appropriate algorithms and data structures can greatly enhance performance. For example, using spatial partitioning algorithms can greatly reduce the computational load of collision detection.
-
Use performance profiling tools: Python's cProfile module can help us find performance bottlenecks in our programs.
import cProfile
def game_loop():
# Main game loop code
cProfile.run('game_loop()')
This code will analyze the performance of the game_loop function, helping us find areas that need optimization.
Graphics Rendering Optimization
For graphics-intensive games, rendering optimization is particularly important. We can consider using OpenGL to accelerate graphics rendering. Pyglet is a lightweight OpenGL wrapper library that can help us better utilize GPU performance.
Multiplayer Game Development
With the development of network technology, multiplayer online games have become increasingly popular. Python also provides us with tools for developing multiplayer games.
Network Programming Libraries
Python's standard library includes the socket module, which can be used for low-level network programming. However, for game development, I recommend using high-level network libraries like Twisted. Twisted provides support for asynchronous I/O, which can better handle a large number of concurrent connections.
from twisted.internet import reactor, protocol
class GameProtocol(protocol.Protocol):
def dataReceived(self, data):
# Handle received data
self.transport.write(response)
class GameFactory(protocol.Factory):
def buildProtocol(self, addr):
return GameProtocol()
reactor.listenTCP(8000, GameFactory())
reactor.run()
This code creates a simple game server that can handle client connections and data exchange.
Game Server Frameworks
For more complex multiplayer games, we can consider using specialized game server frameworks. Django Channels and Flask-SocketIO are both good choices, providing real-time communication and player state management functions.
Using these frameworks, we can more easily implement features like room systems and real-time battles. For example, using Flask-SocketIO, we can implement a simple chat room like this:
from flask import Flask, render_template
from flask_socketio import SocketIO, emit
app = Flask(__name__)
socketio = SocketIO(app)
@app.route('/')
def index():
return render_template('index.html')
@socketio.on('message')
def handle_message(message):
emit('message', message, broadcast=True)
if __name__ == '__main__':
socketio.run(app)
This code creates a basic chat server where clients can send and receive messages in real-time via WebSocket.
Conclusion
Python game development is a field full of challenges and fun. From simple 2D mini-games to complex 3D multiplayer online games, Python can handle it all. Although it may not be as good as lower-level languages like C++ in some aspects, Python's simplicity and rich ecosystem make it an excellent choice for game development, especially independent game development.
Are you eager to start your Python game development journey? Remember, the most important thing is to maintain enthusiasm and creativity. Don't get bogged down by technical details, focus on realizing your game ideas. Trust me, when you see your game running on the screen, that sense of achievement is incomparable!
So, are you ready to start your Python game development adventure? Let's explore together in this world full of infinite possibilities!
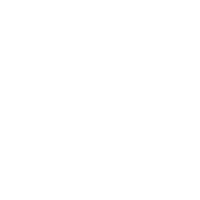
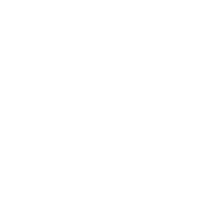