Have you heard? Python 3.12 has been released! This version brings quite a few exciting new features. Today, let's talk about these new functionalities and see how they can make our Python programming experience even better.
Performance Improvements
First, let's talk about performance. Python 3.12 has seen significant performance improvements again. Although not as impressive as the 10-60% boost in 3.11, 3.12 still has about a 5% performance improvement compared to 3.11. What does this mean? Simply put, your code will run faster!
For example, suppose you have a script that processes a large amount of data and takes 100 seconds to run on Python 3.11. Now, on Python 3.12, the same script might only take about 95 seconds to complete. It may not seem like much, but when you need to run this script frequently, this 5-second difference will accumulate into considerable time savings.
This performance improvement mainly comes from optimizations in the Python interpreter. The development team has made several improvements to CPython (the official implementation of Python), including optimized bytecode execution and improved memory management. While these improvements might seem a bit esoteric to us ordinary developers, the result is tangible - our code runs faster.
Syntactic Sugar
Next, let's look at some syntactic improvements in Python 3.12. These improvements may seem small, but they can make our code more concise and readable.
New Tricks with f-strings
Remember f-strings? They allow us to conveniently insert variables into strings. Now, Python 3.12 has made f-strings even more powerful.
Previously, if we wanted to use quotes in an f-string, we might encounter some trouble. For example:
name = "Alice"
greeting = f"She said, "Hello, {name}!"" # This would cause an error
Now, we can write it like this:
name = "Alice"
greeting = f"She said, "Hello, {name}!"" # This is fine now
Doesn't seem like a big deal? But when you need to handle a large number of strings containing quotes, this improvement will make your code clearer and more readable.
Improvements in Type Annotations
Python 3.12 has also improved the syntax for type annotations. Now, we can use |
in type annotations to represent union types, without needing to import Union
from the typing
module.
For instance, we might have written this before:
from typing import Union
def greet(name: Union[str, None]) -> str:
if name is None:
return "Hello, stranger!"
return f"Hello, {name}!"
Now, we can simplify it to:
def greet(name: str | None) -> str:
if name is None:
return "Hello, stranger!"
return f"Hello, {name}!"
Doesn't the code feel more concise? This improvement not only makes the code more readable but also reduces our dependency on the typing
module.
New Standard Library Modules
Python 3.12 also brings us some new standard library modules, allowing us to handle some common tasks more conveniently.
New Tool for Asynchronous Programming: asyncio.TaskGroup
If you've done asynchronous programming, you might know that managing multiple coroutine tasks can be a bit tricky. Now, Python 3.12 introduces asyncio.TaskGroup
, a new feature that makes it easier for us to manage a group of related asynchronous tasks.
Let me demonstrate:
import asyncio
async def fetch_data(url):
# Assume this is an asynchronous network request
await asyncio.sleep(1)
return f"Data from {url}"
async def main():
async with asyncio.TaskGroup() as tg:
task1 = tg.create_task(fetch_data("https://api1.example.com"))
task2 = tg.create_task(fetch_data("https://api2.example.com"))
task3 = tg.create_task(fetch_data("https://api3.example.com"))
results = [task1.result(), task2.result(), task3.result()]
print(results)
asyncio.run(main())
In this example, we create three asynchronous tasks that will execute concurrently. TaskGroup
will wait for all tasks to complete, and if any of the tasks fail, it will cancel the other running tasks. This approach makes it easier for us to handle concurrent tasks while also providing better error handling mechanisms.
More Powerful Regular Expressions: regex Module
Python's re
module has always been a powerful tool for handling text pattern matching, but it also has some limitations. Python 3.12 introduces the new regex
module, which provides more advanced features, such as support for Unicode properties, conditional pattern matching, and more.
Let's look at an example:
import regex
text = "The quick brown fox jumps over the lazy dog."
pattern = r"\b\p{Lu}\w+\b"
matches = regex.findall(pattern, text)
print(matches) # Output: ['The']
In this example, \p{Lu}
matches any uppercase letter, which is an example of a Unicode property. This kind of pattern is particularly useful when dealing with multilingual text.
Security Enhancements
Python 3.12 has also made some improvements in terms of security. One notable change is the introduction of a new secure random number generator.
Previously, we might generate random numbers like this:
import random
secret_key = ''.join(random.choices('0123456789ABCDEF', k=16))
While this method is fine in most cases, it might not be secure enough in scenarios requiring high security (such as generating encryption keys).
Now, Python 3.12 introduces the secrets.randbytes()
function, which uses the operating system's random source to generate truly random bytes:
import secrets
secret_key = secrets.randbytes(16).hex()
This method generates more secure random numbers, suitable for cryptography-related applications.
Summary
Python 3.12 brings many exciting new features. From performance improvements to syntax enhancements, from new standard library modules to security improvements, this version has made progress in multiple aspects. These improvements not only make our code run faster but also make our programming experience more enjoyable.
Which of these new features do you find most attractive? Is it the performance boost, the new syntactic sugar, or those powerful new modules? Feel free to share your thoughts in the comments section.
Remember, the joy of programming lies not only in solving problems but also in continuously learning and trying new things. So, update to Python 3.12 and start exploring these new features! You'll find that Python programming has become even more fun and efficient.
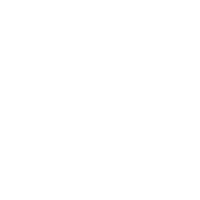
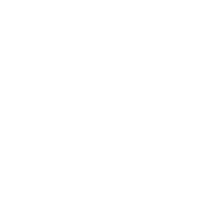