Hello, Python enthusiasts! Today let's talk about an exciting new feature introduced in Python 3.10 - Structural Pattern Matching. This feature not only makes our code more elegant but also greatly improves its readability and maintainability. So, what exactly is it, and what conveniences does it bring us? Let's explore!
Introduction to Pattern Matching
Remember the if-elif-else structure we often use in programming? While practical, it can become lengthy and hard to read when dealing with complex logic. Structural Pattern Matching is like an upgraded version of if-elif-else, allowing us to handle complex conditional logic in a more concise and intuitive way.
Here's a simple example:
def greet(name):
match name:
case "Alice":
return "Hi Alice, how are you?"
case "Bob":
return "Hello Bob, nice to see you!"
case _:
return f"Hey {name}, welcome!"
print(greet("Alice")) # Output: Hi Alice, how are you?
print(greet("Charlie")) # Output: Hey Charlie, welcome!
See? With the match
and case
keywords, we can return appropriate greetings based on different input values. Isn't this much more concise than a series of if-elif statements?
Understanding Pattern Matching
The power of pattern matching lies not only in its ability to match simple values but also in matching complex data structures. Let's look at some more advanced usages:
1. Sequence Matching
def analyze_list(data):
match data:
case []:
return "Empty list"
case [x]:
return f"Single element list: {x}"
case [x, y]:
return f"Two element list: {x} and {y}"
case [x, *rest]:
return f"List with {len(rest) + 1} elements, starting with {x}"
print(analyze_list([])) # Output: Empty list
print(analyze_list([1, 2, 3, 4])) # Output: List with 4 elements, starting with 1
This example shows how to match lists of different lengths. Doesn't it feel more elegant than writing a bunch of if len(data) == ...
?
2. Class Matching
class Point:
def __init__(self, x, y):
self.x = x
self.y = y
def classify_point(point):
match point:
case Point(x=0, y=0):
return "Origin"
case Point(x=0, y=y):
return f"On Y-axis at y={y}"
case Point(x=x, y=0):
return f"On X-axis at x={x}"
case Point():
return f"Point at ({point.x}, {point.y})"
case _:
return "Not a point"
print(classify_point(Point(0, 0))) # Output: Origin
print(classify_point(Point(5, 0))) # Output: On X-axis at x=5
This example shows how to match class instances and extract their attributes. You see, we can even access object properties directly during matching. Isn't that convenient?
3. Or Patterns
def describe_number(x):
match x:
case 0 | 1 | 2:
return "Small number"
case int(n) if n > 100:
return "Big number"
case float():
return "Floating point number"
case _:
return "Something else"
print(describe_number(1)) # Output: Small number
print(describe_number(200)) # Output: Big number
print(describe_number(3.14)) # Output: Floating point number
This example shows how to use |
to match multiple possible values and how to use guard conditions (if n > 100
) for more complex matching.
Practical Applications
So, what benefits can this new feature bring in actual programming? Let's look at some specific application scenarios:
1. Command Line Argument Parsing
Suppose we're developing a command line tool that needs to parse different commands and arguments. Using pattern matching, we can do this:
def process_command(command):
match command.split():
case ["quit"]:
return "Exiting program"
case ["create", filename]:
return f"Creating file: {filename}"
case ["delete", filename]:
return f"Deleting file: {filename}"
case ["move", source, destination]:
return f"Moving {source} to {destination}"
case ["help"]:
return "Available commands: quit, create, delete, move, help"
case _:
return "Unknown command. Type 'help' for assistance."
print(process_command("create myfile.txt")) # Output: Creating file: myfile.txt
print(process_command("move old.txt new.txt")) # Output: Moving old.txt to new.txt
print(process_command("invalid")) # Output: Unknown command. Type 'help' for assistance.
Isn't this method clearer than traditional string parsing and conditional judgment?
2. State Machine Implementation
Pattern matching is also very useful when implementing state machines. For example, suppose we're implementing a simple traffic light controller:
class TrafficLight:
def __init__(self):
self.state = "RED"
def change(self):
match self.state:
case "RED":
self.state = "GREEN"
case "GREEN":
self.state = "YELLOW"
case "YELLOW":
self.state = "RED"
def __str__(self):
return f"Traffic light is {self.state}"
light = TrafficLight()
print(light) # Output: Traffic light is RED
light.change()
print(light) # Output: Traffic light is GREEN
light.change()
print(light) # Output: Traffic light is YELLOW
This implementation is much more concise and clear than using if-elif statements, and it's easier to extend and modify.
3. Data Parsing and Validation
When handling complex data structures, pattern matching can also be very useful. For example, suppose we need to parse and validate a dictionary containing user information:
def validate_user_data(data):
match data:
case {"name": str(name), "age": int(age), "email": str(email)}:
if age < 0 or age > 120:
return "Invalid age"
if "@" not in email:
return "Invalid email format"
return f"Valid user: {name}, {age} years old, email: {email}"
case {"name": str(name), "age": int(age)}:
return "Missing email"
case {"name": str(name), "email": str(email)}:
return "Missing age"
case _:
return "Invalid data format"
print(validate_user_data({"name": "Alice", "age": 30, "email": "[email protected]"}))
print(validate_user_data({"name": "Bob", "age": 25}))
print(validate_user_data({"name": "Charlie", "age": 150, "email": "[email protected]"}))
This method not only allows easy checking of the dictionary structure but also performs type checking and data extraction during matching.
