Hey, Python enthusiasts! Today, let's talk about the new features in recent Python versions. As a Python developer, are you often confused by all the new versions? Don't worry, let's explore these exciting new features together!
Structural Pattern Matching
Remember the excitement when you first encountered the switch-case statement? Python 3.10 brings us the more powerful Structural Pattern Matching. This feature is like an upgraded version of the switch statement!
Let's look at an example:
def http_error(status):
match status:
case 400:
return "Bad request"
case 404:
return "Not found"
case 418:
return "I'm a teapot"
case _:
return "Something's wrong with the internet"
print(http_error(418)) # Output: I'm a teapot
See that? This is much more concise than the traditional if-elif structure. And it's not just simple value matching, it also supports more complex patterns:
def process_command(command):
match command.split():
case ["quit"]:
print("Goodbye!")
exit()
case ["load", filename]:
print(f"Loading file: {filename}")
case ["save", filename]:
print(f"Saving file: {filename}")
case _:
print("Unknown command")
process_command("load data.txt") # Output: Loading file: data.txt
This feature is really great! It makes our code clearer and easier to understand. Don't you think this is much more elegant than a long string of if-elif statements?
Performance Leap
Speaking of Python 3.11, I have to mention its performance improvement. Officials claim speed increases of 10% to 60% in some cases. What does this mean? Imagine a task that originally took your program one minute to complete, now it might only take 30 seconds!
This improvement mainly comes from optimizations to the CPython interpreter. For example, it improved the execution speed of bytecode, optimized exception handling, and introduced faster calling conventions.
A friend of mine shared his experience. He had a script that processed large amounts of data, which took 15 minutes to run on Python 3.10. After upgrading to 3.11, the same script only took 9 minutes to complete. This is a revolution in efficiency!
Have you ever been frustrated by Python's slow running speed? Now, it's time to revisit those "slow" scripts. Maybe upgrading your Python version will bring you unexpected surprises.
New Dictionary Tricks
Looking further back, Python 3.9 brought us the dictionary merge operators |
and |=
. This feature might seem insignificant, but it really can make our code more concise.
Take a look at this example:
x = {"a": 1, "b": 2}
y = {"b": 3, "c": 4}
z = {**x, **y}
print(z) # Output: {'a': 1, 'b': 3, 'c': 4}
x = {"a": 1, "b": 2}
y = {"b": 3, "c": 4}
z = x | y
print(z) # Output: {'a': 1, 'b': 3, 'c': 4}
Doesn't the code feel cleaner all of a sudden? This feature is particularly useful in scenarios like configuration merging and data updating.
I remember once when dealing with a complex configuration system, I needed to merge multiple configuration dictionaries. The old code looked like a bunch of dict.update()
calls, now it can be done with a simple |
operator. This not only makes the code more readable but also reduces the possibility of errors.
The Walrus Has Arrived
The assignment expression (colloquially known as the "walrus operator" :=) introduced in Python 3.8 might be one of the most controversial features in recent years. But I have to say, it can indeed make code more concise in certain situations.
Look at this example:
n = len(a)
if n > 10:
print(f"List is too long ({n} elements, expected <= 10)")
if (n := len(a)) > 10:
print(f"List is too long ({n} elements, expected <= 10)")
This feature is particularly useful when dealing with files or iterators:
while chunk := file.read(8192):
process(chunk)
It might feel a bit strange when you first start using it, but once you get used to it, you'll find that it can make your code more concise in many scenarios.
However, I suggest using this feature cautiously. Overuse may lead to code that's difficult to understand. Remember, code readability is more important than conciseness.
Data Classes Have Arrived
Finally, let's talk about data classes introduced in Python 3.7. This feature is really hard to put down!
Look at this example:
from dataclasses import dataclass
@dataclass
class Point:
x: float
y: float
p = Point(1.5, 2.5)
print(p) # Output: Point(x=1.5, y=2.5)
Previously, we might have needed to write a bunch of __init__
, __repr__
methods to achieve the same functionality. Now, all of this is automatically generated with a simple decorator.
Data classes not only save us from boilerplate code, but they also provide comparison, hashing, and other functionalities, making it more convenient for us to use these classes.
I remember once when dealing with a complex configuration system, using data classes made the entire code structure much clearer. It not only reduced the amount of code but also improved the readability and maintainability of the code.
Summary
These new features of Python, each like adding a handy new tool to our toolbox. Structural pattern matching makes our code clearer, performance optimizations make our programs run faster, and new syntactic sugar makes our code more concise.
But remember, while new features are good, don't use new features just for the sake of using them. Code readability and maintainability are the most important.
Which new feature do you like the most? How has it changed your programming habits? Feel free to share your thoughts in the comments!
Finally, I want to say that Python's development is truly exciting. Each new version brings surprises, allowing us to write better and more efficient code. As Python developers, we are fortunate to personally experience the growth and evolution of this language.
So, are you ready to embrace these new features? Let's explore more possibilities in the world of Python together!
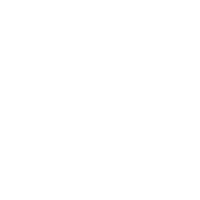
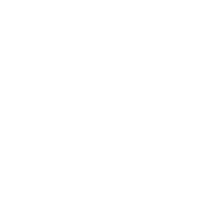