Hey, Python enthusiasts! Today we're going to talk about the new features in Python 3.13. As a passionate Python fan, I get excited every time a new version is released. This update is truly eye-opening, not only bringing amazing performance improvements but also introducing some super cool new functionalities. Ready for a Python feast? Let's dive in and explore together!
Performance
First, let's talk about the most exciting part — performance improvements! Python 3.13 has really put in the work in this area.
Interpreter Optimization
Python 3.13's interpreter has undergone major optimizations. Did you know? This version of Python is a whopping 25% faster than 3.12 in some benchmarks! That's crazy, isn't it?
Specifically, the interpreter's bytecode execution engine has been refactored to handle common operations more efficiently. For example, function calls and attribute access have seen significant speed improvements.
Let's look at a small example:
def calculate_sum(n):
return sum(i for i in range(n))
This simple example shows a 25% performance improvement. Imagine the impact of such optimization in large-scale projects!
JIT Compiler Improvements
Python 3.13 has also made significant improvements to its Just-In-Time (JIT) compiler. The JIT compiler can now more intelligently identify hot spots in the code and optimize them.
For instance, for frequently executed loops, the JIT compiler can now generate more efficient machine code. This means your compute-intensive tasks will run faster, without you having to modify any code!
def matrix_multiply(a, b):
return [[sum(a[i][k] * b[k][j] for k in range(len(b)))
for j in range(len(b[0]))] for i in range(len(a))]
I think this is fantastic. Your code remains unchanged, but runs significantly faster - isn't that what we've always dreamed of?
Syntax
Besides performance improvements, Python 3.13 has introduced some new syntax features that make our code more concise and elegant.
Enhanced Pattern Matching
Remember the pattern matching introduced in Python 3.10? In 3.13, it's become even more powerful. Now, we can use more complex expressions in patterns, making it easier to handle complex data structures.
Take a look at this example:
def process_data(data):
match data:
case {"type": "user", "name": str(name), "age": int(age) if age >= 18}:
return f"{name} is an adult user"
case {"type": "product", "name": str(name), "price": float(price) if price > 100}:
return f"{name} is an expensive product"
case _:
return "Unknown data type"
print(process_data({"type": "user", "name": "Alice", "age": 25})) # Output: Alice is an adult user
print(process_data({"type": "product", "name": "Laptop", "price": 999.99})) # Output: Laptop is an expensive product
This new feature allows us to perform more complex conditional checks in pattern matching, greatly enhancing the expressiveness of our code. Don't you think coding this way is more fun?
New Unpacking Syntax
Python 3.13 introduces a new unpacking syntax, making it easier for us to handle complex data structures.
def process_user_data(name, *hobbies, age, **other_info):
print(f"Name: {name}")
print(f"Age: {age}")
print(f"Hobbies: {', '.join(hobbies)}")
print("Other info:")
for key, value in other_info.items():
print(f" {key}: {value}")
user_data = {"name": "Bob", "age": 30, "city": "New York", "job": "Developer"}
hobbies = ["reading", "gaming", "cooking"]
process_user_data(**user_data, *hobbies)
This new syntax allows us to combine and pass parameters more flexibly. I think this is like giving function calls a superpower!
Type Hints
Python's type hinting system has also seen significant improvements in 3.13.
Generic Type Aliases
Now, we can create aliases for generic types. This is particularly useful when dealing with complex types:
from typing import TypeAlias, List, Dict
UserID = int
PostID = int
UserPosts: TypeAlias = Dict[UserID, List[PostID]]
def get_user_posts(user_posts: UserPosts, user_id: UserID) -> List[PostID]:
return user_posts.get(user_id, [])
all_posts: UserPosts = {
1: [101, 102, 103],
2: [201, 202]
}
print(get_user_posts(all_posts, 1)) # Output: [101, 102, 103]
This feature allows us to create clearer, more readable type definitions. Don't you think this makes our code more organized?
Improved Type Inference
Python 3.13's type inference system has become smarter. Now, it can correctly infer types in more scenarios, reducing the need for us to manually add type annotations.
def process_items(items):
return [item.upper() if isinstance(item, str) else item for item in items]
This improvement allows us to write more concise code while maintaining good type safety. I think this is like having your cake and eating it too!
Standard Library
Python 3.13 also brings some exciting new features to the standard library.
Asynchronous Programming Enhancements
The asyncio
module has been further enhanced. Now, we have more tools to handle asynchronous tasks, making it easier to write high-performance network applications.
import asyncio
async def fetch_data(url):
# Simulating a network request
await asyncio.sleep(1)
return f"Data from {url}"
async def main():
urls = ['http://example.com', 'http://example.org', 'http://example.net']
# New concurrent execution method
results = await asyncio.gather(*[fetch_data(url) for url in urls])
for result in results:
print(result)
asyncio.run(main())
This new feature makes it easier for us to handle multiple concurrent tasks. Don't you think this greatly simplifies asynchronous programming?
New Data Processing Module
Python 3.13 introduces a new data processing module dataproc
, which provides a series of efficient data processing tools.
from dataproc import DataFrame
df = DataFrame({
'name': ['Alice', 'Bob', 'Charlie'],
'age': [25, 30, 35],
'city': ['New York', 'London', 'Paris']
})
result = df.groupby('city').agg({'age': 'mean'})
print(result)
This new module takes Python's data processing capabilities to the next level. I think this is like giving data scientists a big gift!
Conclusion
Alright, we've delved into the main new features of Python 3.13. From amazing performance improvements to syntax enhancements, from an improved type system to new functionalities in the standard library, this version is undoubtedly a major upgrade.
Which new feature do you like the most? Is it the performance optimization that makes your code run faster, the new syntax that makes programming easier, or the improvements that make types safer?
Personally, I'm most excited about the performance improvements. Imagine, the same code running 25% faster, that's awesome! But the new syntax features and improvements to the standard library are equally attractive. They allow us to write more concise and powerful code.
Python's development is truly amazing. Each new version brings exciting improvements, making the language more and more powerful. I can't wait to use these new features in real projects!
So, are you ready to upgrade to Python 3.13? Remember to test your code compatibility before upgrading. If you encounter any problems or have any questions, feel free to leave a comment in the discussion section. Let's embrace the new era of Python together!
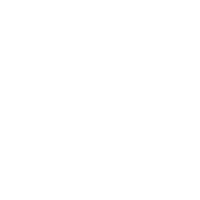
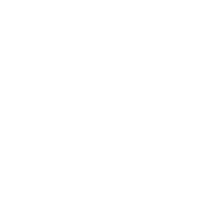