Preface
Have you ever felt frustrated by repeating the same DevOps tasks daily - manually deploying applications, configuring servers, checking logs - tasks that are not only tedious but also error-prone? In fact, through Python DevOps automation, we can delegate these repetitive tasks to programs. So, how exactly can Python help us achieve DevOps automation?
Fundamentals
When it comes to DevOps automation, Python is indispensable. With its elegant syntax and rich third-party libraries, Python has become a powerful tool for DevOps engineers. In my view, Python has become the preferred language for DevOps automation for several key reasons:
First is its simple syntax. Compared to other languages, Python has a gentler learning curve. I remember when I first started learning Python, I was able to write simple DevOps scripts in just a few days. For example, a script to traverse server directories:
import os
def list_files(path):
for root, dirs, files in os.walk(path):
for file in files:
print(os.path.join(root, file))
list_files('/var/log')
Second is its rich ecosystem. Python has numerous ready-to-use DevOps-related libraries, such as: - paramiko: for SSH connections and remote command execution - fabric: for automated deployment and system management - psutil: for obtaining system and process information - requests: for sending HTTP requests - ansible: for automated configuration management
These libraries greatly improve our development efficiency. Take paramiko for example, it makes remote command execution remarkably simple:
import paramiko
def execute_command(host, command):
client = paramiko.SSHClient()
client.set_missing_host_key_policy(paramiko.AutoAddPolicy())
client.connect(host, username='root', password='password')
stdin, stdout, stderr = client.exec_command(command)
print(stdout.read().decode())
client.close()
Advanced Topics
After mastering the basics, let's look at how to solve real DevOps problems using Python.
First is server monitoring. Using the psutil library, we can easily obtain server CPU, memory, disk, and other information:
import psutil
import time
from datetime import datetime
def monitor_resources():
while True:
cpu_percent = psutil.cpu_percent(interval=1)
memory = psutil.virtual_memory()
disk = psutil.disk_usage('/')
print(f"Time: {datetime.now().strftime('%Y-%m-%d %H:%M:%S')}")
print(f"CPU Usage: {cpu_percent}%")
print(f"Memory Usage: {memory.percent}%")
print(f"Disk Usage: {disk.percent}%")
print("-" * 50)
time.sleep(5)
This script outputs system resource usage every 5 seconds. In my practice, it has helped me identify multiple performance bottlenecks.
Next is automated deployment. Using the fabric library, we can easily implement multi-server application deployment:
from fabric import Connection
def deploy_application(hosts):
for host in hosts:
with Connection(host) as c:
# Update code
c.run('cd /app && git pull')
# Install dependencies
c.run('pip install -r requirements.txt')
# Restart service
c.run('systemctl restart myapp')
print(f"Deployment on {host} completed")
hosts = ['server1.example.com', 'server2.example.com']
deploy_application(hosts)
Practical Applications
In actual work, I find log analysis and alert systems are most commonly used. Here's a log analysis script I wrote:
import re
from collections import defaultdict
import smtplib
from email.mime.text import MIMEText
def analyze_log(log_file):
error_patterns = {
'oom': r'Out of memory',
'timeout': r'Connection timed out',
'error_500': r'HTTP 500'
}
errors = defaultdict(int)
with open(log_file, 'r') as f:
for line in f:
for error_type, pattern in error_patterns.items():
if re.search(pattern, line):
errors[error_type] += 1
return errors
def send_alert(errors):
if sum(errors.values()) > 100: # Error count exceeds threshold
msg = MIMEText(f"Large number of errors detected: {errors}")
msg['Subject'] = 'System Alert'
smtp = smtplib.SMTP('smtp.example.com')
smtp.send_message(msg)
smtp.quit()
errors = analyze_log('/var/log/app.log')
send_alert(errors)
This script analyzes error messages in log files and automatically sends email alerts when the error count exceeds a threshold. In production environments, it has helped us identify multiple potential system issues.
Finally, let's talk about configuration management. Using Python's yaml library, we can easily manage configuration files:
import yaml
import os
def update_config(env, changes):
config_file = f'config_{env}.yaml'
with open(config_file, 'r') as f:
config = yaml.safe_load(f)
# Update configuration
for key, value in changes.items():
config[key] = value
# Backup original configuration
os.rename(config_file, f'{config_file}.bak')
# Write new configuration
with open(config_file, 'w') as f:
yaml.dump(config, f)
changes = {
'database_url': 'mongodb://new-server:27017',
'cache_size': 1024
}
update_config('production', changes)
Lessons Learned
Through years of Python DevOps automation practice, I've summarized several key insights:
-
Scripts should be simple and reliable. The most important aspect of DevOps scripts is stability. It's better to write a few extra lines of code to ensure robustness.
-
Handle errors properly. In production environments, various unexpected situations can occur. Consider exceptional cases thoroughly and implement proper logging and error handling.
-
Focus on code reusability. Package commonly used functions into modules to avoid reinventing the wheel. For example, we can encapsulate common functionalities like server connections and configuration reading.
-
Keep scripts updated. As business evolves, DevOps scripts need continuous updates. Regularly check and update scripts to ensure they meet current requirements.
Future Outlook
Looking ahead, Python DevOps automation has significant room for development, particularly in these areas:
-
Containerized Operations. With the popularity of Docker and Kubernetes, using Python to better manage container environments will become an important topic.
-
Intelligent Operations. Combining machine learning to implement smarter monitoring and alert systems, predicting potential issues in advance.
-
Cloud-Native Support. With the development of cloud computing, better supporting multi-cloud environment management is also a direction worth attention.
What do you think DevOps work will look like in the future? Feel free to share your thoughts in the comments.
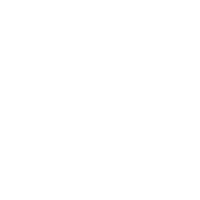