Hello, dear Python enthusiasts! Today, I want to share a very important topic—Python performance optimization. As a Python developer, have you ever been troubled by slow code execution? Do you want to know how to make your Python programs run faster? Don’t worry, this article will reveal the secrets of Python performance optimization!
The Source of Performance
First, let's talk about the root of Python performance. Python is an interpreted language, which means its code is executed line by line at runtime. This trait makes Python highly flexible and easy to use but also leads to slower execution speeds compared to compiled languages.
However, don't be discouraged! Python's dynamic typing and easy debugging make it very popular in rapid development environments. Moreover, with some optimization techniques, we can significantly enhance the performance of Python code.
Speaking of Python performance, we must mention the Global Interpreter Lock (GIL). GIL is a mechanism in the Python interpreter that ensures only one thread can execute Python bytecode at a time. This greatly impacts the performance of multithreaded programs, especially on multi-core systems. But don’t worry, we have ways to bypass this limitation!
Data Structures Matter
In Python, choosing the right data structure has a huge impact on performance. Let's look at some commonly used data structures:
-
List: Suitable for storing ordered data, great for iteration. However, it may not be efficient for frequent insertions and deletions.
-
Dictionary: Key-value structure with very fast lookup speed. If you need to frequently look up data, a dictionary is the best choice.
-
Set: Stores unique elements, ideal for deduplication and membership testing.
-
Tuple: Immutable sequence, ensures data is not accidentally modified.
Here's an example:
fruits = ['apple', 'banana', 'orange']
'apple' in fruits # O(n) time complexity
fruits_set = {'apple', 'banana', 'orange'}
'apple' in fruits_set # O(1) time complexity
See? Using a set for membership testing is much faster than using a list!
Moreover, for a large amount of numerical calculations, NumPy arrays are often more efficient than native Python lists. NumPy arrays have advantages in both memory usage and computation speed.
The Magic of Built-in Libraries
Python's standard library has many treasures waiting for you to discover. For example, the collections
module provides some efficient data structures:
from collections import deque, Counter
queue = deque(['a', 'b', 'c'])
queue.appendleft('d')
queue.pop()
counter = Counter(['a', 'b', 'c', 'a', 'b', 'b'])
print(counter.most_common(2)) # Outputs the two most common elements
Another powerful module is itertools
, which can help you create efficient iterators, greatly reducing memory usage:
from itertools import cycle
colors = cycle(['red', 'green', 'blue'])
for _ in range(10):
print(next(colors)) # Cycles through red, green, blue
These built-in libraries not only improve code efficiency but also make your code more concise and elegant. Why not take advantage?
Profiling is Key
Want to know where your code is slow? You need profiling tools. Python's built-in cProfile
module can help you analyze function call times and frequencies:
import cProfile
def slow_function():
for i in range(1000000):
i * i
cProfile.run('slow_function()')
Running this code will show you detailed information about each function call, including call counts and execution times. This way, you can identify performance bottlenecks and optimize accordingly.
For memory usage analysis, memory_profiler
is a good choice:
from memory_profiler import profile
@profile
def my_func():
a = [1] * (10 ** 6)
b = [2] * (2 * 10 ** 7)
del b
return a
if __name__ == '__main__':
my_func()
This tool can help you track memory usage for each line of code, making memory leaks easy to spot.
Concurrency is Powerful
For I/O-intensive tasks, multithreading can greatly improve efficiency. For CPU-intensive tasks, due to the GIL, multiprocessing is often a better choice.
Here's an example of multiprocessing:
from multiprocessing import Pool
def f(x):
return x*x
if __name__ == '__main__':
with Pool(5) as p:
print(p.map(f, [1, 2, 3]))
This code creates a pool of 5 processes that can compute square values in parallel. This method can significantly speed up processing for large amounts of data.
Generators Save Memory
When handling large amounts of data, generators can save a lot of memory. Instead of generating all data at once, generators produce data on demand:
squares = [x**2 for x in range(1000000)] # Generates all data immediately
squares_gen = (x**2 for x in range(1000000)) # Generates data on demand
for square in squares_gen:
print(square)
if square > 100:
break
Using generators, you can handle datasets far exceeding memory capacity, which is very useful in big data processing.
Loop Optimization is Important
Loops are one of the most common performance bottlenecks in programs. Optimizing loops can greatly improve program efficiency. A common technique is to reduce calculations within loops:
for i in range(1000000):
result = i * 2
factor = 2
for i in range(1000000):
result = i * factor
It seems like a small change, but for many iterations, this optimization can save a lot of time.
Another powerful technique is using NumPy for vectorized operations:
import numpy as np
result = []
for i in range(1000000):
result.append(i * 2)
array = np.arange(1000000)
result = array * 2
NumPy's vectorized operations not only make the code more concise but also execute much faster.
