Hello, Python enthusiasts! Today, let's talk about the major updates in the recent Python versions. From 3.10 to 3.12, Python has made rapid progress, not just syntactically but also with significant performance improvements. These new features make our code simpler, faster, and help us write more powerful and reliable programs. Let's take a look at these exciting changes!
The Beauty of Syntax
The Magic of Parentheses
Remember the excitement when you first used the with
statement? Python 3.10 has introduced a small but very practical improvement—allowing the use of outer parentheses to write multiple context managers. This seemingly trivial change greatly improves code readability.
with (
open('file1.txt') as f1,
open('file2.txt') as f2
):
data1 = f1.read()
data2 = f2.read()
Doesn't this code look refreshing? Compared to the previous way, this method makes using multiple context managers clearer and neater. I personally love this improvement because it perfectly embodies Python's philosophy that "elegance is better than ugliness."
Simplified Union Types
Type hints have always been a hot topic in the Python community. Some love them, some hate them. But there's no denying that type hints help us write more robust code. Python 3.10 takes a big step forward by introducing a simpler way to represent union types.
def handle_data(data: int | str) -> None:
print(data)
Doesn't this new syntax look comfortable? Compared to the previous way using Union
, this is more intuitive and concise. I think this improvement is fantastic, as it not only makes the code more readable but also allows us to handle different data types more flexibly.
The Power of Pattern Matching
Speaking of new features in Python 3.10, how can we not mention pattern matching? Introduced in Python 3.10, this feature has been further refined in subsequent versions. Check out this example:
match command:
case 'start':
print("Starting...")
case 'stop':
print("Stopping...")
case _:
print("Unknown command")
Doesn't this remind you of the switch
statement in other languages? But Python's pattern matching is far more powerful than a simple switch
. It can match simple values and perform structured matching, which is particularly useful when dealing with complex data structures.
Personally, I think the introduction of pattern matching is one of the most important syntax improvements in Python in recent years. It allows us to handle complex conditional logic in a more elegant and Pythonic way. If you haven't tried it yet, I strongly recommend giving it a go in your next project!
The Speed of Performance
Lightning Fast
When people think of Python, many immediately think "easy to use, but average performance." However, Python 3.11 has dealt a heavy blow to that notion! The performance improvements in this version are astonishing, with many common operations seeing a 10% to 60% speed increase.
Imagine your program suddenly becoming twice as fast—exciting, right? This isn't a dream; it's the reality brought by Python 3.11. Especially in simple mathematical calculations and string operations, you'll noticeably feel the speed boost.
I remember the first time I ran a program on Python 3.11, that lightning-fast feeling was truly thrilling. If you haven't upgraded to 3.11 yet, I suggest you try it out. Trust me, you'll love the speed!
The Art of Memory Management
Performance optimization isn't just about speed; memory efficiency is equally important. Python 3.12 has made new breakthroughs in this area. It has optimized memory management and garbage collection, reducing memory usage and improving overall performance.
What does this mean? Simply put, your program not only runs faster but also utilizes system resources more efficiently. For programs that need to handle large amounts of data or run for long periods, this is a significant boon.
I personally believe these optimizations reflect the Python development team's continuous focus on language performance. They may not be as eye-catching as new syntax features, but they are crucial for enhancing Python's performance in practical applications.
The Wisdom of Error Handling
Clearer Error Messages
As someone who frequently works with Python, I must say the improvements in error handling in Python 3.10 and 3.11 are truly eye-opening. Especially with syntax errors, the new versions provide clearer and more specific error messages.
For example, look at this:
expected = {9: 1, 18: 2, 19: 2, 27: 3, 28: 3, 29: 3, 36: 4, 37: 4,
SyntaxError: '{' was never closed
Doesn't this way of pinpointing the error location make debugging much easier? I remember in the past, I often had to carefully check the entire code block to find similar issues. Now, Python directly tells you where the error is—this experience is fantastic!
The Usefulness of Exception Groups
Python 3.11 also brings us the new feature of exception groups. This feature allows us to catch multiple exceptions in a single except
block, greatly simplifying error handling code.
try:
# Code that may raise multiple exceptions
pass
except (TypeError, ValueError) as e:
print(f"Handling exception: {e}")
This not only makes the code more concise but also helps us better organize and manage various potential exceptions. I find this feature especially useful for complex programs that need to handle multiple possible errors.
Richer Context
Python 3.11's improvements in error messages don't stop there. It provides more context information to help developers locate issues faster. For example:
def foo(x):
return x + 1
foo("string") # Will prompt a type error
In this example, Python not only tells you a type error occurred but also provides more context about where the error happened. This detailed error reporting is invaluable for quickly locating and resolving issues.
I believe these improvements in error handling and debugging highlight Python's focus on developer experience. They may seem like small changes, but they can greatly improve our efficiency in everyday programming.
The Strength of the Type System
More Powerful Type Hints
Python's type system has been continually evolving, and Python 3.12 has made new breakthroughs in this area. It supports more complex type combinations, allowing us to describe our code more precisely.
Check out this example:
from typing import Literal
def handle_status(status: Literal['success', 'failure']) -> None:
print(f"Status: {status}")
Using Literal
allows us to precisely specify allowed values, which is especially useful in API design and code documentation. I think this feature is fantastic because it not only improves code readability but also helps us catch potential errors during development.
Progress in Type Checking
As the type system continues to improve, Python's static type checking tools (like mypy) are becoming more powerful. This means we can catch more potential issues before runtime, greatly enhancing code quality and reliability.
I believe that although Python is still a dynamically typed language, these improvements in the type system provide great convenience for projects that require stricter type checks. It allows Python to maintain flexibility while also meeting higher code quality requirements.
Looking Ahead
After seeing these exciting new features, are you as excited about Python's future as I am? From syntax optimization to performance enhancement, error handling improvements to type system strengthening, Python is evolving at an amazing pace.
However, while new features are great, we also need to be mindful of some practical issues in their usage. For example, if your project needs to be compatible with multiple Python versions, you should be cautious about using these new features. Additionally, some third-party libraries may need time to adapt to changes in new versions.
So, how should we respond to these changes? My suggestions are:
- Keep the passion for learning. Python evolves rapidly, and we need to continually learn new knowledge.
- Gradually introduce new features into projects. Don't rush to use all the new features at once; instead, adopt them gradually based on project needs.
- Stay informed about community dynamics. The Python community is very active, with frequent discussions and best practices regarding new features.
What do you think about these new features? Feel free to share your thoughts in the comments! Let's explore Python's future together and grow and progress together.
Remember, the joy of programming is not only in solving problems but also in continuously learning and trying new things. So, are you ready to embrace the new era of Python? Let's move forward together on this exciting journey!
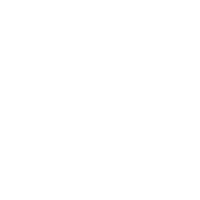
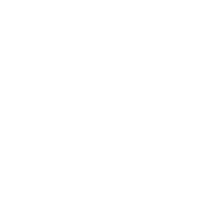