Hey guys! Today we're going to talk about Python's applications in the game development field. Many people have doubts about whether Python is suitable for game development, but in fact, Python has a lot to offer in this area.
Natural Advantages
First, let's look at some of Python's unique advantages in game development. Did you know that Python has very powerful integration capabilities? It can seamlessly combine with other languages. For example, the Panda3D engine allows you to write 3D games in Python while also being able to port some code to run on C++. Although this might be a bit "overkill" for some small projects, it demonstrates Python's power as a "glue" language.
Moreover, through libraries like Pyglet and PyGame, Python can also call OpenGL to reduce rendering pressure. If you're very concerned about game performance, don't worry, because you can completely use Cython to compile Python code into C extension modules, or use PyPy for JIT compilation, thus gaining a significant performance boost.
So, you can completely prototype a game demo quickly with Python, and then, once the requirements and ideas are formed, optimize the performance bottlenecks specifically. Isn't that killing two birds with one stone?
Complete Toolkit
In the Python game development ecosystem, there are many excellent libraries and frameworks to choose from, such as:
- Pygame: A cross-platform 2D game engine library that provides various modules needed for game development, including audio, images, fonts, etc.
- Pyglet: An object-oriented window and multimedia library that can be used for game, multimedia, and various other application development.
- Cocos2D: A well-known game engine that provides various functions needed for development such as 2D sprites, actions, animations, etc.
- Panda3D: A 3D game engine written in Python and C++ that can be used to create 3D animations and games.
In addition to these well-known engines and libraries, Python game development has many other auxiliary libraries to choose from, such as PyGame Mixer for handling sound, PyGame Font for handling fonts, and so on. In short, the tools are complete and comprehensive.
Technical Breakdown
Alright, since the tools are so abundant, let's break down some of the core technical points in Python game development.
Collision Detection
In game development, collision detection is undoubtedly a very important part. In Pygame, we usually use the pygame.sprite.spritecollide()
method to detect collisions between sprites. But if more precise collision detection is needed, you'll need to use the pygame.mask
module.
This module allows you to perform collision detection at the pixel level. You only need to create a mask and then use its overlap()
method to accurately detect the actual overlapping area between two images. Through this method, you can implement more precise collision detection logic.
Animation Handling
Of course, besides collision detection, animation handling is also a very crucial part of game development. Implementing smooth 2D animations in Python is not difficult, we can use Pygame's pygame.time.Clock()
to control the game frame rate.
You can create an animation class to manage different animation frames. Then update the current frame based on time in the game loop, and you can easily achieve animation effects. As for displaying animation frames, you only need to use pygame.image.load()
to load images and use the blit()
method to draw them on the screen.
Game State Management
Another very important concept is game state management. You can create a state manager class to maintain and switch between different game states, such as main menu, game in progress, game over, etc.
Each state can be a separate class, responsible for handling its own update and rendering logic. Through this design, you can easily manage different states of the game. I think this technique is very useful and can greatly improve the readability and maintainability of game code.
Performance Optimization
Finally, let's talk about performance optimization techniques in Python game development. First, you can use Pygame's pygame.sprite.Group()
to efficiently manage sprites, thereby reducing unnecessary collision detection calculations.
Secondly, you can improve rendering efficiency by reducing the screen update area, only updating the parts that need to be redrawn. This can greatly reduce the pressure on CPU and GPU.
If you have very high performance requirements, don't worry, because you can completely use Cython or PyPy to accelerate performance-critical code segments. Cython can compile Python code into C extension modules, while PyPy improves execution efficiency through JIT compilation.
Lastly, I want to remind you to avoid executing some expensive operations in the game loop, such as frequent memory allocation and file IO, as these can seriously slow down the game running speed.
Summary
Overall, although Python is not as mainstream in the game development field as C++ or Java, its unique advantages and powerful ecosystem also make it very promising in this field. I believe that through today's sharing, you have gained an initial understanding of Python game development.
If you have further interest and questions about this, feel free to continue the discussion in the comments! After all, this is just an appetizer, and there's much more to Python game development than this. Let's walk hand in hand on this path and explore more interesting possibilities together!
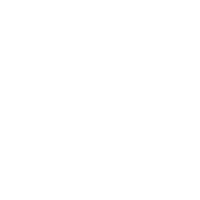
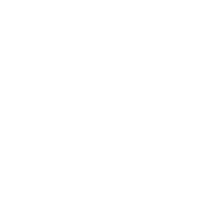