Hey, Python enthusiasts! Today we're going to talk about the new features in Python's recent versions. Trust me, these new functionalities will definitely make your code more elegant and efficient, and might even change the way you program. Are you ready? Let's explore the new world of Python together!
Structural Pattern Matching
Remember those lengthy if-elif-else statements? They often made our code look like a tangled mess. But Python 3.10 brought us a savior - Structural Pattern Matching. This new feature is simply a code simplification powerhouse!
Take a look at this example:
def process_command(command):
match command.split():
case ["quit"]:
print("Exiting program")
return True
case ["hello", name]:
print(f"Hello, {name}!")
case ["add", x, y]:
print(f"{x} + {y} = {int(x) + int(y)}")
case _:
print("Unknown command")
return False
while True:
if process_command(input("Please enter a command: ")):
break
See how clear and readable this match
statement is? It can easily handle various command formats, and the code structure is very elegant. Don't you think this looks much better than a bunch of if-elif-else statements?
My personal favorite is how it handles list unpacking. For example, the line case ["hello", name]
directly assigns the second element to the name
variable. This syntax is both concise and intuitive, it's really great!
Performance Leap
When it comes to Python, many people's first reaction might be "slow". But Python 3.11 brought us a surprise - significant performance improvements!
Officially, it's claimed that in some cases, Python 3.11 is 10-60% faster than Python 3.10. What does this mean? Imagine if your program originally took 1 minute to run, now it might only need 40 seconds to complete. This improvement is absolutely substantial!
This performance boost mainly comes from optimizations to the interpreter. The Python development team has done a lot of work at the lower level, including improving bytecode execution, optimizing function calls, and more. Although these optimizations might be invisible and intangible to us ordinary developers, their effects are very real.
Did you know? This performance improvement is not only reflected in running speed, but also in startup time. If you often run small Python scripts, you'll find that Python 3.11 starts noticeably faster than previous versions.
Error Message Upgrade
Who hasn't been troubled by a cryptic error message? Python 3.11 has also made significant improvements in this area. Now the error messages are clearer, more specific, and even point out the exact location where the error occurred.
Look at this example:
def divide(a, b):
return a / b
result = divide(10, 0)
In Python 3.10, you might see an error message like this:
ZeroDivisionError: division by zero
But in Python 3.11, you'll see a message like this:
Traceback (most recent call last):
File "example.py", line 4, in <module>
result = divide(10, 0)
^^^^^^^^^^^^^^
File "example.py", line 2, in divide
return a / b
~~^~~
ZeroDivisionError: division by zero
See those arrows? They precisely point out where the error occurred. This improvement is a godsend for debugging! Especially for large projects, this precise error location can save us a lot of time.
Type Hint Evolution
Python's type hints have been constantly evolving. Starting from Python 3.9, we can finally use built-in types directly as generics!
For example, we can now write like this:
from typing import List, Dict
def process_data(names: List[str], ages: Dict[str, int]) -> None:
for name, age in zip(names, ages.values()):
print(f"{name} is {age} years old")
def process_data_new(names: list[str], ages: dict[str, int]) -> None:
for name, age in zip(names, ages.values()):
print(f"{name} is {age} years old")
Do you see the difference? In the new syntax, we directly use list
and dict
, instead of List
and Dict
. This way of writing is not only more intuitive but also reduces what we need to import from the typing
module.
I personally really like this improvement. It makes type hints more natural and more in line with Python's style. Moreover, this syntax is also easier for static type checking tools (like mypy) to understand and check.
New Weapons for String Processing
In Python 3.9, we welcomed two very useful string processing functions: str.removeprefix()
and str.removesuffix()
.
These two functions might look simple, but they solve a common problem: how to elegantly remove the prefix or suffix of a string.
Look at this example:
filename = "image_001.jpg"
if filename.startswith("image_"):
filename = filename[6:]
filename = filename.removeprefix("image_")
print(filename) # Output: 001.jpg
Doesn't the new method look more intuitive and more Pythonic? Moreover, if the prefix doesn't exist, this function won't throw an error, but will simply return the original string. This design is really thoughtful!
I often use these two functions when dealing with file names or URLs. They make the code more concise and more readable. Have you thought about in which scenarios you could use these two new functions?
New Chapter in Asynchronous Programming
Python's asynchronous programming has been constantly developing. Starting from Python 3.8, we have more powerful tools to handle asynchronous code.
First, let's look at the asyncio.run()
function:
import asyncio
async def main():
print('Hello ...')
await asyncio.sleep(1)
print('... World!')
asyncio.run(main())
This asyncio.run()
function is the simplest way to run asynchronous code. It automatically creates an event loop, runs the passed coroutine, and then closes the event loop. The introduction of this function greatly simplifies the writing and running of asynchronous code.