Caching is Powerful
For functions that are computationally complex but frequently called, using caching can greatly improve efficiency. Python’s functools
module provides a convenient decorator lru_cache
:
from functools import lru_cache
@lru_cache(maxsize=None)
def fib(n):
if n < 2:
return n
return fib(n-1) + fib(n-2)
print(fib(100)) # The first call will be slow
print(fib(100)) # The second call is almost instantaneous
In this example, the results of the fib
function are cached. When called again with the same parameters, the function returns the cached result instead of recalculating.
Avoid Unnecessary Calculations
In Python, we can use short-circuit conditions to avoid unnecessary calculations:
def complex_condition(x, y):
return x != 0 and y / x > 2
In this example, if x
equals 0, y / x
will not be calculated, avoiding a division by zero error. This technique not only improves efficiency but also makes your code more robust.
Conclusion
Well, dear Python developers, today we've learned many Python performance optimization techniques. From choosing the right data structures to using built-in libraries, profiling, concurrency, generators, loop optimization, caching, and avoiding unnecessary calculations, each technique can be the key to improving your code's performance.
Remember, performance optimization is an ongoing process. In practical development, we need to choose the right optimization strategies based on specific situations. Sometimes, code readability and maintainability may be more important than performance. So, find a balance between performance and other factors.
Do you have any experiences with Python performance optimization? Feel free to share your thoughts in the comments! Let’s discuss and improve together!
Happy coding, see you next time!
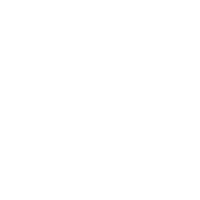
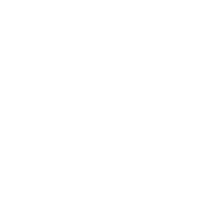
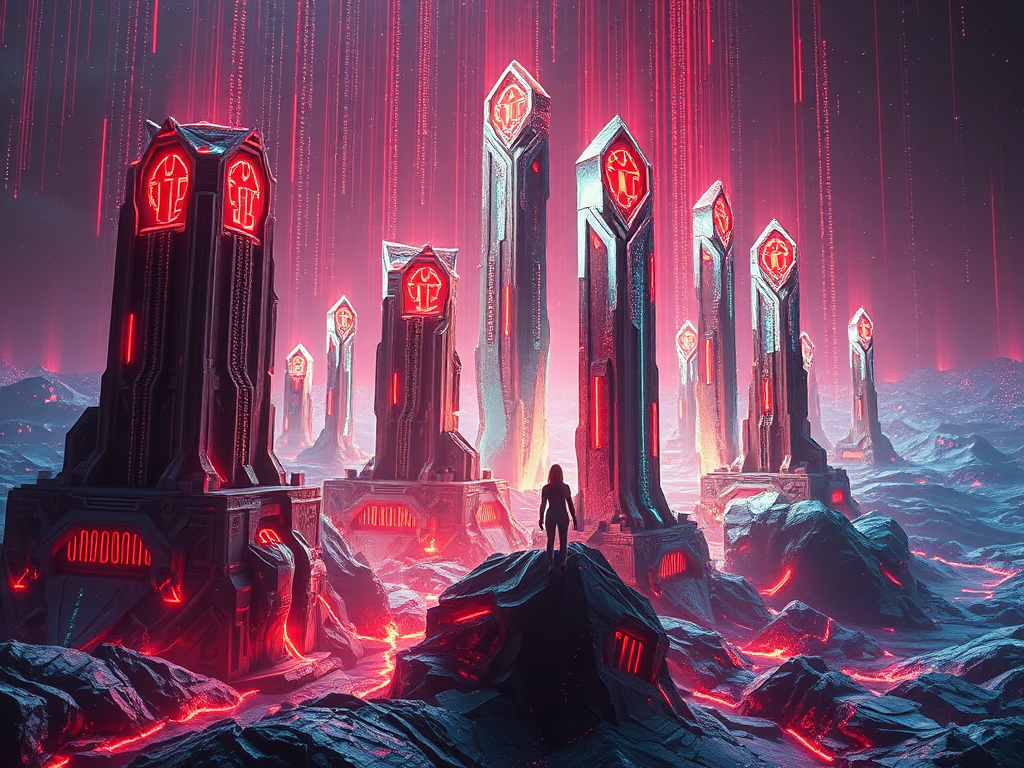
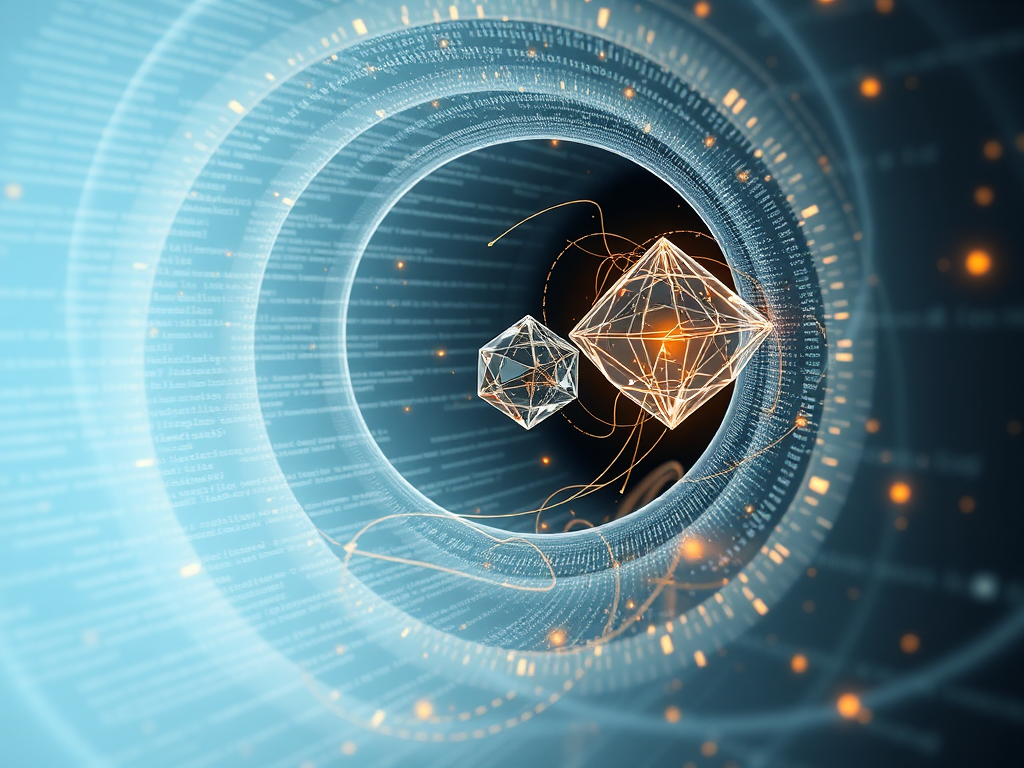
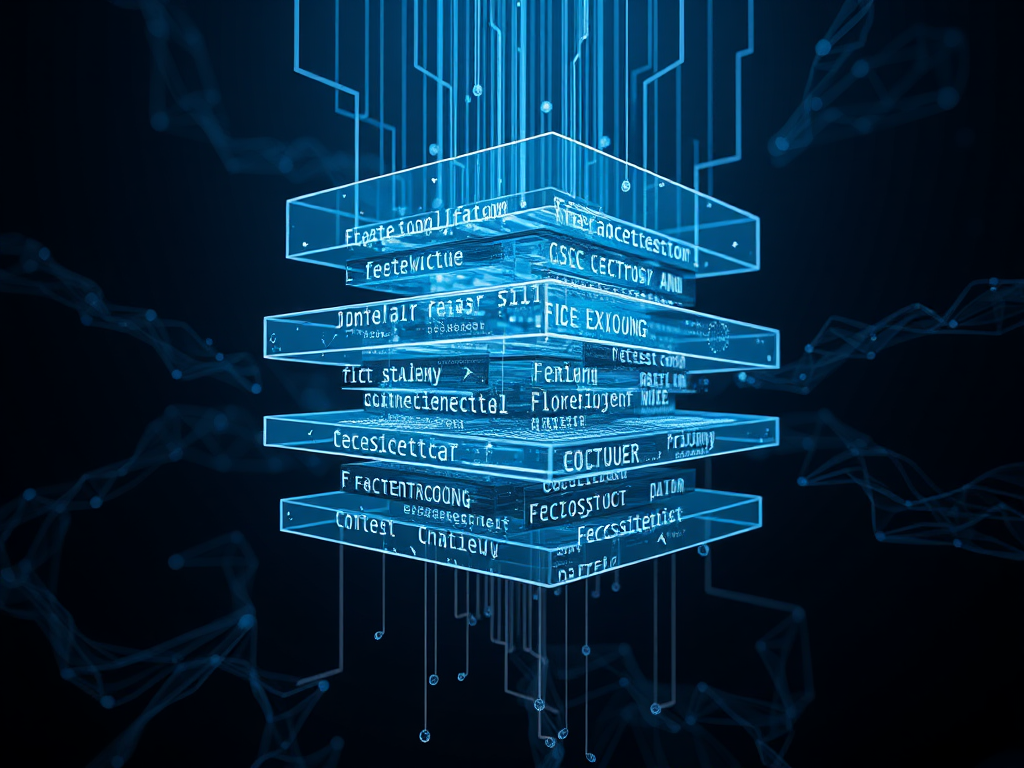