Considerations
While structural pattern matching is powerful, there are some considerations when using it:
-
Performance Consideration: For simple conditional judgments, the traditional if-elif structure might be slightly better in performance. Pattern matching is more suited for handling complex structures and multi-branch logic.
-
Readability Balance: Although pattern matching can make code more concise, overusing complex patterns may reduce readability. Balance its use based on the specific situation.
-
Backward Compatibility: If your project needs to support versions before Python 3.10, you can't use this new feature.
-
Pattern Order: Pattern matching is done in order, so more specific patterns should be placed first, or they may never be matched.
Conclusion
Structural Pattern Matching is undoubtedly one of the most exciting new features in Python 3.10. It allows us to handle complex logic in a more elegant and Pythonic way, improving code readability and maintainability.
From simple value matching to complex data structure parsing, from command line argument processing to state machine implementation, pattern matching can shine. It makes our code more concise and intuitive while maintaining flexibility.
Of course, like all programming tools, pattern matching is not a silver bullet. We need to decide whether to use it based on the specific scenario. But there's no doubt it provides us with a powerful new tool that can greatly simplify our code in many situations.
Are you ready to try using structural pattern matching in your next project? Trust me, once you start using it, you'll love this new feature!
Let's embrace Python's new features and write more elegant and efficient code!
What are your thoughts on structural pattern matching? Feel free to share your ideas and experiences in the comments. If you have any questions, feel free to ask, and we can discuss and learn together.
Happy coding!
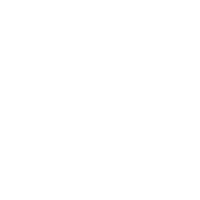
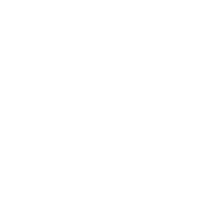
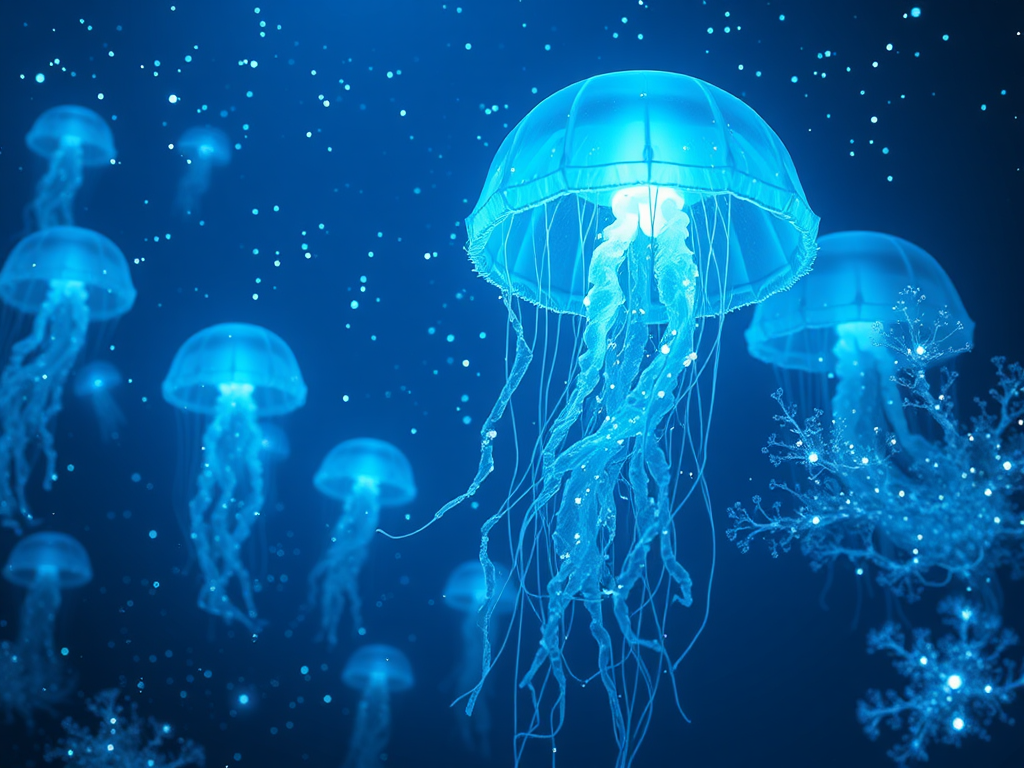
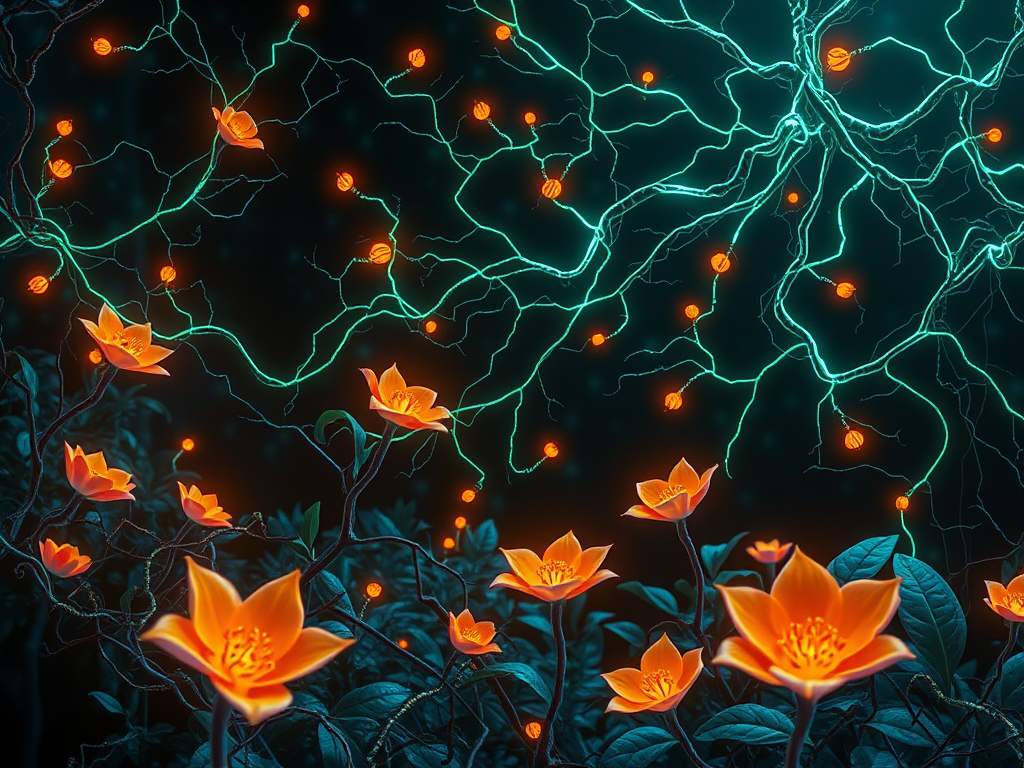
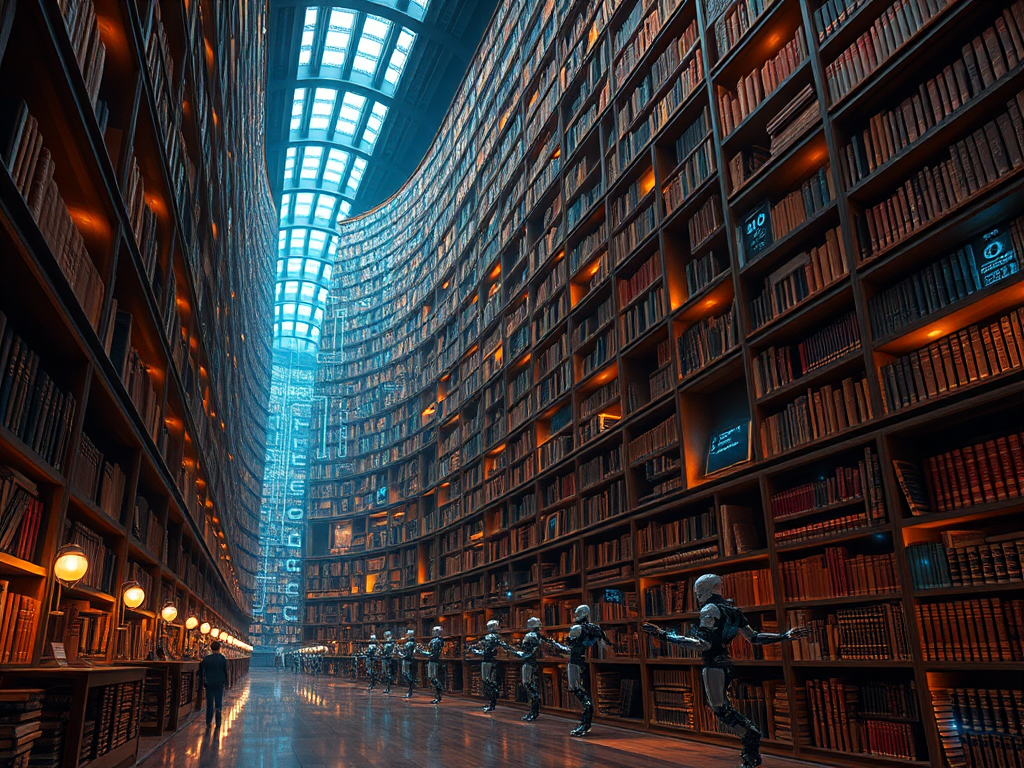