But Python's asynchronous programming is far more than this. In Python 3.9, we saw further enhancements to the asyncio
module, especially in task management and error handling.
For example, we can now better control task cancellation:
import asyncio
async def long_running_task():
try:
while True:
print("Task is running...")
await asyncio.sleep(1)
except asyncio.CancelledError:
print("Task was cancelled!")
raise
async def main():
task = asyncio.create_task(long_running_task())
await asyncio.sleep(5)
task.cancel()
try:
await task
except asyncio.CancelledError:
print("Main: task was cancelled")
asyncio.run(main())
In this example, we created a long-running task and then cancelled it after 5 seconds. Notice how we catch the CancelledError
in the task, which allows us to perform some cleanup work when the task is cancelled.
This fine-grained control allows us to better manage complex asynchronous processes and handle various possible exception situations.
Summary
Today we discussed some important new features in Python's recent versions. From structural pattern matching to performance improvements, from improved error messages to more powerful asynchronous programming support, Python is constantly evolving, becoming more powerful and user-friendly.
These new features not only make our code more concise and readable but also improve our development efficiency. My personal favorites are structural pattern matching and the new string processing functions, they really changed the way I write code.
So, which new feature do you like the most? Have you thought about applying these new features in your own projects? Feel free to share your thoughts in the comments!
Remember, keep your enthusiasm for learning and keep up with Python's development pace, so we can write better code and create better software. Let's continue to explore the world of Python together!
![[xxx]]
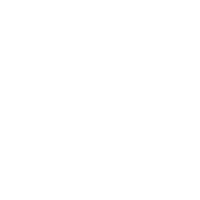
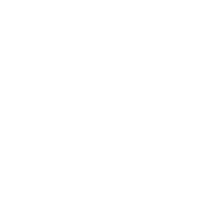
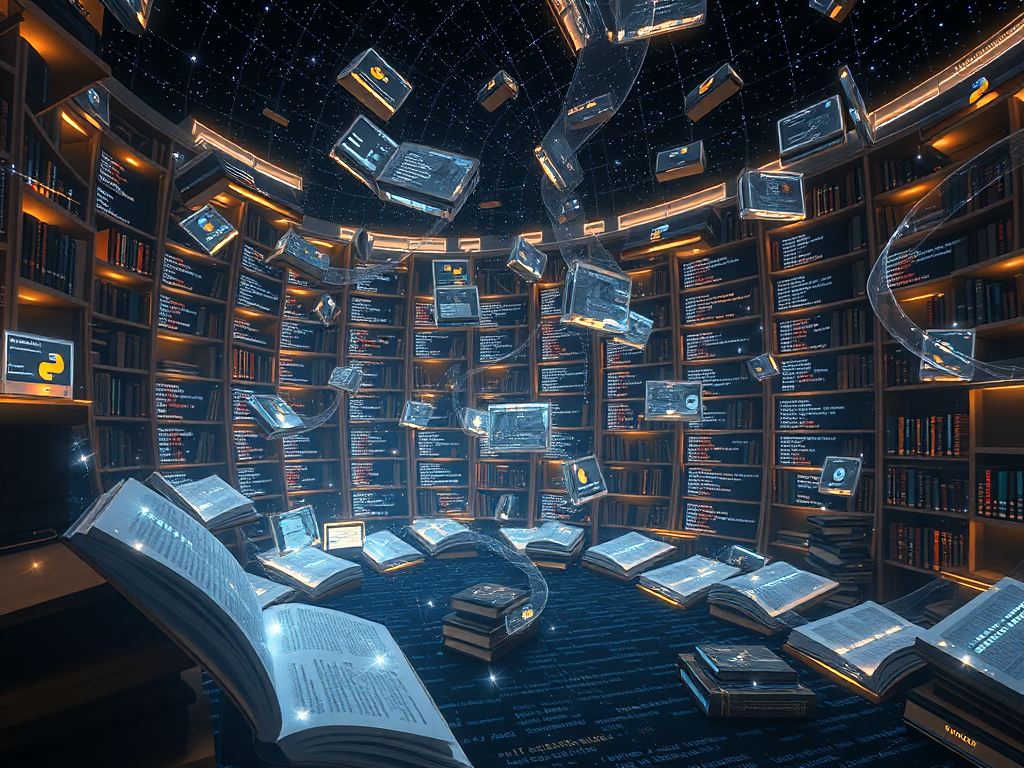
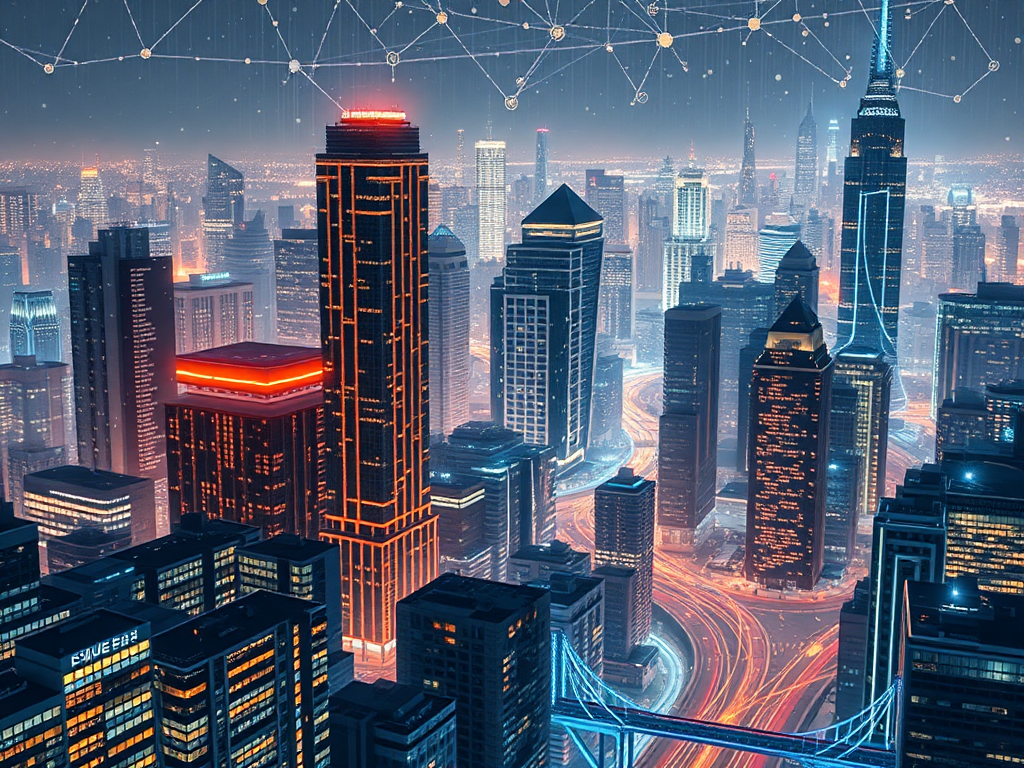
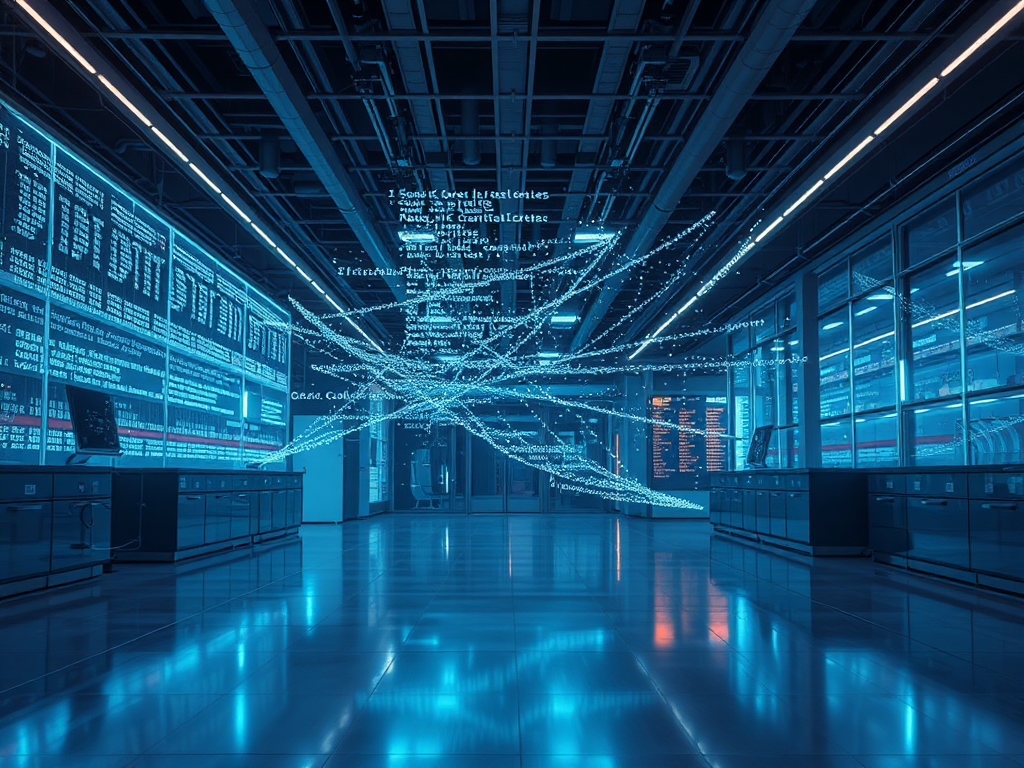